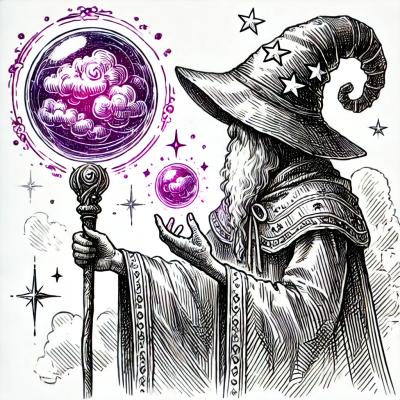
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
mongodb-memory-server-core
Advanced tools
MongoDB Server for testing (core package, without autodownload). The server will allow you to connect your favourite ODM or client library to the MongoDB Server and run parallel integration tests isolated from each other.
The mongodb-memory-server-core package provides an in-memory MongoDB server for testing purposes. It allows developers to spin up a MongoDB server instance that runs entirely in memory, making it ideal for unit tests and integration tests where a real MongoDB server would be overkill or too slow.
Start an In-Memory MongoDB Server
This feature allows you to start an in-memory MongoDB server instance. The code sample demonstrates how to create a new MongoMemoryServer instance and retrieve its URI.
const { MongoMemoryServer } = require('mongodb-memory-server-core');
(async () => {
const mongod = new MongoMemoryServer();
const uri = await mongod.getUri();
console.log(`MongoDB Server started at ${uri}`);
})();
Stop the In-Memory MongoDB Server
This feature allows you to stop the in-memory MongoDB server instance. The code sample demonstrates how to start and then stop the MongoMemoryServer instance.
const { MongoMemoryServer } = require('mongodb-memory-server-core');
(async () => {
const mongod = new MongoMemoryServer();
await mongod.start();
console.log('MongoDB Server started');
await mongod.stop();
console.log('MongoDB Server stopped');
})();
Use with Mongoose
This feature allows you to use the in-memory MongoDB server with Mongoose. The code sample demonstrates how to connect Mongoose to the in-memory MongoDB server, perform database operations, and then disconnect and stop the server.
const mongoose = require('mongoose');
const { MongoMemoryServer } = require('mongodb-memory-server-core');
(async () => {
const mongod = new MongoMemoryServer();
const uri = await mongod.getUri();
await mongoose.connect(uri, { useNewUrlParser: true, useUnifiedTopology: true });
console.log('Mongoose connected to in-memory MongoDB');
// Perform database operations
await mongoose.disconnect();
await mongod.stop();
})();
Mockgoose is a library that provides a mock to mongoose by using an in-memory database. It is similar to mongodb-memory-server-core in that it allows for in-memory MongoDB instances, but it is specifically designed to work with Mongoose.
Mongodb-memory-server is a higher-level package that wraps around mongodb-memory-server-core and provides additional features and utilities. It is more user-friendly and includes more out-of-the-box functionality compared to mongodb-memory-server-core.
Mongo-unit is a library that provides utilities for unit testing with MongoDB. It allows you to start and stop in-memory MongoDB instances and load initial data. It is similar to mongodb-memory-server-core but includes additional utilities for unit testing.
Core package which contains main code. Used in all other packages.
8.6.0 (2022-05-23)
FAQs
MongoDB Server for testing (core package, without autodownload). The server will allow you to connect your favourite ODM or client library to the MongoDB Server and run parallel integration tests isolated from each other.
The npm package mongodb-memory-server-core receives a total of 501,732 weekly downloads. As such, mongodb-memory-server-core popularity was classified as popular.
We found that mongodb-memory-server-core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.