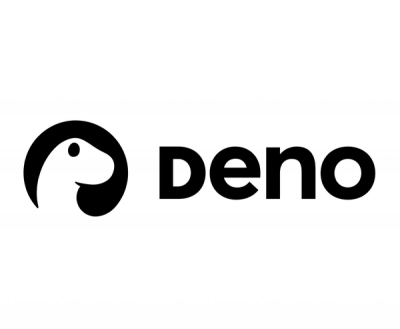
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
This is not a mocking library. This module allows you to require a module and pass mocks for its dependencies.
Dependencies that are not passed will be solved normally.
This module uses vm.runInNewContext and is heavily inspired by this answer in stackoverflow answer.
npm install mockuire
Given a file like this one foo.js
:
var path = require("path");
module.exports = function(a, b){
return path.join(a, b, "burbujas");
};
then we can test as follows:
var mockuire = require("mockuire")(module);
exports.test = function (test) {
//this is the "mock" of the module path
var mockedPath = {
join: function() {
return Array.prototype.slice.call(arguments, 0).join("!");
}
};
//now I "mockuire" the module under test with the mocked path
var foo = mockuire("./fixture/foo", { path: mockedPath });
//let's see if it works:
result = foo( "a", "b" );
test.equal( result, "a!b!burbujas" );
}
You have to pass the module in order to fallback to the module require when needed.
If your SUT is coffee script use this syntax:
var mockuire = require("mockuire")(module, { "coffee": require("coffee-script") });
where "coffee" is the extension and the next thing needs to have a compile function.
Two new methods will be added to the instance returned by mockuire.
Given a file like private.js
:
var count = 1;
module.exports.inc = function() {
return ++count;
};
###method: _private_get(name)### It allows you to get the value of a private variable:
it ('should be able to get value of a private evariable', function() {
var mockuire = require("mockuire")(module);
var private = mockuire("./fixture/private");
assert.equal(private._private_get('count'), 1);
});
###method: _private_set(name, value)### It allows you to set the value of a private variable:
it ('should be able to get value of a private evariable', function() {
var mockuire = require("mockuire")(module);
var private = mockuire("./fixture/private");
private._private_set('count', 10);
assert.equal(private.inc(), 11);
});
You can also set private members in the same moment you pass mocks for its dependencies
it('should be able to set value of a private evariable', function() {
var mockuire = require("../lib/index")(module);
var mocks = {};
var props = {
count: 100
};
var private = mockuire("./fixture/private", mocks, props);
assert.equal(private.inc(), 101);
});
npm test
FAQs
require a module with mocked dependencies
The npm package mockuire receives a total of 35 weekly downloads. As such, mockuire popularity was classified as not popular.
We found that mockuire demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.