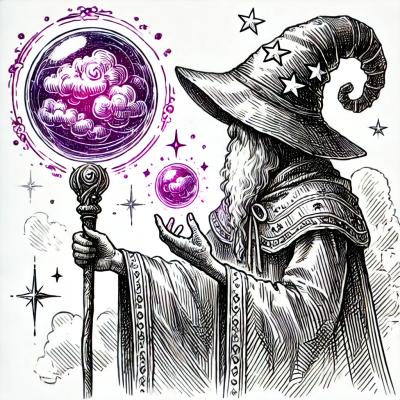
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
miniget is a lightweight HTTP request library for Node.js, primarily used for making simple GET requests. It is designed to be minimalistic and easy to use, focusing on simplicity and performance.
Basic GET Request
This feature allows you to make a basic GET request to a specified URL and handle the response as text. The code sample demonstrates how to fetch a JSON object from a placeholder API and log it to the console.
const miniget = require('miniget');
miniget('https://jsonplaceholder.typicode.com/todos/1').text().then(console.log).catch(console.error);
Stream Response
This feature allows you to stream the response directly to a writable stream, such as a file. The code sample demonstrates how to fetch a JSON object from a placeholder API and save it to a file named 'response.json'.
const miniget = require('miniget');
const fs = require('fs');
miniget('https://jsonplaceholder.typicode.com/todos/1').pipe(fs.createWriteStream('response.json'));
Custom Headers
This feature allows you to specify custom headers for your GET request. The code sample demonstrates how to set a custom 'User-Agent' header when making a request to a placeholder API.
const miniget = require('miniget');
const options = {
headers: {
'User-Agent': 'my-custom-agent'
}
};
miniget('https://jsonplaceholder.typicode.com/todos/1', options).text().then(console.log).catch(console.error);
Axios is a promise-based HTTP client for the browser and Node.js. It provides a more comprehensive set of features compared to miniget, including support for POST requests, request and response interceptors, and automatic transformation of JSON data. It is more feature-rich but also larger in size.
node-fetch is a lightweight module that brings `window.fetch` to Node.js. It is similar to miniget in terms of simplicity and ease of use but offers a more familiar API for those who have used the Fetch API in the browser. It supports a wider range of HTTP methods and is more versatile.
Got is a human-friendly and powerful HTTP request library for Node.js. It offers a rich set of features, including retries, streams, and advanced configuration options. Compared to miniget, Got is more robust and suitable for more complex use cases, but it is also larger and more complex.
A small http(s) GET library with redirects, retries, reconnects, concatenating or streaming, and no dependencies. This keeps filesize small for potential browser use.
Concatenates a response
const miniget = require('miniget');
miniget('http://mywebsite.com', (err, res, body) => {
console.log('webpage contents: ', body);
});
// with await
let [res, body] = await miniget.promise('http://yourwebsite.com');
Request can be streamed right away
miniget('http://api.mywebsite.com/v1/messages.json')
.pipe(someWritableStream());
Makes a GET request. options
can have any properties from the http.request()
function, in addition to
maxRedirects
- Default is 2
.maxRetries
- Number of times to retry the request if there is a 500 or connection error. Default is 1
.maxReconnects
- During a big download, if there is a disconnect, miniget can try to reconnect and continue the download where it left off. Defaults to 0
.backoff
- An object with inc
and max
used to calculate how long to wait to retry a request. Defaults to { inc: 100, max: 10000 }
.retryOnAuthError
- In addition to retrying the request on server and connection errors, any authentication errors will trigger a retry.highWaterMark
- Amount of data to buffer when in stream mode.transform
- Use this to add additional features. Called with the object that http.get()
or https.get()
would be called with. Must return a transformed object.acceptEncoding
- An object with encoding name as the key, and the value as a function that returns a decoding stream.
acceptEncoding: { gzip: () => require('zlip').createGunzip(stream) }
Given encodings will be added to the Accept-Encoding
header, and the response will be decoded if the server responds with encoded content.If callback
is given, will concatenate the response, and call callback
with a possible error, the response, and the response body. If you'd like a concatenated response, but want to use await
instead, you can call miniget.promise()
.
let [res, body] = await miniget.promise('http://yourwebsite.com');
Miniget returns a readable stream if callback
is not given, errors will then be emitted on the stream. Returned stream also contains an .abort()
method, and can emit the following events.
string
- URL redirected to.Emitted when the request was redirected with a redirection status code.
number
- Number of retry.Error
- Request or status code error.Emitted when the request fails, or the response has a status code >= 500.
number
- Number of reconnect.Error
- Request or response error.Emitted when the request or response fails after download has started.
npm install miniget
Tests are written with mocha
npm test
FAQs
A small HTTP(S) GET request library, with redirects and streaming.
We found that miniget demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.