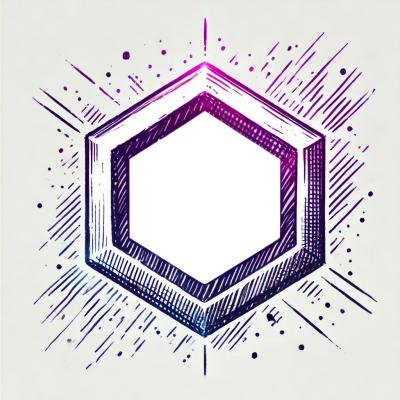
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
mongoose-slug-updater
Advanced tools
➔ Schema-based slug plugin for Mongoose ✓ single⋮compound ✓ unique within collection⋮group ✓ nested docs⋮arrays ✓ relative⋮abs paths ✓ sync on change: create⋮save⋮update⋮updateOne⋮updateMany⋮findOneAndUpdate ✓ $set operator ✓ counter⋮shortId
Sophisticated slugifier plugin for Mongoose.
Features:
create
, save
, update
, updateOne
, updateMany
or findOneAndUpdate
methods supported, and you can switch them on/off.The best way to install it is using npm
npm install mongoose-slug-updater --save
var slug = require('mongoose-slug-updater');
var mongoose = require('mongoose');
mongoose.plugin(slug);
This plugin is based on the idea of using the mongoose schema as the way to check the use of slug fields.
The plugin checks and updates automatically the slug field with the correct slug.
If you only want to create the slug based on a simple field.
var mongoose = require('mongoose'),
slug = require('mongoose-slug-updater'),
mongoose.plugin(slug),
Schema = mongoose.Schema,
schema = new Schema({
title: String,
slug: { type: String, slug: "title" }
});
You can add as many slug fields as you wish
var mongoose = require('mongoose'),
slug = require('mongoose-slug-updater'),
mongoose.plugin(slug),
Schema = mongoose.Schema,
schema = new Schema({
title: String,
subtitle: String,
slug: { type: String, slug: "title" },
slug2: { type: String, slug: "title" },
slug3: { type: String, slug: "subtitle" }
});
If you want, you can use more than one field in order to create a new slug field.
var mongoose = require('mongoose'),
slug = require('mongoose-slug-updater'),
mongoose.plugin(slug),
Schema = mongoose.Schema,
schema = new Schema({
title: String,
subtitle: String,
slug: { type: String, slug: ["title", "subtitle"] }
});
This option accepts a funtion which receives actual field value and can be used to tranform value before generating slug.
var mongoose = require('mongoose'),
slug = require('mongoose-slug-updater'),
mongoose.plugin(slug),
Schema = mongoose.Schema,
schema = new Schema({
title: String,
subtitle: String,
slug: { type: String, slug: ["title", "subtitle"], transform: v => stripHtmlTags(v) }
});
To create a unique slug field, you must only add add the unique: true parameter in the path (also, this way the default mongo unique index gets created)
var mongoose = require('mongoose'),
slug = require('mongoose-slug-updater'),
mongoose.plugin(slug),
Schema = mongoose.Schema,
schema = new Schema({
title: String,
subtitle: String,
slug: { type: String, slug: ["title", "subtitle"], unique: true }
});
If unique
or uniqueSlug
is set, the plugin searches in the mongo database, and if the slug already exists in the collection, it appends to the slug a separator (default: "-") and a random string (generated with the shortid module).
mongoose.model('Resource').create({
title: "Am I wrong, fallin' in love with you!",
subtitle: "tell me am I wrong, well, fallin' in love with you",
}); // slug -> 'am-i-wrong-fallin-in-love-with-you'
mongoose.model('Resource').create({
title: "Am I wrong, fallin' in love with you!",
subtitle: "tell me am I wrong, well, fallin' in love with you",
}); // slug -> 'am-i-wrong-fallin-in-love-with-you-Nyiy4wW9l'
mongoose.model('Resource').create({
title: "Am I wrong, fallin' in love with you!",
subtitle: "tell me am I wrong, well, fallin' in love with you",
}); // slug -> 'am-i-wrong-fallin-in-love-with-you-NJeskEPb5e'
Alternatively you can modify this behaviour and instead of appending a random string, an incremental counter will be used. For that to happen, you must use the parameter slugPaddingSize
specifying the total length of the counter:
var mongoose = require('mongoose'),
slug = require('mongoose-slug-updater'),
mongoose.plugin(slug),
Schema = mongoose.Schema,
schema = new Schema({
title: String,
subtitle: String,
slug: { type: String, slug: ["title", "subtitle"], slugPaddingSize: 4, unique: true }
});
mongoose.model('Resource').create({
title: 'Am I wrong, fallin\' in love with you!',
subtitle: "tell me am I wrong, well, fallin' in love with you"
}) // slug -> 'am-i-wrong-fallin-in-love-with-you'
mongoose.model('Resource').create({
title: 'Am I wrong, fallin\' in love with you!',
subtitle: "tell me am I wrong, well, fallin' in love with you"
}) // slug -> 'am-i-wrong-fallin-in-love-with-you-0001'
mongoose.model('Resource').create({
title: 'Am I wrong, fallin\' in love with you!',
subtitle: "tell me am I wrong, well, fallin' in love with you"
}) // slug -> 'am-i-wrong-fallin-in-love-with-you-0002'
If you don't want to define your field as unique for some reasons, but still need slug to be unique,
you can use uniqueSlug:true
option instead of unique
.
This option will not cause index creation, but still will be considered by the plugin.
forceIdSlug
option will append shortId even if no duplicates were found.
This is useful for applications with high chance of concurrent modification of unique fields.
Check for conflict made by plugin is not atomic with subsequent insert/update operation,
so there is a possibility of external change of data in the moment between check and write.
If this happened, mongo will throw unique index violation error.
Chances of such case higher for counter unique mode, but with shortId this is possible too.
You can just retry operation, so plugin will check collection again and regenerate correct unique slug.
Or you can set forceIdSlug
option - this will solve the problem completely, but you will pay for this by less readabilty of your slugs, because they will always be appended with random string.
In most cases write operations not so frequent to care about possible conflicts.
note: forceIdSlug
option will also overwite unique
to the true
, and slugPaddingSize
option will be ignored.
Sometimes you only want slugs to be unique within a specific group.
This is done with the uniqueGroupSlug
property which is an array of fields to group by:
ResourceGroupedUnique = new mongoose.Schema({
title: { type: String },
subtitle: { type: String },
group: { type: String },
uniqueSlug: {
type: String,
uniqueGroupSlug: ['group'],
slugPaddingSize: 4,
slug: 'title',
index: true,
},
});
mongoose.model('ResourceGroupedUnique').create({
title: "Am I wrong, fallin' in love with you!",
subtitle: "tell me am I wrong, well, fallin' in love with you",
group: 'group 1',
}); // slug -> 'am-i-wrong-fallin-in-love-with-you'
mongoose.model('ResourceGroupedUnique').create({
title: "Am I wrong, fallin' in love with you!",
subtitle: "tell me am I wrong, well, fallin' in love with you",
group: 'group 2',
}); // slug -> 'am-i-wrong-fallin-in-love-with-you'
mongoose.model('ResourceGroupedUnique').create({
title: "Am I wrong, fallin' in love with you!",
subtitle: "tell me am I wrong, well, fallin' in love with you",
group: 'group 1',
}); // slug -> 'am-i-wrong-fallin-in-love-with-you-0001'
mongoose.model('ResourceGroupedUnique').create({
title: "Am I wrong, fallin' in love with you!",
subtitle: "tell me am I wrong, well, fallin' in love with you",
group: 'group 2',
}); // slug -> 'am-i-wrong-fallin-in-love-with-you-0001'
Important: you must not have a unique: true
option, but it's a good idea to have an index: true
option.
MongoDB supports unique index for nested arrays elements, but he checks for duplication conflicts only on per-document basis, so inside document duplicate nested array's elements are still allowed.
mongoose-slug-updater works differently. It checks slug for duplicates both in current documentts's nested array and in other documents, considering uniqueGroupSlug option, if specified.
const UniqueNestedSchema = new mongoose.Schema({
children: [
{
subchildren: [
{
title: { type: String },
slug: {
type: String,
slug: 'title',
unique: true // 'global' unique slug
slugPaddingSize: 4,
},
slugLocal: {
type: String,
slug: 'title',
index: true,
slugPaddingSize: 4,
uniqueGroupSlug: '/_id',// slug unique within current document
},
},
],
},
],
});
mongoose.model('UniqueNestedSchema').create({
children:[
{
subchildren:[
{
title: "Am I wrong, fallin' in love with you!"
// slug -> 'am-i-wrong-fallin-in-love-with-you'
// slugLocal -> 'am-i-wrong-fallin-in-love-with-you'
},
{
title: "Am I wrong, fallin' in love with you!"
// slug -> 'am-i-wrong-fallin-in-love-with-you-0001'
// slugLocal -> 'am-i-wrong-fallin-in-love-with-you-0001'
},
]
},
{
subchildren:[
{
title: "Am I wrong, fallin' in love with you!"
// slug -> 'am-i-wrong-fallin-in-love-with-you-0002'
// slugLocal -> 'am-i-wrong-fallin-in-love-with-you-0002'
},
{
title: "Am I wrong, fallin' in love with you!"
// slug -> 'am-i-wrong-fallin-in-love-with-you-0003'
// slugLocal -> 'am-i-wrong-fallin-in-love-with-you-0003'
},
]
},
]
});
mongoose.model('UniqueNestedSchema').create({
children:[
{
subchildren:[
{
title: "Am I wrong, fallin' in love with you!"
// slug -> 'am-i-wrong-fallin-in-love-with-you-0004'
// slugLocal -> 'am-i-wrong-fallin-in-love-with-you'
},
{
title: "Am I wrong, fallin' in love with you!"
// slug -> 'am-i-wrong-fallin-in-love-with-you-0005'
// slugLocal -> 'am-i-wrong-fallin-in-love-with-you-0001'
},
]
},
{
subchildren:[
{
title: "Am I wrong, fallin' in love with you!"
// slug -> 'am-i-wrong-fallin-in-love-with-you-0006'
// slugLocal -> 'am-i-wrong-fallin-in-love-with-you-0002'
},
{
title: "Am I wrong, fallin' in love with you!"
// slug -> 'am-i-wrong-fallin-in-love-with-you-0007'
// slugLocal -> 'am-i-wrong-fallin-in-love-with-you-0003'
},
]
},
]
});
In case of change unique slug related fields (source fields from slug
option or group criteria from uniqueGroupSlug
)
slug will be regenerated considering latest existing duplicate. Presence or lack of the older duplicates, including original slug, will not be taken into account.
By default slugs will be created/updated for any related fields changed by any of create
(it's actually a save
too), save
, update
, updateOne
, updateMany
and findOneAndUpdate
operations.
You can specify which of supported methods should be watched:
const HooksSchema = new mongoose.Schema({
title: { type: String },
slug: {
type: String,
slug: 'title',
//by default all hooks are enabled
//slugOn:{ save: true, update: true, updateOne: true, updateMany: true, findOneAndUpdate: true }
},
slugNoSave: { type: String, slug: 'title', slugOn: { save: false } },
slugNoUpdate: { type: String, slug: 'title', slugOn: { update: false } },
slugNoUpdateOne: { type: String, slug: 'title', slugOn: { updateOne: false } },
slugNoUpdateMany: {
type: String,
slug: 'title',
slugOn: { updateMany: false },
},
slugNoFindOneAndUpdate: {
type: String,
slug: 'title',
slugOn: { findOneAndUpdate: false },
},
});
Note, that flags will affect both creation and updating of documents.
If you disabled save
and still want slug to be generated initially, create
method will not work,
becacuse mongoose emits save
event both for save
and create
methods.
Use upsert
option of update***
methods instead.
For update
and updateMany
methods multiply affected records also handled, but be careful with performance,
because one-by-one iteration over affected documents may happen in case of unique slugs.
In this case _id
field is required.
For update*
family of operations additional queries may be performed, to retrieve data missing in the query (fields not listed in the query but needed for compound or grouped unique slugs).
permanent
optionIf you want to generate slug initially, but keep it unchanged during further modifications of related fields, use permanent
flag like this:
ResourcePermanent = new mongoose.Schema({
title: { type: String },
subtitle: { type: String },
otherField: { type: String },
slug: { type: String, slug: ['title', 'subtitle'] }, //normal slug
titleSlug: { type: String, slug: 'title', permanent: true }, //permanent slug
subtitleSlug: {
type: String,
slug: 'subtitle',
permanent: true, //permanent option
slugPaddingSize: 4,
},
});
Nested docs and arrays declared inline right in the scheme or as a nested schemas declared separately are also supported.
Slug fields can be declared as relative or absolute(starting with slash) path to any point of current document.
Since MongoDB uses dot path notation, colon :
symbol used for relative paths as a reference to the parent, same as double dot ..
for file system paths.
Example of scheme with inline nested docs:
const InlineSchema = new mongoose.Schema({
// root title
title: { type: String },
// root slug with relative path to root title
slug: { type: String, slug: 'title' },
// root slug with absolute path to root title
absoluteSlug: { type: String, slug: '/title' },
// root slug with relative path to child title
childSlug: { type: String, slug: 'child.title' },
// root slug with absolute path to child title
absoluteChildSlug: { type: String, slug: '/child.title' },
// root slug with relative path to child's subchild title
subChildSlug: { type: String, slug: 'child.subChild.title' },
// root slug with relative path to the title of first children array element
childrenSlug0: { type: String, slug: 'children.0.title' },
// root slug with relative path to the title of 5th children array element
childrenSlug4: { type: String, slug: 'children.4.title' },
// root slug with relative path to the title of 4th subChildren' element of first children array element
subChildrenSlug3: { type: String, slug: 'children.0.subChildren.3.title' },
// root slug with relative path to the title of 8th subChildren' element of first children array element
subChildrenSlug7: { type: String, slug: 'children.0.subChildren.7.title' },
subChildrenSlug5SubChild: {
type: String,
// well, you see)
slug: 'children.0.subChildren.5.subChild.title',
},
subChildrenSlug2SubChild: {
type: String,
slug: 'children.0.subChildren.2.subChild.title',
},
child: {
title: { type: String },
// inside nested doc relative path starts from current object,
// so this is slug for child's title
slug: { type: String, slug: 'title' },
// absolute variant of path above, starting from root
absoluteSlug: { type: String, slug: '/child.title' },
// child's slug field generated for root title, absolute path
absoluteParentSlug: { type: String, slug: '/title' },
// relative path with parent reference `:`, so here root title will be used again.
relativeParentSlug: { type: String, slug: ':title' },
subChild: {
title: { type: String },
// relative path to the title of current nested doc,
// in absolute form it wil be /child.subChild.title
slug: { type: String, slug: 'title' },
// absolute path to the root title
absoluteParentSlug: { type: String, slug: '/title' },
// relative path to the parent title, /child.title in this case
relativeParentSlug: { type: String, slug: ':title' },
// parent of the parent is root, so ::title = /title here
relativeGrandParentSlug: { type: String, slug: '::title' },
},
},
// nested arrays work too
children: [
{
title: { type: String },
// title of current array element
slug: { type: String, slug: 'title' },
// root title
absoluteRootSlug: { type: String, slug: '/title' },
// child's title
absoluteChildSlug: { type: String, slug: '/child.title' },
// root title. Array itself not counted as a parent and skipped.
relativeRootSlug: { type: String, slug: ':title' },
// absolute path to 4th element of array
absoluteSiblingSlug: { type: String, slug: '/children.3.title' },
// same in relative form for 5th element
relativeSiblingSlug: { type: String, slug: ':children.4.title' },
subChild: {
title: { type: String },
// current title
slug: { type: String, slug: 'title' },
// root title
absoluteParentSlug: { type: String, slug: '/title' },
// child title
absoluteChildSlug: { type: String, slug: '/child.title' },
// title of current array element, because its a parent of this subChild
relativeParentSlug: { type: String, slug: ':title' },
// two parents up is a root
relativeGrandParentSlug: { type: String, slug: '::title' },
},
// arrays nested into array elements, welcome to the depth
subChildren: [
{
title: { type: String },
// current title
slug: { type: String, slug: 'title' },
// root title
absoluteRootSlug: { type: String, slug: '/title' },
// child title
absoluteChildSlug: { type: String, slug: '/child.title' },
// :--> children :--> root
relativeRootSlug: { type: String, slug: '::title' },
absoluteSiblingSlug: {
type: String,
// I don't know who will need it but it works, check yourself in /test
slug: '/children.0.subChildren.5.title',
},
// relative ref to another subChildren's element from current children's element
relativeSiblingSlug: { type: String, slug: ':subChildren.6.title' },
// hope you got it.
subChild: {
title: { type: String },
slug: { type: String, slug: 'title' },
absoluteParentSlug: { type: String, slug: '/title' },
absoluteChildSlug: { type: String, slug: '/child.title' },
relativeParentSlug: { type: String, slug: ':title' },
relativeGrandParentSlug: { type: String, slug: '::title' },
},
},
],
},
],
});
Example of nested schemas declared separately:
const SubChildSchema = new mongoose.Schema({
title: { type: String },
slug: { type: String, slug: 'title' },
absoluteRootSlug: { type: String, slug: '/title' },
absoluteChildSlug: { type: String, slug: '/child.title' },
relativeParentSlug: { type: String, slug: ':title' },// child's title
relativeGrandParentSlug: { type: String, slug: '::title' },//parent's title
});
const ChildSchema = new mongoose.Schema({
title: { type: String },
subChild: SubChildSchema,
subChildren: [SubChildSchema],
slug: { type: String, slug: 'title' },
subChildSlug: { type: String, slug: 'subChild.title' },
absoluteSlug: { type: String, slug: '/child.title' },
absoluteRootSlug: { type: String, slug: '/title' },
relativeParentSlug: { type: String, slug: ':title' },//Parent
subChildrenSlug2: { type: String, slug: 'subChildren.2.title' },
subChildrenSlug3: { type: String, slug: 'subChildren.3.title' },
});
const ParentSchema = new mongoose.Schema({
title: { type: String },
child: ChildSchema,
children: [ChildSchema],
slug: { type: String, slug: 'title' },
absoluteSlug: { type: String, slug: '/title' },
childSlug: { type: String, slug: 'child.title' },
absoluteChildSlug: { type: String, slug: '/child.title' },
subChildSlug: { type: String, slug: 'child.subChild.title' },
childrenSlug0: { type: String, slug: 'children.0.title' },
childrenSlug4: { type: String, slug: 'children.4.title' },
subChildrenSlug3: { type: String, slug: 'children.7.subChildren.3.title' },
subChildrenSlug7: { type: String, slug: 'children.3.subChildren.7.title' },
});
This will work too:
await SimpleInline.findOneAndUpdate(
{/*some criteria*/},
{
$set: {
title: 'New root title',
'child.title': 'New nested title',
'children.2.title': 'New title for the 3d item of nested array',
},
}
);
All the slugs which depend on modified titles will be found and regenerated.
This is recommended way to do partial modifications.
When you perform updates by object value instead of path:value list,
unobvious data loss may happen for nested docs or arrays, if they contain slugs affected by your modification.
Plugin always checks will current update operation be made with $set operator or not, and adds extra slug fields to the query as an object fields or $set paths accordingly.
So if you do have whole document you want to change - better use save
,
but if you dont have it, but you need to update some particular fields - it's more safe to use $set and paths:values.
You can change any options adding to the plugin
var mongoose = require('mongoose'),
slug = require('mongoose-slug-updater'),
options = {
separator: "-",
lang: "en",
truncate: 120,
backwardCompatible: true//support for the old options names used in the mongoose-slug-generator
},
mongoose.plugin(slug, options),
Schema = mongoose.Schema,
schema = new Schema({
title: String,
subtitle: String,
slug: { type: String, slug: ["title", "subtitle"], unique: true }
});
You can find more options in the speakingURL's npm page
This plugin is supported by Yuri Gor
This plugin was initially forked from mongoose-slug-generator, which is not maintained currently.
Merged and fixed uniqueGroupSlug
feature by rickogden.
update
, updateOne
, updateMany
and findOneAndUpdate
operations support implemented.
Nested docs and arrays support implemented.
Absolute and relative paths added.
Updating with $set operator and deep paths now works too.
All the update operators will be implemented soon.
Plugin rewritten with modern js and a lot of tests were added.
FAQs
➔ Schema-based slug plugin for Mongoose ✓ single⋮compound ✓ unique within collection⋮group ✓ nested docs⋮arrays ✓ relative⋮abs paths ✓ sync on change: create⋮save⋮update⋮updateOne⋮updateMany⋮findOneAndUpdate ✓ $set operator ✓ counter⋮shortId
The npm package mongoose-slug-updater receives a total of 0 weekly downloads. As such, mongoose-slug-updater popularity was classified as not popular.
We found that mongoose-slug-updater demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.