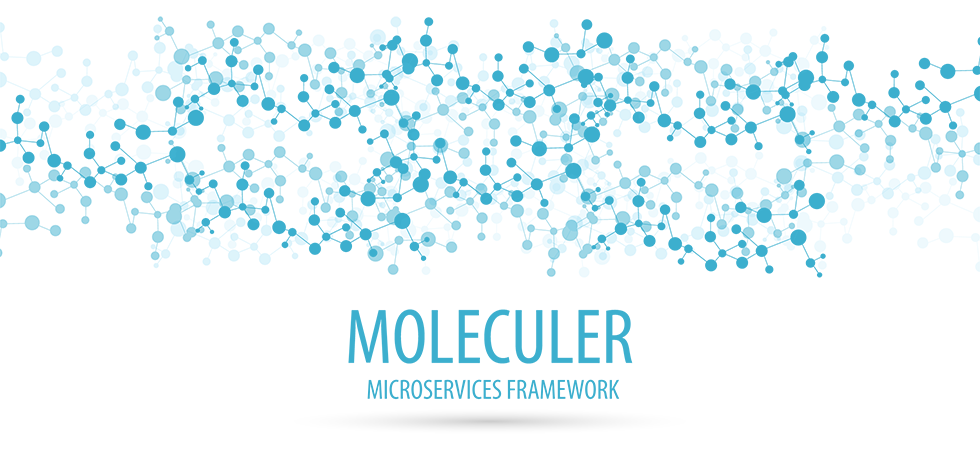
moleculer-db-adapter-sequelize 
SQL adapter (Postgres, MySQL, SQLite & MSSQL) for Moleculer DB service with Sequelize.
Features
Install
$ npm install moleculer-db-adapter-sequelize sequelize --save
You have to install additional packages for your database server:
$ npm install sqlite3 --save
$ npm install mysql2 --save
$ npm install mariadb --save
$ npm install pg pg-hstore --save
$ npm install tedious --save
Usage
"use strict";
const { ServiceBroker } = require("moleculer");
const DbService = require("moleculer-db");
const SqlAdapter = require("moleculer-db-adapter-sequelize");
const Sequelize = require("sequelize");
const broker = new ServiceBroker();
broker.createService({
name: "posts",
mixins: [DbService],
adapter: new SqlAdapter("sqlite://:memory:"),
model: {
name: "post",
define: {
title: Sequelize.STRING,
content: Sequelize.TEXT,
votes: Sequelize.INTEGER,
author: Sequelize.INTEGER,
status: Sequelize.BOOLEAN
},
options: {
}
},
});
broker.start()
.then(() => broker.call("posts.create", {
title: "My first post",
content: "Lorem ipsum...",
votes: 0
}))
.then(() => broker.call("posts.find").then(console.log));
Raw queries
You can reach the sequelize
instance via this.adapter.db
. To call Raw queries:
actions: {
findHello2() {
return this.adapter.db.query("SELECT * FROM posts WHERE title = 'Hello 2' LIMIT 1")
.then(([res, metadata]) => res);
}
}
Options
Every constructor arguments are passed to the Sequelize
constructor. Read more about Sequelize connection.
Example with connection URI
new SqlAdapter("postgres://user:pass@example.com:5432/dbname");
Example with connection options
new SqlAdapter('database', 'username', 'password', {
host: 'localhost',
dialect: ,
pool: {
max: 5,
min: 0,
idle: 10000
},
storage: 'path/to/database.sqlite'
});
Test
$ npm test
In development with watching
$ npm run ci
License
The project is available under the MIT license.
Contact
Copyright (c) 2016-2024 MoleculerJS
