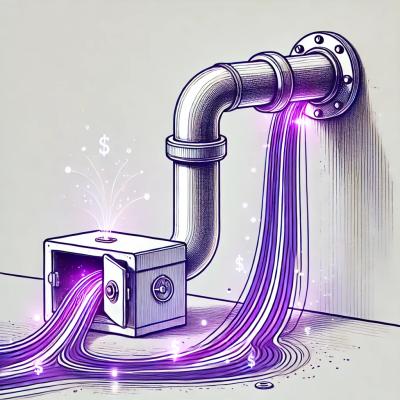
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
mirax-player
Advanced tools
Mirax Player is a powerful free video player for React, Vue, Angular, and Svelte that can embed videos from platforms like TikTok, YouTube/Shorts, and Vimeo. This library package enables you to set any URL once within a single embed code tag and dynamical
Disclaimer: This image is based on an example TikTok embedded video clip.
Mirax Player is a powerful free video player for React, Vue, Angular, and Svelte that can embed videos from platforms like TikTok, YouTube/Shorts, and Vimeo. This library package enables you to set any URL once within a single embed code tag and dynamically embed videos from any video sites. It was written in pure JavaScript but can be implemented in both TypeScript and JavaScript.
.whatever
class: margin: 0 auto;
and width: 100%;
Copy link
To install the Mirax Player, you can use the following npm command:
npm install mirax-player
Sites | Source type | Add-on Params | Links |
---|---|---|---|
YouTube / Shorts | Iframe Api | https://developers.google.com/youtube/player_parameters | https://developers.google.com/youtube/iframe_api_reference |
Vimeo | Player SDK | https://developer.vimeo.com/player/sdk/embed | https://developer.vimeo.com/player/sdk |
TikTok | oEmbed API | https://developers.tiktok.com/doc/embed-videos/ | https://developers.tiktok.com/doc/overview/ |
Thanks to these documentations of YouTube, Vimeo, and TikTok, I was able to integrate their APIs seamlessly into this library package. 😆 👏
Mirax embed tags | Type | Functionality |
---|---|---|
mirax-embed-class | class name | responsiveness |
data-mirax-width | attribute | dynamic width |
data-mirax-height | attribute | dynamic height |
data-mirax-embed | attribute | video links to embed |
example:
<div className="mirax-embed-class">
<div ref={embedVideo}
data-mirax-width="640" // You can set any value, such as 800x450, to make it larger.
data-mirax-height="360" //
data-mirax-embed="https://www.tiktok.com/@scout2015/video/6718335390845095173" // links from TikTok Youtube/Shorts and Vimeo
>
</div>
</div>
Keyboard keys / buttons | Functions | Description | Supported Browsers |
---|---|---|---|
space bar | Play & Pause | The video will play or pause | All browsers |
click ▶ | Play & Pause | The video will play or pause | All browsers |
alt+p | PiP | Picture in Picture screen | !firefox but auto appear PiP icon |
click Γ | PiP | Picture in Picture screen | All browsers |
double click the video | Fullscreen | It will set as fullscreen mode | All browsers |
click ❐ | Fullscreen | It will set as fullscreen mode | All browsers |
swipe for volume | Volume | To adjust the volume level | All browsers |
swipe for time frame | Progress bar | To adjust video frame timestamp | All browsers |
input:
src=" " // Link public/clip.mp4 from your frameworks | assets/clip.mp4 angular | example.com/video/clip.mp4
import React, { useEffect, useRef } from "react";
import { miraxplayer } from 'mirax-player';
const ExampleComponent = () => {
const videoPlayer = useRef(null);
const miraxCustomizer = {
playerTheme: "",
progressTheme: ""
};
useEffect(() => {
if (videoPlayer.current) {
miraxplayer(videoPlayer.current, miraxCustomizer);
}
});
return (
<div className="whatever">
<video ref={videoPlayer} className="mirax-player" src="clip.mp4"></video>
</div>
);
};
export default ExampleComponent;
import React, { useEffect, useRef } from "react";
import { miraxEmbed } from 'mirax-player';
const ExampleComponent = () => {
const embedVideo = useRef(null);
const youtubeParams = {
playerVars: {
controls: 1,
autoplay: 0,
fs: 1,
iv_load_policy: 3,
cc_load_policy: 1
}
};
const vimeoParams = {
autopause: 0,
controls: true,
responsive: true
};
useEffect(() => {
miraxEmbed(embedVideo.current, youtubeParams, vimeoParams);
});
return (
<div className="mirax-embed-class">
<div ref={embedVideo}
data-mirax-width="640"
data-mirax-height="360"
data-mirax-embed="https://vimeo.com/217499569">
</div>
</div>
);
};
export default ExampleComponent;
src/react/TypeScriptPlayer.md
src/react/TypeScriptEmbed.md
<template>
<div class="whatever">
<video ref="videoPlayer" class="mirax-player" src="clip.mp4"></video>
</div>
</template>
<script>
import { ref, onMounted } from "vue";
import { miraxplayer } from 'mirax-player';
export default {
setup() {
const videoPlayer = ref(null);
const miraxCustomizer = {
playerTheme: "",
progressTheme: ""
};
onMounted(() => {
if (videoPlayer.value) {
miraxplayer(videoPlayer.value, miraxCustomizer);
}
});
return {
videoPlayer
};
}
};
</script>
<template>
<div class="mirax-embed-class">
<div ref="embedVideo"
data-mirax-width="640"
data-mirax-height="360"
data-mirax-embed="https://vimeo.com/217499569">
</div>
</div>
</template>
<script>
import { ref, onMounted } from "vue";
import { miraxEmbed } from 'mirax-player';
export default {
setup() {
const embedVideo = ref(null);
const youtubeParams = {
playerVars: {
controls: 1,
autoplay: 0,
fs: 1,
iv_load_policy: 3,
cc_load_policy: 1
}
};
const vimeoParams = {
autopause: 0,
controls: true,
responsive: true
};
onMounted(() => {
if (embedVideo.value) {
miraxEmbed(embedVideo.value, youtubeParams, vimeoParams);
}
});
return {
embedVideo
};
}
};
</script>
src/vue/TypeScriptPlayer.md
src/vue/TypeScriptEmbed.md
import { Component, ElementRef, OnInit, ViewChild } from '@angular/core';
import { miraxplayer } from 'mirax-player';
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
styleUrls: ['./example.component.css']
})
export class ExampleComponent implements OnInit {
@ViewChild('videoPlayer', { static: true }) videoPlayer!: ElementRef<HTMLVideoElement>;
miraxCustomizer = {
playerTheme: "",
progressTheme: ""
};
ngOnInit(): void {
this.initializeMiraxplayer();
}
initializeMiraxplayer() {
if (this.videoPlayer.nativeElement) {
miraxplayer(this.videoPlayer.nativeElement, this.miraxCustomizer);
}
}
}
example.component.html
<div>
<div class="whatever">
<video #videoPlayer class="mirax-player" src="assets/clip.mp4"></video>
</div>
</div>
import { Component, ElementRef, OnInit, ViewChild } from '@angular/core';
import { miraxEmbed } from 'mirax-player';
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
styleUrls: ['./example.component.css']
})
export class ExampleComponent implements OnInit {
@ViewChild('embedVideo', { static: true }) embedVideo!: ElementRef;
constructor() { }
ngOnInit(): void {
const youtubeParams = {
playerVars: {
controls: 1,
autoplay: 0,
fs: 1,
iv_load_policy: 3,
cc_load_policy: 1
}
};
const vimeoParams = {
autopause: 0,
controls: true,
responsive: true
};
miraxEmbed(this.embedVideo.nativeElement, youtubeParams, vimeoParams);
}
}
example.component.html
<div class="mirax-embed-class">
<div #embedVideo
data-mirax-width="640"
data-mirax-height="360"
data-mirax-embed="https://vimeo.com/217499569">
</div>
</div>
<script>
import { onMount } from 'svelte';
import { miraxplayer } from 'mirax-player';
let videoPlayer;
const miraxCustomizer = {
playerTheme: "",
progressTheme: ""
};
onMount(() => {
if (videoPlayer) {
miraxplayer(videoPlayer, miraxCustomizer);
}
});
</script>
<div class="whatever">
<video bind:this={videoPlayer} class="mirax-player" src="clip.mp4">
<track kind="captions" src="" label="English" default>
</video>
</div>
<script>
import { onMount } from 'svelte';
import { miraxEmbed } from 'mirax-player';
let embedVideo;
const youtubeParams = {
playerVars: {
controls: 1,
autoplay: 0,
fs: 1,
iv_load_policy: 3,
cc_load_policy: 1
}
};
const vimeoParams = {
autopause: 0,
controls: true,
responsive: true
};
onMount(() => {
miraxEmbed(embedVideo, youtubeParams, vimeoParams);
});
</script>
<div class="mirax-embed-class">
<div bind:this={embedVideo} data-mirax-width="640" data-mirax-height="360" data-mirax-embed="https://vimeo.com/217499569"></div>
</div>
src/svelte/TypeScriptPlayer.md
src/svelte/TypeScriptEmbed.md
You can assign your own class name to encapsulate the video player.
.whatever {
position: relative;
float: left;
text-align: left;
}
.whatever {
position: relative;
text-align: center;
}
.whatever {
position: relative;
float: right;
text-align: right;
}
const miraxCustomizer = {
playerTheme: "rgba(250, 149, 35, 0.9)",
progressTheme: "rgba(17, 117, 59, 0.9)"
};
const miraxCustomizer = {
playerTheme: "rgb(0,0,0)",
progressTheme: "rgb(255, 255, 255)"
};
const miraxCustomizer = {
playerTheme: "#000000",
progressTheme: "#00ff00"
};
const miraxCustomizer = {
playerTheme: "black",
progressTheme: "red"
};
const playerTheme = "rgba(0, 0, 0, 0)";
You have the freedom to freely set a theme color.
Color Types | Color syntax | Example | Opacity Range | Appearance |
---|---|---|---|---|
RGBA | rgba() | rgba(255,0,0, 0.5) | 0.1 to 0.9 or 0 and 1 | Red half transparency |
RGB | rgb() | rgb(255, 0, 0) | none | Red |
HEXA | #6digits | #ff0000 | none | Red |
COLORNAME | colorname | red | none | Red |
Demjhon Silver
FAQs
A free video player compatible with React, Vue, Angular, and Svelte.
The npm package mirax-player receives a total of 4 weekly downloads. As such, mirax-player popularity was classified as not popular.
We found that mirax-player demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.