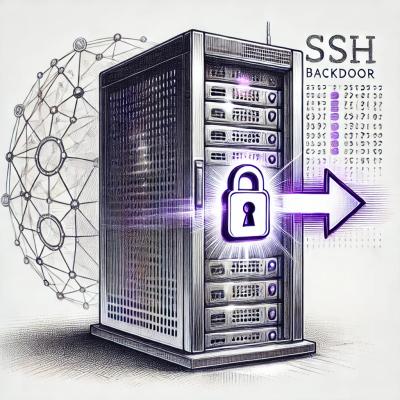
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Create connection
const mkrweb = new MKRWEB({
sql: {
database: 'mkrweb_db',
host: 'localhost',
username: 'root',
password: ''
}
})
Assign the path and language for recording log files
const sql = mkrweb.sql();
sql.setLanguage("EN");
sql.setPath(join('sql.log'));
Exmaple log file
-------ERROR IN ASYNCHRONOUS REQUEST-------
Code: ER_PARSE_ERROR
Message: You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'use' at line 1
Request: SELECT * FROM use
Time: 23.11.2023 22:22:49
Select data from database (Synchronous / Asynchronous)
const data = {
columns: '*',
identifier: "name = 'Alex'",
order: {
column: 'id',
type: "DESC",
limit: 10
}
}
//Asynchronous
sql.select<userType[]>('user', data, result => console.log(result));
//Synchronous
sql.selectSync<userType[]>('users', data).then(result => console.log(result));
Add data to database (Synchronous / Asynchronous)
// table columns: id, name, age, email, password
// If id is auto increment then write null
const data = [null, 'Alex', 18, 'alex@email.com', 'hwabcdksjb'];
//Asynchronous
sql.add('users', data, added => {
if(added) console.log('user added');
else console.log('user not added');
});
//Synchronous
sql.addSync('users', data).then(added => {
if(added) console.log('user added');
else console.log('user not added');
})
Update data in database (Synchronous / Asynchronous)
const columns = ['name', 'email'];
const newData = ['Jack', 'jack@email.com'];
//Asynchronous
sql.update('users', {columns: columns, values: newData, identifier: 'id = 5'}, updated => {
if(updated) console.log('user updated');
else console.log('user not updated');
})
//Synchronous
sql.updateSync('users', {columns: columns, values: newData, identifier: 'id = 5'}).then( updated => {
if(updated) console.log('user updated');
else console.log('user not updated');
})
Delete data from database (Synchronous / Asynchronous)
// if you do not specify an identifier, all rows will be deleted from the database
//Asynchronous
sql.delete({table: 'users', identifier: 'id = 5'}, deleted => {
if(deleted) console.log('user deleted');
else console.log('user not deleted');
});
//Synchronous
sql.deleteSync({table: 'users', identifier: 'id = 5'}).then(deleted => {
if(deleted) console.log('user deleted');
else console.log('user not deleted');
});
Get a crypter
const crypto = mkrweb.crypt();
Encrypt data You can encrypt both strings and objects, arrays, etc...
const string = 'hello world!';
const user = {
name: 'Alex',
age: 18
}
const encryptString = crypto.encrypt(string);
// bUpmUlcrUERTUDUlU1A1JTQ4NDVzcml4V3NFczQ4NDVBdjd6U1A1JU1FYVA5ZjRn
const encryptUser = crypto.encrypt(JSON.stringify(user));
// dmpkYXM2NWQ3c1c1UXBzVG1qSGRXK1BEczY1ZHh2d3NzNjVkTFMmV1NQNSVXK1BEYk5hc3M2NWQwNmdhczY1ZFFwc1RXYkhzVytQRHM2NWR4dndza29zcHNkZGt2c3B4
Decrypt data
const decryptString = crypto.decrypt(encryptString);
// hello world!
const decryptUser = crypto.decrypt(encryptUser);
// {"name":"Alex","age":18}
Remove HTML tags
const user = {
name: '<h1>Alex</h1>',
age: '<script>alert(18)</script>'
}
const removeHtml = crypto.clearHTML(user);
// { name: 'h1Alexh1', age: 'scriptalert(18)script' }
This method monitors the number of requests to your server and if the requests exceed the norm, it blocks the user’s ip
Create connection
const mkrweb = new MKRWEB({
bot: {
path: join(__dirname, 'bots.JSON'),
block: join(__dirname, 'block.JSON'),
interval: 3000, // Blocking interval
maxRequests: 50 // Maximum number of requests
}
})
How does it work
// ***Server simulation
const ip = '15.210.48.54';
for(let i = 0; i < 100; i++){
if(bot.check(ip)) {
console.log('user blocked!'); break;
}
}
FAQs
Small package for server-side development.
The npm package mkrweb receives a total of 0 weekly downloads. As such, mkrweb popularity was classified as not popular.
We found that mkrweb demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.