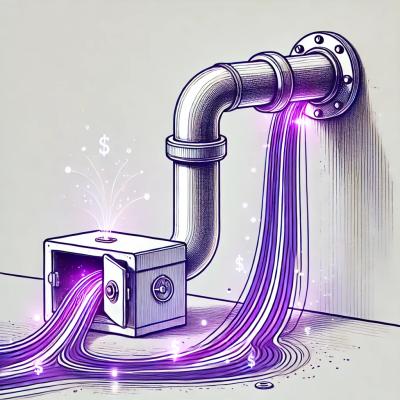
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
moment-range
Advanced tools
The moment-range npm package extends the functionality of the Moment.js library to handle date ranges. It allows you to create, manipulate, and query ranges of dates with ease.
Creating Date Ranges
This feature allows you to create a date range by specifying a start and end date. The code sample demonstrates how to create a date range from January 1, 2023, to December 31, 2023.
const moment = require('moment');
const { extendMoment } = require('moment-range');
const momentRange = extendMoment(moment);
const start = moment('2023-01-01');
const end = moment('2023-12-31');
const range = momentRange.range(start, end);
console.log(range.toString());
Checking if a Date is within a Range
This feature allows you to check if a specific date falls within a given date range. The code sample checks if June 15, 2023, is within the range from January 1, 2023, to December 31, 2023.
const moment = require('moment');
const { extendMoment } = require('moment-range');
const momentRange = extendMoment(moment);
const start = moment('2023-01-01');
const end = moment('2023-12-31');
const range = momentRange.range(start, end);
const date = moment('2023-06-15');
console.log(range.contains(date)); // true
Iterating over Date Ranges
This feature allows you to iterate over each day within a date range. The code sample iterates over each day from January 1, 2023, to January 7, 2023, and prints the date.
const moment = require('moment');
const { extendMoment } = require('moment-range');
const momentRange = extendMoment(moment);
const start = moment('2023-01-01');
const end = moment('2023-01-07');
const range = momentRange.range(start, end);
for (let day of range.by('day')) {
console.log(day.format('YYYY-MM-DD'));
}
Date Range Intersections
This feature allows you to find the intersection of two date ranges. The code sample finds the intersection of two date ranges: January 1, 2023, to June 30, 2023, and April 1, 2023, to December 31, 2023.
const moment = require('moment');
const { extendMoment } = require('moment-range');
const momentRange = extendMoment(moment);
const range1 = momentRange.range('2023-01-01', '2023-06-30');
const range2 = momentRange.range('2023-04-01', '2023-12-31');
const intersection = range1.intersect(range2);
console.log(intersection.toString()); // 2023-04-01 - 2023-06-30
date-fns is a modern JavaScript date utility library that provides a comprehensive set of functions for manipulating dates. Unlike moment-range, date-fns is modular, allowing you to import only the functions you need. It also has a smaller footprint and better performance.
Luxon is a modern JavaScript library for working with dates and times. It is a successor to Moment.js and offers a more powerful and flexible API. Luxon supports date ranges through its Interval class, which provides similar functionality to moment-range.
Day.js is a minimalist JavaScript library that parses, validates, manipulates, and displays dates and times. It is a lightweight alternative to Moment.js with a similar API. Day.js can be extended with plugins to support date range functionality, making it a versatile option.
Fancy date ranges for Moment.js.
Create a date range:
var start = new Date(2012, 0, 15);
var end = new Date(2012, 4, 23);
var range = moment().range(start, end);
You can also create a date range with moment objects:
var start = moment("2011-04-15", "YYYY-MM-DD");
var end = moment("2011-11-27", "YYYY-MM-DD");
var range = moment().range(start, end);
Arrays work too:
var dates = [moment("2011-04-15", "YYYY-MM-DD"), moment("2011-11-27", "YYYY-MM-DD")];
var range = moment().range(dates);
Check to see if your range contains a date/moment:
var start = new Date(2012, 4, 1);
var end = new Date(2012, 4, 23);
var lol = new Date(2012, 4, 15);
var wat = new Date(2012, 4, 27);
var range = moment().range(start, end);
var range2 = moment().range(lol, wat);
range.contains(lol); // true
range.contains(wat); // false
A optional second parameter indicates if the end of the range should be excluded when testing for inclusion
range.contains(end) // true
range.contains(end, false) // true
range.contains(end, true) // false
Find out if your moment falls within a date range:
var start = new Date(2012, 4, 1);
var end = new Date(2012, 4, 23);
var when = moment("2012-05-10", "YYYY-MM-DD");
var range = moment().range(start, end);
when.within(range); // true
Does it overlap another range?
range.overlaps(range2); // true
What are the intersecting ranges?
range.intersect(range2); // [moment().range(lol, end)]
Add/combine/merge overlapping ranges.
range.add(range2); // [moment().range(start, wat)]
var range3 = moment.range(new Date(2012, 3, 1), new Date(2012, 3, 15);
range.add(range3); // [null]
Subtracting one range from another.
range.subtract(range2); // [moment().range(start, lol)]
Iterate over your date range by an amount of time or another range:
var start = new Date(2012, 2, 1);
var two = new Date(2012, 2, 2);
var end = new Date(2012, 2, 5);
var range1 = moment().range(start, end);
var range2 = moment().range(start, two); // One day
var acc = [];
range1.by('days', function(moment) {
// Do something with `moment`
});
Any of the units accepted by moment.js' add
method may be used.
You can also iterate by another range:
range1.by(range2, function(moment) {
// Do something with `moment`
acc.push(moment);
});
acc.length == 5 // true
Iteration also supports excluding the end value of the range by setting the
last parameter to true
.
acc2 = [];
range1.by('d', function (moment) {
acc2.push(moment)
}, true);
acc2.length == 4 // true
Compare range lengths or add them together with simple math:
var r_1 = moment().range(new Date(2011, 2, 5), new Date(2011, 3, 15));
var r_2 = moment().range(new Date(1995, 0, 1), new Date(1995, 12, 25));
r_2 > r_1 // true
r_1 + r_2 // duration of both ranges in milliseconds
Math.abs(r_1 - r_2); // difference of ranges in milliseconds
Check if two ranges are the same, i.e. their starts and ends are the same:
var r_1 = moment().range(new Date(2011, 2, 5), new Date(2011, 3, 15));
var r_2 = moment().range(new Date(2011, 2, 5), new Date(2011, 3, 15));
var r_3 = moment().range(new Date(2011, 3, 5), new Date(2011, 6, 15));
r_1.isSame(r_2); // true
r_2.isSame(r_3); // false
The difference of the entire range given various units.
Any of the units accepted by moment.js' add
method may be used.
var start = new Date(2011, 2, 5);
var end = new Date(2011, 5, 5);
var dr = moment.range(start, end);
dr.diff('months'); // 3
dr.diff('days'); // 92
dr.diff(); // 7945200000
toDate
var start = new Date(2011, 2, 5);
var end = new Date(2011, 5, 5);
var dr = moment.range(start, end);
dr.toDate(); // [new Date(2011, 2, 5), new Date(2011, 5, 5)]
Calculate the center of a range
var start = new Date(2011, 2, 5);
var end = new Date(2011, 3, 5);
var dr = moment.range(start, end);
dr.center(); // 1300622400000
Deep clone a range
var start = new Date(2011, 2, 5);
var end = new Date(2011, 3, 5);
var dr = moment.range(start, end);
var dr2 = dr.clone();
dr2.start.add(2, 'days');
dr2.start.toDate() === dr.start.toDate() // false
moment-range works in both the browser and node.js.
Simply include moment-range after moment.js:
<script src="/javascripts/moment-range.js"></script>
Thanks to the fine people at cdnjs, you can link to moment-range from the cdnjs servers.
Install via npm:
npm install moment-range
Or put it in your package.json
:
{ "moment-range": "~1" }
Note: Include moment-range
after moment
.
bower install moment-range
Note: Include moment-range
after moment
.
Clone this bad boy:
git clone https://git@github.com/gf3/moment-range.git
Install the dependencies:
npm install
Do all the things!
npm build
npm test
moment-range is UNLICENSED.
FAQs
Fancy date ranges for Moment.js
The npm package moment-range receives a total of 237,700 weekly downloads. As such, moment-range popularity was classified as popular.
We found that moment-range demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.