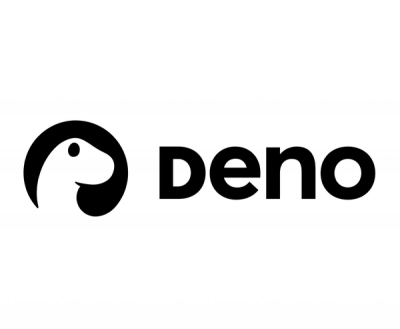
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
middlewarify
Advanced tools
Apply the middleware pattern to any function.
npm install middlewarify --save
Apply the middleware pattern:
var midd = require('middlewarify');
var tasks = module.exports = {};
// this will be the last callback to be invoked
tasks._create = function() {
console.log('tasks._create');
};
// Make the 'create' prop a middleware function.
midd.make(tasks, 'create', tasks._create);
...Add middleware
// ... somewhere far far away in another file
var tasks = require('./tasks');
// add a middleware to the 'create' operation
tasks.create.use(function(done){
console.log('middleware 1');
done();
});
// add another middleware to the 'create' operation
tasks.create.use(function(done){
console.log('middleware 2');
done();
});
... Invoke all the middleware
// ... Invoking them all together
tasks.create(function(err){
// all middleware finished.
});
The middlewarify.make()
method will apply the middleware pattern to an Object's property.
var crud = {};
middlewarify.make(crud, 'create');
middlewarify.make(crud, 'read');
middlewarify.make(crud, 'update');
middlewarify.make(crud, 'delete');
Each time make()
is used two new functions are added to the crud
Object:
crud.create([, ...], optCallback)
This method will invoke all added middleware in the sequence they were defined. If the final argument is a Function, it will be treated as a callback after all middleware have finished. crud.create( function( err ){ /* check err */ } )
crud.create.use(middleware [, ...])
This method will add middleware Functions to the crud.create
container.The middleware container exposes a use
method to add middleware. use()
accepts any number of parameters as long they are type Function or Arrays of Functions.
var crud = {};
middlewarify.make(crud, 'create');
// add middleware
crud.create.use([fn1, fn2], fn3);
The middleware container is a function that accepts any number of arguments and an optional callback.
Any argument passed to the middleware container will also be passed to all middleware.
var crud = {};
middlewarify.make(crud, 'create');
// run all middleware
crud.create(userDataObject);
The optional callback should always be defined last, it gets invoked when all middleware have finished. It provides one argument, the err
which if has a truthy value (typically an instance of Error
) meas that something did not go well.
var crud = {};
middlewarify.make(crud, 'create');
// run all middleware
crud.create(userDataObject, function(err) {
if (err) { /* tough love */ }
});
When adding a middleware
Function (i.e. crud.create.use(middleware)
) by default it will be invoked with only one argument, the next
callback. If you add arguments when calling the middleware container crud.create(arg1, arg2)
, all middleware callbacks will be invoked with these arguments first. The next
callback will always be last.
Invoking next() with a truthy argument (i.e.
next(err)
) will stop invoking more middleware. The flow is passed to theoptFinalCallback
if defined in themake()
method or silently dismissed.
crud.create.use(function(arg1, arg2, next) {
if (arg1 !== arg2) { return next(new Error('not a match'))}
next();
});
crud.create(foo, bar);
Copyright 2013 Thanasis Polychronakis
Licensed under the MIT License
FAQs
Apply the middleware pattern to any function.
The npm package middlewarify receives a total of 39 weekly downloads. As such, middlewarify popularity was classified as not popular.
We found that middlewarify demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.