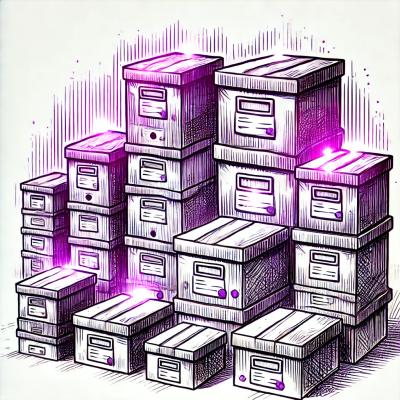
Security News
PyPI’s New Archival Feature Closes a Major Security Gap
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Awesome MongoDB ODM for Node.js apps. Just take a look at its beautiful models and API.
Uses official mongodb driver under the hood.
const mongorito = require('mongorito');
const Model = mongorito.Model;
class Post extends Model {
}
mongorito.connect('localhost/blog');
let post = new Post({
title: 'Steve Angello rocks',
author: {
name: 'Emma'
}
});
post.save();
// post saved
$ npm install mongorito --save
Check out connection example.
Here's how to connect to a blog
database on localhost
:
await mongorito.connect('localhost/blog');
To disconnect, use mongorito.disconnect()
:
await mongorito.disconnect();
Use classes to define models:
const Model = mongorito.Model;
class Post extends Model {
}
This defines model Post
with documents in posts
collection.
To use a custom collection, add collection()
method, which returns the name of the desired collection:
class Post extends Model {
collection () {
return 'super_cool_posts';
}
}
Mongorito models can also be defined old-fashioned Backbone way:
const Post = Model.extend({
collection: 'posts'
});
Note: collection
property has to be set.
Check out attributes example.
To create a new instance of a model, simply use new
:
let post = new Post({
title: 'Great title',
author: {
name: 'Emma'
}
});
To retrieve a specific attribute (even a nested one):
let title = post.get('title');
let author = post.get('author.name');
All attributes can be retrieved at once using either toJSON()
or get()
:
let attrs = post.toJSON();
let attrs = post.get();
Set new values via set()
method:
post.set('title', 'New title');
post.set('author.name', 'Rachel');
Check out manage example.
Use a save()
method to create/update (Mongorito handles that for you) a model:
let post = new Post();
await post.save(); // creates a new post
post.set('title', 'New title');
await post.save(); // updates an existing post
To remove a model from collection:
await post.remove();
You can also remove all models matching a certain criteria:
await Post.remove({ title: 'New title' });
To fetch all models find()
or all()
can be used (they're identical):
Post.find();
Post.all();
Post.findOne({ title: 'New title' });
Post.findById('56c9e0922cc9215110ab26dc');
Post.where('title', 'New title').find();
Post.where('author.name', 'Emma').find();
Post.where('title', /something/i).find();
Post.exists('title').find();
Post.where('comments_number').lt(5).find(); // less than 5
Post.where('comments_number').lte(5).find(); // less than or equal 5
Post.where('comments_number').gt(5).find(); // greater than 5
Post.where('comments_number').gte(5).find(); // greater than or equal 5
Post.in('comments_number', [4, 8]).find();
Find all models where title
equals either "First" or "Second":
Post.or({ title: 'First' }, { title: 'Second' }).find();
Post.limit(10).find();
Skip first N results:
Post.skip(4).find();
// descending
Post.sort('comments_number', -1);
// ascending
Post.sort('comments_number', 1);
Count all documents in collection:
Post.count();
Count all documents matching a certain criteria:
Post.count({ awesome: true });
$ npm test
Mongorito is released under the MIT License.
FAQs
ES6 generator-based MongoDB ODM.
The npm package mongorito receives a total of 104 weekly downloads. As such, mongorito popularity was classified as not popular.
We found that mongorito demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Research
Security News
Malicious npm package postcss-optimizer delivers BeaverTail malware, targeting developer systems; similarities to past campaigns suggest a North Korean connection.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.