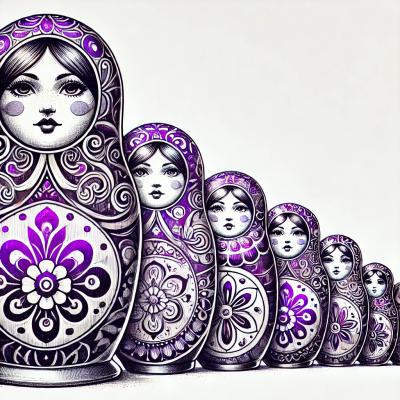
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
minisearch
Advanced tools
MiniSearch is a lightweight, full-text search engine for JavaScript. It is designed to be simple to use and efficient, making it suitable for client-side applications as well as server-side usage. MiniSearch allows you to index documents and perform search queries on them, providing features like tokenization, stemming, and field-based search.
Indexing Documents
This feature allows you to index a collection of documents. You specify which fields to index and which fields to store in the search results. The `addAll` method is used to add multiple documents to the index.
const MiniSearch = require('minisearch')
let miniSearch = new MiniSearch({
fields: ['title', 'text'], // fields to index for full-text search
storeFields: ['title'] // fields to return with search results
})
let documents = [
{ id: 1, title: 'Moby Dick', text: 'Call me Ishmael. Some years ago...' },
{ id: 2, title: 'Pride and Prejudice', text: 'It is a truth universally acknowledged...' },
// more documents...
]
miniSearch.addAll(documents)
Performing Searches
Once documents are indexed, you can perform search queries on them. The `search` method returns a list of documents that match the query, sorted by relevance.
let results = miniSearch.search('Ishmael')
console.log(results)
Customizing Tokenization
MiniSearch allows you to customize the tokenization process. In this example, the `tokenize` function splits the text into tokens based on whitespace.
let miniSearch = new MiniSearch({
fields: ['title', 'text'],
tokenize: (string, _fieldName) => string.split(/\s+/)
})
Stemming and Stop Words
You can also customize how terms are processed and specify stop words. In this example, terms are converted to lowercase, and common stop words are excluded from the index.
let miniSearch = new MiniSearch({
fields: ['title', 'text'],
processTerm: (term) => term.toLowerCase(),
stopWords: new Set(['the', 'is', 'and'])
})
Lunr.js is a small, full-text search library for use in the browser and Node.js. It provides similar functionality to MiniSearch, such as indexing and searching documents. However, Lunr.js is more feature-rich and has a larger community, which might make it a better choice for more complex applications.
Elasticlunr.js is a lightweight full-text search engine developed based on Lunr.js. It offers similar features to MiniSearch but with additional support for more advanced search capabilities like field boosting and custom scoring. It is a good alternative if you need more control over the search ranking.
Search-index is a powerful, full-text search library for Node.js and the browser. It provides a more comprehensive set of features compared to MiniSearch, including support for faceted search, real-time indexing, and more complex query capabilities. It is suitable for applications that require more advanced search functionalities.
MiniSearch
is a tiny but powerful in-memory full-text search engine for
JavaScript. It is respectful of resources, so it can comfortably run both in
Node and in the browser, but it can do a lot.
MiniSearch
addresses use cases where full-text search features are needed
(e.g. prefix search, fuzzy search, boosting of fields), but the data to be
indexed can fit locally in the process memory. While you may not index the whole
Wikipedia with it, there are surprisingly many use cases that are served well by
MiniSearch
. By storing the index in local memory, MiniSearch
can work
offline, and can process queries quickly, without network latency.
A prominent use-case is search-as-you-type features in web and mobile applications, where keeping the index on the client-side enables fast and reactive UI, removing the need to make requests to a search server.
Memory-efficient index, able to store tens of thousands of documents and terms in memory, even in the browser.
Exact match, prefix match and fuzzy match, all with a single performant and multi-purpose index data structure.
Small and maintainable code base, well tested, with no external dependency.
Provide good building blocks that empower developers to build solutions to their specific problems, rather than try to offer a general-purpose tool to satisfy every use-case at the cost of complexity.
With npm
:
npm install --save minisearch
With yarn
:
yarn add minisearch
API documentation is available, but here are some quick examples:
// A collection of documents for our example
const documents = [
{ id: 1, title: 'Moby Dick', text: 'Call me Ishmael. Some years ago...' },
{ id: 2, title: 'Zen and the Art of Motorcycle Maintenance', text: 'I can see by my watch...' },
{ id: 3, title: 'Neuromancer', text: 'The sky above the port was...' },
{ id: 4, title: 'Zen and the Art of Archery', text: 'At first sight it must seem...' },
// ...and more
]
let miniSearch = new MiniSearch({ fields: ['title', 'text'] })
// Index all documents
miniSearch.addAll(documents)
// Search with default options
let results = miniSearch.search('zen art motorcycle')
// => [ { id: 2, score: 2.77258, match: { ... } }, { id: 4, score: 1.38629, { ... } } ]
// Search only specific fields
results = miniSearch.search('zen', { fields: ['title'] })
// Boost fields
results = miniSearch.search('zen', { boost: { title: 2 } })
// Prefix search
results = miniSearch.search('moto', {
termToQuery: term => ({ term, prefix: true })
})
// Fuzzy search (in this example, with a max edit distance of 0.2 * term length,
// rounded to nearest integer)
results = miniSearch.search('ismael', {
termToQuery: term => ({ term, fuzzy: 0.2 })
})
// Set default search options upon initialization
miniSearch = new MiniSearch({
fields: ['title', 'text'],
searchOptions: {
boost: { title: 2 },
termToQuery: term => ({ term, fuzzy: 0.2 })
}
})
miniSearch.addAll(documents)
// Will now by default perform fuzzy search and boost "title":
results = miniSearch.search('zen and motorcycles')
FAQs
Tiny but powerful full-text search engine for browser and Node
The npm package minisearch receives a total of 208,915 weekly downloads. As such, minisearch popularity was classified as popular.
We found that minisearch demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.