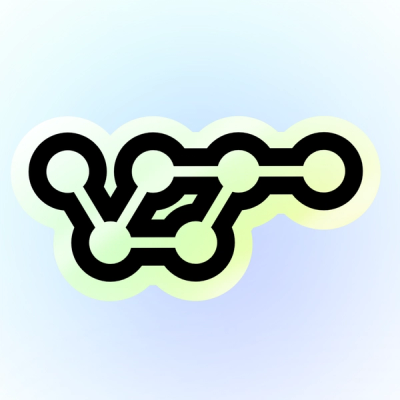
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
The mkdirp npm package is a utility module that allows you to create directories and all necessary subdirectories in a single command, similar to the 'mkdir -p' command in UNIX systems. It is useful for ensuring that a given directory path exists without manually checking and creating each level of the directory structure.
Create a directory with subdirectories
This feature allows you to create a directory and any necessary parent directories. The function returns a promise that resolves to the first directory that had to be created, or null if all directories already existed.
const mkdirp = require('mkdirp');
mkdirp('/tmp/some/long/path').then(made => console.log(`Made directories: ${made}`));
Use with async/await
This feature demonstrates how mkdirp can be used with async/await syntax for cleaner, more readable asynchronous code.
const mkdirp = require('mkdirp');
(async () => {
try {
const made = await mkdirp('/tmp/some/long/path');
console.log(`Made directories: ${made}`);
} catch (e) {
console.error(e);
}
})();
Custom file mode (permissions)
This feature allows you to specify the file mode (permissions) when creating directories. The mode can be set so that the created directories have the desired permissions.
const mkdirp = require('mkdirp');
mkdirp('/tmp/some/long/path', { mode: 0o775 }).then(made => console.log(`Made directories with mode: ${made}`));
fs-extra is a package that builds on the native fs module, providing additional methods and ensuring consistency across different platforms. It includes a method called 'ensureDir' which is similar to mkdirp's functionality, ensuring that a directory exists and creating it if necessary.
make-dir is another package that offers similar functionality to mkdirp. It provides a method to create a directory and its parents if needed. One of the differences is that make-dir uses the native fs.mkdir/mkdirSync with the recursive option when available (Node.js v10.12.0 or later), potentially providing better performance on newer Node.js versions.
Like mkdir -p
, but in Node.js!
Now with a modern API and no* bugs!
* may contain some bugs
// hybrid module, import or require() both work
import { mkdirp } from 'mkdirp'
// or:
const { mkdirp } = require('mkdirp')
// return value is a Promise resolving to the first directory created
mkdirp('/tmp/foo/bar/baz').then(made =>
console.log(`made directories, starting with ${made}`)
)
Output (where /tmp/foo
already exists)
made directories, starting with /tmp/foo/bar
Or, if you don't have time to wait around for promises:
import { mkdirp } from 'mkdirp'
// return value is the first directory created
const made = mkdirp.sync('/tmp/foo/bar/baz')
console.log(`made directories, starting with ${made}`)
And now /tmp/foo/bar/baz exists, huzzah!
import { mkdirp } from 'mkdirp'
mkdirp(dir: string, opts?: MkdirpOptions) => Promise<string | undefined>
Create a new directory and any necessary subdirectories at dir
with octal permission string opts.mode
. If opts
is a string
or number, it will be treated as the opts.mode
.
If opts.mode
isn't specified, it defaults to 0o777
.
Promise resolves to first directory made
that had to be
created, or undefined
if everything already exists. Promise
rejects if any errors are encountered. Note that, in the case of
promise rejection, some directories may have been created, as
recursive directory creation is not an atomic operation.
You can optionally pass in an alternate fs
implementation by
passing in opts.fs
. Your implementation should have
opts.fs.mkdir(path, opts, cb)
and opts.fs.stat(path, cb)
.
You can also override just one or the other of mkdir
and stat
by passing in opts.stat
or opts.mkdir
, or providing an fs
option that only overrides one of these.
mkdirp.sync(dir: string, opts: MkdirpOptions) => string|undefined
Synchronously create a new directory and any necessary
subdirectories at dir
with octal permission string opts.mode
.
If opts
is a string or number, it will be treated as the
opts.mode
.
If opts.mode
isn't specified, it defaults to 0o777
.
Returns the first directory that had to be created, or undefined if everything already exists.
You can optionally pass in an alternate fs
implementation by
passing in opts.fs
. Your implementation should have
opts.fs.mkdirSync(path, mode)
and opts.fs.statSync(path)
.
You can also override just one or the other of mkdirSync
and
statSync
by passing in opts.statSync
or opts.mkdirSync
, or
providing an fs
option that only overrides one of these.
mkdirp.manual
, mkdirp.manualSync
Use the manual implementation (not the native one). This is the default when the native implementation is not available or the stat/mkdir implementation is overridden.
mkdirp.native
, mkdirp.nativeSync
Use the native implementation (not the manual one). This is the default when the native implementation is available and stat/mkdir are not overridden.
On Node.js v10.12.0 and above, use the native fs.mkdir(p, {recursive:true})
option, unless fs.mkdir
/fs.mkdirSync
has
been overridden by an option.
made
.fs.mkdir(path, { recursive: true })
(or fs.mkdirSync
)made
.fs.mkdir
implementation, with recursive: false
made
mkdir
errormade
mkdir
errorundefined
if a root dir, or made
if set, or path
On Windows file systems, attempts to create a root directory (ie,
a drive letter or root UNC path) will fail. If the root
directory exists, then it will fail with EPERM
. If the root
directory does not exist, then it will fail with ENOENT
.
On posix file systems, attempts to create a root directory (in
recursive mode) will succeed silently, as it is treated like just
another directory that already exists. (In non-recursive mode,
of course, it fails with EEXIST
.)
In order to preserve this system-specific behavior (and because
it's not as if we can create the parent of a root directory
anyway), attempts to create a root directory are passed directly
to the fs
implementation, and any errors encountered are not
handled.
The native implementation (as of at least Node.js v13.4.0) does not provide appropriate errors in some cases (see nodejs/node#31481 and nodejs/node#28015).
In order to work around this issue, the native implementation
will fall back to the manual implementation if an ENOENT
error
is encountered.
There are a few to choose from! Use the one that suits your needs best :D
fs.mkdir(path, {recursive: true}, cb)
if:ENOENT
as the error when some other
problem is the actual cause.fs
methods in use.ENOENT
to achieve this.fs
methods in use.make-dir
if:stat
calls when using the native implementation for
this reason).ENOENT
errors for
failures that are actually related to something other than a
missing file system entry.fs
methods in use.fs
methods in use.Promise
language primitive. (Please don't.
You deserve better.)This package also ships with a mkdirp
command.
$ mkdirp -h
usage: mkdirp [DIR1,DIR2..] {OPTIONS}
Create each supplied directory including any necessary parent directories
that don't yet exist.
If the directory already exists, do nothing.
OPTIONS are:
-m<mode> If a directory needs to be created, set the mode as an octal
--mode=<mode> permission string.
-v --version Print the mkdirp version number
-h --help Print this helpful banner
-p --print Print the first directories created for each path provided
--manual Use manual implementation, even if native is available
With npm do:
npm install mkdirp
to get the library locally, or
npm install -g mkdirp
to get the command everywhere, or
npx mkdirp ...
to run the command without installing it globally.
This module works on node v8, but only v10 and above are officially supported, as Node v8 reached its LTS end of life 2020-01-01, which is in the past, as of this writing.
MIT
FAQs
Recursively mkdir, like `mkdir -p`
The npm package mkdirp receives a total of 47,083,734 weekly downloads. As such, mkdirp popularity was classified as popular.
We found that mkdirp demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.