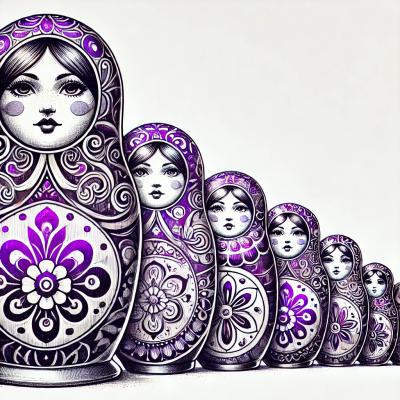
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Misuse resistant symmetric encryption using the AES-SIV (RFC 5297) and CHAIN/STREAM constructions
The best crypto you've never heard of, brought to you by Phil Rogaway
JavaScript-compatible TypeScript implementation of Miscreant: Advanced symmetric encryption using the AES-SIV (RFC 5297) and CHAIN/STREAM constructions, providing easy-to-use (or rather, hard-to-misuse) encryption of individual messages or message streams.
AES-SIV provides nonce-reuse misuse-resistance (NRMR): accidentally reusing a nonce with this construction is not a security catastrophe, unlike it is with more popular AES encryption modes like AES-GCM. With AES-SIV, the worst outcome of reusing a nonce is an attacker can see you've sent the same plaintext twice, as opposed to almost all other AES modes where it can facilitate chosen ciphertext attacks and/or full plaintext recovery.
For more information, see the toplevel README.md.
Have questions? Want to suggest a feature or change?
Though this library is written by cryptographic professionals, it has not undergone a thorough security audit, and cryptographic professionals are still humans that make mistakes. Use this library at your own risk.
This library contains two implementations of the cryptographic primitives which underlie its implementation: ones based on the Web Cryptography API, (a.k.a. Web Crypto) and a set of pure JavaScript polyfills.
By default only the Web Crypto versions will be used, and an exception raised if Web Crypto is not available. Users of this library may opt into using the polyfills in environments where Web Crypto is unavailable, but see the security notes below and understand the potential risks before doing so.
The Web Crypto API should provide access to high-quality implementations of the underlying cryptographic primitive functions used by this library in most modern browsers, implemented in optimized native code.
On Node.js, you will need a native WebCrypto provider such as node-webcrypto-ossl to utilize native code implementations of the underlying ciphers instead of the polyfills. However, please see the security warning on this package before using it.
The AES polyfill implementation (off by default, see above) uses table lookups and is therefore not constant time. This means there's potential that co-tenant or even remote attackers may be able to measure minute timing variations and use them to recover AES keys.
If at all possible, use the Web Crypto implementation instead of the polyfills.
Via npm:
npm install miscreant
Via Yarn:
yarn install miscreant
Import miscreant.js into your project with:
import Miscreant from "miscreant";
The Miscreant.importKey() method creates a new instance of an AES-SIV encryptor/decryptor.
Miscreant.importKey(keyData, algorithm[, crypto = window.crypto])
"AES-SIV"
.The Miscreant.importKey() method returns a Promise that, when fulfilled, returns a SIV encryptor/decryptor.
The Miscreant.importKey() method will throw an error if it's attempting to use
the default window.crypto
provider either doesn't exist (e.g. window
is
not defined because we're on Node.js) or if that provider does not provide
native implementations of the cryptographic primitives AES-SIV is built
on top of.
In these cases, you may choose to use PolyfillCrypto
, but be aware this may
decrease security.
// Assuming window.crypto.getRandomValues is available
let keyData = new Uint32Array(32);
window.crypto.getRandomValues(keyData);
let key = await Miscreant.importKey(keyData, "AES-SIV");
The seal() method encrypts a message along with a set of message headers known as associated data.
key.seal(associatedData, plaintext)
The seal() method returns a Promise that, when fulfilled, returns a Uint8Array containing the resulting ciphertext.
// Assuming window.crypto.getRandomValues is available
let keyData = new Uint8Array(32);
window.crypto.getRandomValues(keyData);
let key = await Miscreant.importKey(keyData, "AES-SIV");
// Encrypt plaintext
let plaintext = new Uint8Array([2,3,5,7,11,13,17,19,23,29]);
let nonce = new Uint8Array(16);
window.crypto.getRandomValues(nonce);
let ciphertext = await key.seal([nonce], plaintext);
The open() method decrypts a message which has been encrypted using AES-SIV.
key.open(associatedData, ciphertext)
The open() method returns a Promise that, when fulfilled, returns a Uint8Array containing the decrypted plaintext.
If the message has been tampered with or is otherwise corrupted, the promise will be rejected with an IntegrityError.
// Assuming window.crypto.getRandomValues is available
let keyData = new Uint8Array(32);
window.crypto.getRandomValues(keyData);
let key = await Miscreant.importKey(keyData, "AES-SIV");
// Encrypt plaintext
let plaintext = new Uint8Array([2,3,5,7,11,13,17,19,23,29]);
let nonce = new Uint8Array(16);
window.crypto.getRandomValues(nonce);
let ciphertext = await key.seal([nonce], plaintext);
// Decrypt ciphertext
var decrypted = await key.open([nonce], ciphertext);
WARNING: The polyfill implementation is not constant time! Please read the Polyfill Security Warning before proceeding!
By default, this library uses a WebCrypto-based implementation of AES-SIV and will throw an exception if WebCrypto is unavailable.
However, this library also contains a PolyfillCrypto
implementation which
can be passed as the second parameter to Miscreant.importKey()
. This implementation
uses pure JavaScript, however is not provided by default because there are
security concerns around its implementation.
This implementation should only be used in environments which have no support for WebCrypto whatsoever. WebCrypto should be available on most modern browsers. On Node.js, we would suggest you consider node-webcrypto-ossl before using the polyfill implementations, although please see that project's security warning before using it.
If you have already read the Polyfill Security Warning,
understand the security concerns, and would like to use it anyway, call the
following to obtain a PolyfillCrypto
instance:
Miscreant.getCryptoProvider("polyfill")
You can pass it to Miscreant.importKey()
like so:
const polyfillCrypto = Miscreant.getCryptoProvider("polyfill");
const key = Miscreant.importKey(keyData, "AES-SIV", polyfillCrypto);
Bug reports and pull requests are welcome on GitHub at https://github.com/miscreant/miscreant
Copyright (c) 2017 The Miscreant Developers.
AES polyfill implementation derived from the Go standard library: Copyright (c) 2012 The Go Authors. All rights reserved.
See LICENSE.txt for further details.
FAQs
Misuse resistant symmetric encryption library providing AES-SIV (RFC 5297), AES-PMAC-SIV, and STREAM constructions
The npm package miscreant receives a total of 11,654 weekly downloads. As such, miscreant popularity was classified as popular.
We found that miscreant demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.