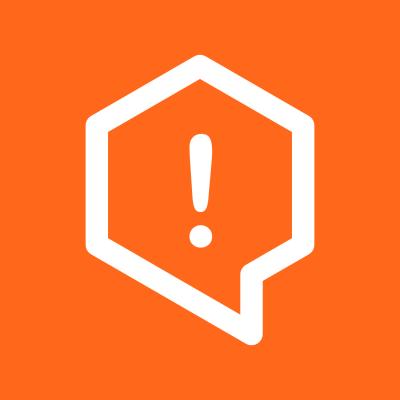
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
mobx-react-lite
Advanced tools
mobx-react-lite is a lightweight wrapper around MobX that provides React bindings for functional components. It allows you to use MobX for state management in your React applications with minimal boilerplate and high performance.
Observer Component
The `observer` function is used to create a reactive component that will automatically re-render when the observed state changes. In this example, the `Counter` component re-renders whenever `counter.count` is updated.
import React from 'react';
import { observer } from 'mobx-react-lite';
import { observable } from 'mobx';
const counter = observable({ count: 0 });
const Counter = observer(() => (
<div>
<button onClick={() => counter.count++}>Increment</button>
<p>{counter.count}</p>
</div>
));
export default Counter;
Using MobX stores
This example demonstrates how to use MobX stores with `mobx-react-lite`. The `CounterStore` class is an observable store, and the `Counter` component observes changes to the store and re-renders accordingly.
import React from 'react';
import { observer } from 'mobx-react-lite';
import { observable } from 'mobx';
class CounterStore {
@observable count = 0;
increment() {
this.count++;
}
}
const counterStore = new CounterStore();
const Counter = observer(() => (
<div>
<button onClick={() => counterStore.increment()}>Increment</button>
<p>{counterStore.count}</p>
</div>
));
export default Counter;
Using hooks with MobX
The `useLocalObservable` hook allows you to create local observable state within a functional component. This example shows how to use the hook to create a local counter state and update it within the component.
import React from 'react';
import { observer, useLocalObservable } from 'mobx-react-lite';
const Counter = observer(() => {
const counter = useLocalObservable(() => ({ count: 0, increment() { this.count++; } }));
return (
<div>
<button onClick={counter.increment}>Increment</button>
<p>{counter.count}</p>
</div>
);
});
export default Counter;
Redux is a popular state management library for JavaScript applications. Unlike MobX, which uses observables and reactive programming, Redux relies on a unidirectional data flow and immutable state updates. Redux is often used with React through the `react-redux` bindings.
Recoil is a state management library for React that provides a set of utilities for managing state in a more granular and efficient way. It uses atoms and selectors to manage state and derive computed values. Recoil is designed to work seamlessly with React's concurrent mode.
Zustand is a small, fast, and scalable state management library for React. It uses hooks to manage state and provides a simple API for creating and updating state. Zustand is less opinionated than MobX and can be a good choice for simpler state management needs.
This is a next iteration of mobx-react coming from introducing React hooks which simplifies a lot of internal workings of this package.
You need React version 16.7.0-alpha.0 which is highly experimental and not recommended for a production.
The more detailed documentation will be coming later. For now you can just use observer
& Observer
same way as before. There is no Provider/inject
anymore as these can be handled by React.createContext
without extra hassle. There might be bugs, as tests are not covering all scenarios just yet. No devtools & SSR support.
Note that class based components are not supported except using <Observer>
directly in its render
method.
FAQs
Lightweight React bindings for MobX based on React 16.8+ and Hooks
The npm package mobx-react-lite receives a total of 1,150,902 weekly downloads. As such, mobx-react-lite popularity was classified as popular.
We found that mobx-react-lite demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.