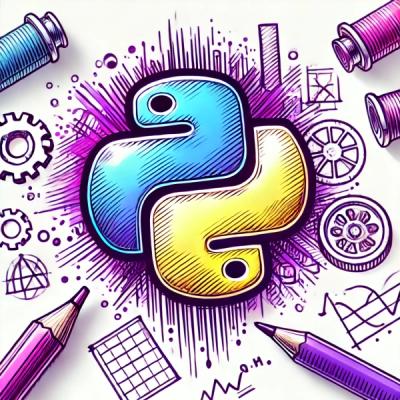
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
[](https://travis-ci.org/dmbch/mixinable) [](https://www.npmjs.com/package/mixinable)
mixinable
is a small utility library allowing you to use mixins in your code. Apart from enabling you to add and override new methods to your prototypes, it helps you apply different strategies to those additional methods.
mixinable
keeps your protoype chain intact (instanceof
works as expected), allows you to provide custom constructor
functions and supports asynchronous methods returning Promises
.
Using NPM:
npm install -S mixinable
Using Yarn:
yarn add mixinable
mixin([...definition])
The main export of mixinable
is a variadic function accepting any number of mixin definitions. It returns a constructor/factory function that creates instances containing the mixin methods you defined.
mixin.replace([...implementation])
replace
is the default mixin method application strategy. It mimics the behavior of, for example, Backbone's extend implementation. replace
accepts any number of functions, i.e. implementations.
mixin.parallel([...implementation])
parallel
executes all defined implementations in parallel. This is obviously most useful if there are asynchronous implementations involved - otherwise, it behaves identically to sequence
.
mixin.pipe([...implementation])
pipe
passes the each implementation's output to the next, using the first argument as the initial value. All other arguments are being passed to all implementations as-is.
mixin.sequence([...implementation])
sequence
executes all implementation sequentially, passing all arguments unchanged. Use it if your implementations might rely on others changing the instance they are run on.
import mixin from 'mixinable';
// const Foo = mixin({
const createFoo = mixin({
bar() {
// ...
}
});
// const foo = new Foo();
const foo = createFoo();
// console.log(foo instanceof Foo);
console.log(foo instanceof createFoo);
import mixin from 'mixinable';
// multiple mixin arguments are being merged
const createFoo = mixin(
{
bar() {
// ...
}
},
{
// you can pass multiple implementations at once
baz: [
function () { /* ... */ },
function () { /* ... */ }
]
}
);
// constructors/factories created with mixin can be extended
const createQux = createFoo.mixin({
bar() {
// ...
}
});
parallel
Exampleimport mixin, { parallel } from 'mixinable';
// const Foo = mixin({
const createFoo = mixin({
bar: parallel(
function () {
return Promise.resolve(1);
},
function () {
return Promise.resolve(2);
}
)
});
const foo = createFoo();
foo.bar().then(res => console.log(res));
// [1, 2]
pipe
Exampleimport mixin, { pipe } from 'mixinable';
// const Foo = mixin({
const createFoo = mixin({
bar: pipe(
function (val, inc) {
return Promise.resolve(val + inc);
},
function (val, inc) {
return (val + inc);
}
)
});
const foo = createFoo();
foo.bar(0, 1).then(res => console.log(res));
// 2
sequence
Exampleimport mixin, { sequence } from 'mixinable';
// const Foo = mixin({
const createFoo = mixin({
bar: sequence(
function (options) {
this.baz = options.baz;
},
function (options) {
this.qux = this.bar * 42;
}
)
});
const foo = createFoo();
foo.bar({ baz: 23 });
console.log(foo.qux);
// '966'
If you want to contribute to this project, create a fork of its repository using the GitHub UI. Check out your new fork to your computer:
mkdir mixinable && cd $_
git clone git@github.com:user/mixinable.git
Afterwards, you can yarn install
the required dev dependencies and start hacking away. When you are finished, please do go ahead and create a pull request.
mixinable
is entirely written in ECMAScript 5 and adheres to semistandard code style. Please make sure your contribution does, too.
FAQs
Functional JavaScript Mixin Utility
The npm package mixinable receives a total of 152 weekly downloads. As such, mixinable popularity was classified as not popular.
We found that mixinable demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.