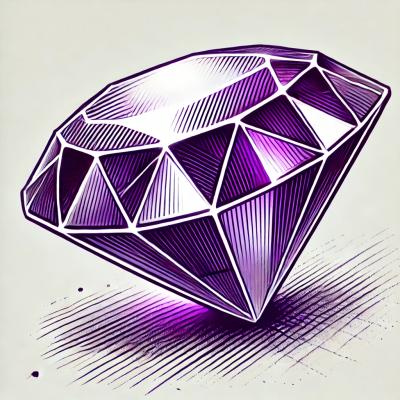
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
min-heap-typed
Advanced tools
This is a standalone Min Heap data structure from the data-structure-typed collection. If you wish to access more data structures or advanced features, you can transition to directly installing the complete data-structure-typed package
npm i min-heap-typed --save
yarn add min-heap-typed
import {MinHeap} from 'data-structure-typed';
// /* or if you prefer */ import {MinHeap} from 'heap-typed';
const minNumHeap = new MinHeap<number>();
minNumHeap.add(1).add(6).add(2).add(0).add(5).add(9);
minNumHeap.has(1) // true
minNumHeap.has(2) // true
minNumHeap.poll() // 0
minNumHeap.poll() // 1
minNumHeap.peek() // 2
minNumHeap.has(1); // false
minNumHeap.has(2); // true
const arrFromHeap = minNumHeap.toArray();
arrFromHeap.length // 4
arrFromHeap[0] // 2
arrFromHeap[1] // 5
arrFromHeap[2] // 9
arrFromHeap[3] // 6
minNumHeap.sort() // [2, 5, 6, 9]
const {MinHeap} = require('data-structure-typed');
// /* or if you prefer */ const {MinHeap} = require('heap-typed');
const minNumHeap = new MinHeap();
minNumHeap.add(1).add(6).add(2).add(0).add(5).add(9);
minNumHeap.has(1) // true
minNumHeap.has(2) // true
minNumHeap.poll() // 0
minNumHeap.poll() // 1
minNumHeap.peek() // 2
minNumHeap.has(1); // false
minNumHeap.has(2); // true
const arrFromHeap = minNumHeap.toArray();
arrFromHeap.length // 4
arrFromHeap[0] // 2
arrFromHeap[1] // 5
arrFromHeap[2] // 9
arrFromHeap[3] // 6
minNumHeap.sort() // [2, 5, 6, 9]
Data Structure | Unit Test | Performance Test | API Documentation | Implemented |
---|---|---|---|---|
Binary Tree | Binary Tree | |||
Binary Search Tree (BST) | BST | |||
AVL Tree | AVLTree | |||
Tree Multiset | TreeMultiset | |||
Segment Tree | SegmentTree | |||
Binary Indexed Tree | BinaryIndexedTree | |||
Graph | AbstractGraph | |||
Directed Graph | DirectedGraph | |||
Undirected Graph | UndirectedGraph | |||
Linked List | SinglyLinkedList | |||
Singly Linked List | SinglyLinkedList | |||
Doubly Linked List | DoublyLinkedList | |||
Queue | Queue | |||
Object Deque | ObjectDeque | |||
Array Deque | ArrayDeque | |||
Stack | Stack | |||
Coordinate Set | CoordinateSet | |||
Coordinate Map | CoordinateMap | |||
Heap | Heap | |||
Priority Queue | PriorityQueue | |||
Max Priority Queue | MaxPriorityQueue | |||
Min Priority Queue | MinPriorityQueue | |||
Trie | Trie |
Big O Notation | Type | Computations for 10 elements | Computations for 100 elements | Computations for 1000 elements |
---|---|---|---|---|
O(1) | Constant | 1 | 1 | 1 |
O(log N) | Logarithmic | 3 | 6 | 9 |
O(N) | Linear | 10 | 100 | 1000 |
O(N log N) | n log(n) | 30 | 600 | 9000 |
O(N^2) | Quadratic | 100 | 10000 | 1000000 |
O(2^N) | Exponential | 1024 | 1.26e+29 | 1.07e+301 |
O(N!) | Factorial | 3628800 | 9.3e+157 | 4.02e+2567 |
Data Structure | Access | Search | Insertion | Deletion | Comments |
---|---|---|---|---|---|
Array | 1 | n | n | n | |
Stack | n | n | 1 | 1 | |
Queue | n | n | 1 | 1 | |
Linked List | n | n | 1 | n | |
Hash Table | - | n | n | n | In case of perfect hash function costs would be O(1) |
Binary Search Tree | n | n | n | n | In case of balanced tree costs would be O(log(n)) |
B-Tree | log(n) | log(n) | log(n) | log(n) | |
Red-Black Tree | log(n) | log(n) | log(n) | log(n) | |
AVL Tree | log(n) | log(n) | log(n) | log(n) | |
Bloom Filter | - | 1 | 1 | - | False positives are possible while searching |
Name | Best | Average | Worst | Memory | Stable | Comments |
---|---|---|---|---|---|---|
Bubble sort | n | n2 | n2 | 1 | Yes | |
Insertion sort | n | n2 | n2 | 1 | Yes | |
Selection sort | n2 | n2 | n2 | 1 | No | |
Heap sort | n log(n) | n log(n) | n log(n) | 1 | No | |
Merge sort | n log(n) | n log(n) | n log(n) | n | Yes | |
Quick sort | n log(n) | n log(n) | n2 | log(n) | No | Quicksort is usually done in-place with O(log(n)) stack space |
Shell sort | n log(n) | depends on gap sequence | n (log(n))2 | 1 | No | |
Counting sort | n + r | n + r | n + r | n + r | Yes | r - biggest number in array |
Radix sort | n * k | n * k | n * k | n + k | Yes | k - length of longest key |
FAQs
Min Heap. Javascript & Typescript Data Structure.
The npm package min-heap-typed receives a total of 240 weekly downloads. As such, min-heap-typed popularity was classified as not popular.
We found that min-heap-typed demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.