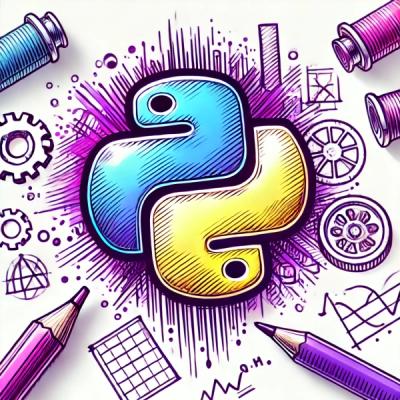
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
The MinIO npm package is a client library for interacting with MinIO and Amazon S3 compatible cloud storage services. It allows developers to perform a variety of operations such as uploading, downloading, and managing data in object storage.
Initialize MinIO Client
This code initializes a MinIO client instance with the necessary configuration such as endpoint, port, SSL usage, access key, and secret key.
const Minio = require('minio');
const minioClient = new Minio.Client({
endPoint: 'play.min.io',
port: 9000,
useSSL: true,
accessKey: 'Q3AM3UQ867SPQQA43P2F',
secretKey: 'zuf+tfteSlswRu7BJ86wekitnifILbZam1KYY3TG'
});
Upload an Object
This code uploads a file from the local filesystem to a specified bucket in the MinIO server.
minioClient.fPutObject('my-bucket', 'my-object', '/path/to/file', function(err, etag) {
if (err) return console.log(err);
console.log('File uploaded successfully.');
});
Download an Object
This code downloads an object from a specified bucket in the MinIO server to the local filesystem.
minioClient.fGetObject('my-bucket', 'my-object', '/path/to/destination', function(err) {
if (err) return console.log(err);
console.log('File downloaded successfully.');
});
List Objects in a Bucket
This code lists all objects in a specified bucket. It uses a stream to handle the data and errors.
var stream = minioClient.listObjects('my-bucket', '', true);
stream.on('data', function(obj) { console.log(obj); });
stream.on('error', function(err) { console.log(err); });
Remove an Object
This code removes a specified object from a bucket in the MinIO server.
minioClient.removeObject('my-bucket', 'my-object', function(err) {
if (err) return console.log(err);
console.log('Object removed successfully.');
});
The AWS SDK for JavaScript provides a comprehensive set of tools for interacting with Amazon Web Services, including S3. It offers similar functionalities to MinIO for managing object storage, but it is specifically designed for AWS services and has a broader scope covering many other AWS services.
The Google Cloud Storage client library for Node.js allows developers to interact with Google Cloud Storage. It provides similar functionalities to MinIO for managing object storage but is tailored for Google Cloud Platform services.
The Azure Storage SDK for Node.js provides tools for interacting with Azure Blob Storage. It offers similar functionalities to MinIO for managing object storage but is specifically designed for Microsoft Azure services.
The MinIO JavaScript Client SDK provides simple APIs to access any Amazon S3 compatible object storage server.
This quickstart guide will show you how to install the client SDK and execute an example JavaScript program. For a complete list of APIs and examples, please take a look at the JavaScript Client API Reference documentation.
This document assumes that you have a working nodejs setup in place.
npm install --save minio
git clone https://github.com/minio/minio-js
cd minio-js
npm install
npm install -g
npm install --save-dev @types/minio
You need five items in order to connect to MinIO object storage server.
Params | Description |
---|---|
endPoint | URL to object storage service. |
port | TCP/IP port number. This input is optional. Default value set to 80 for HTTP and 443 for HTTPs. |
accessKey | Access key is like user ID that uniquely identifies your account. |
secretKey | Secret key is the password to your account. |
useSSL | Set this value to 'true' to enable secure (HTTPS) access |
var Minio = require('minio')
var minioClient = new Minio.Client({
endPoint: 'play.min.io',
port: 9000,
useSSL: true,
accessKey: 'Q3AM3UQ867SPQQA43P2F',
secretKey: 'zuf+tfteSlswRu7BJ86wekitnifILbZam1KYY3TG'
});
This example program connects to an object storage server, makes a bucket on the server and then uploads a file to the bucket.
We will use the MinIO server running at https://play.min.io in this example. Feel free to use this service for testing and development. Access credentials shown in this example are open to the public.
var Minio = require('minio')
// Instantiate the minio client with the endpoint
// and access keys as shown below.
var minioClient = new Minio.Client({
endPoint: 'play.min.io',
port: 9000,
useSSL: true,
accessKey: 'Q3AM3UQ867SPQQA43P2F',
secretKey: 'zuf+tfteSlswRu7BJ86wekitnifILbZam1KYY3TG'
});
// File that needs to be uploaded.
var file = '/tmp/photos-europe.tar'
// Make a bucket called europetrip.
minioClient.makeBucket('europetrip', 'us-east-1', function(err) {
if (err) return console.log(err)
console.log('Bucket created successfully in "us-east-1".')
var metaData = {
'Content-Type': 'application/octet-stream',
'X-Amz-Meta-Testing': 1234,
'example': 5678
}
// Using fPutObject API upload your file to the bucket europetrip.
minioClient.fPutObject('europetrip', 'photos-europe.tar', file, metaData, function(err, etag) {
if (err) return console.log(err)
console.log('File uploaded successfully.')
});
});
node file-uploader.js
Bucket created successfully in "us-east-1".
mc ls play/europetrip/
[2016-05-25 23:49:50 PDT] 17MiB photos-europe.tar
The full API Reference is available here.
makeBucket
listBuckets
bucketExists
removeBucket
listObjects
listObjectsV2
listObjectsV2WithMetadata
(Extension)listIncompleteUploads
getBucketVersioning
setBucketVersioning
setBucketLifecycle
getBucketLifecycle
removeBucketLifecycle
getObjectLockConfig
setObjectLockConfig
getObject
putObject
copyObject
statObject
removeObject
removeObjects
removeIncompleteUpload
selectObjectContent
getBucketNotification
setBucketNotification
removeAllBucketNotification
listenBucketNotification
(MinIO Extension)FAQs
S3 Compatible Cloud Storage client
The npm package minio receives a total of 84,118 weekly downloads. As such, minio popularity was classified as popular.
We found that minio demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.