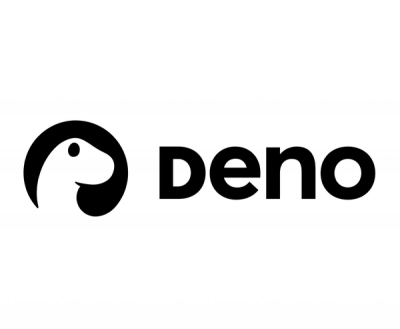
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
mongorito-tcomb
Advanced tools
npm i -S mongorito-tcomb
import Mongorito, {t, Model} from '../lib'
class Person extends Model {
get Schema() {
return t.struct({ // return a tcomb struct
name: t.String,
surname: t.maybe(t.String),
age: t.Number
})
}
}
(async () => {
try {
await Mongorito.connect('localhost/mongorito-tcomb') // Connect like with Mongorito
await Person.index('name', {unique: true}) // Ensure name is unique
var paul = new Person({name: 'paul', age: 42})
await paul.save()
await paul.remove()
await Mongorito.disconnect()
} catch (e) {
console.log(e.stack)
}
})()
patch: (Model) -> PatchedModel
The patch function can be useful to combine different Mongorito plugins. It take a class, extend it then return the extended class.
t.ID
It represent the ID of an element in the database.
Usage: t.ID(ctx, M)
where M is a Mongorito model and ctx the instance
of the model
It ensure that ID is valid and exist in the database
FAQs
Bring schema validation to Mongorito thanks to tcomb
The npm package mongorito-tcomb receives a total of 0 weekly downloads. As such, mongorito-tcomb popularity was classified as not popular.
We found that mongorito-tcomb demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.