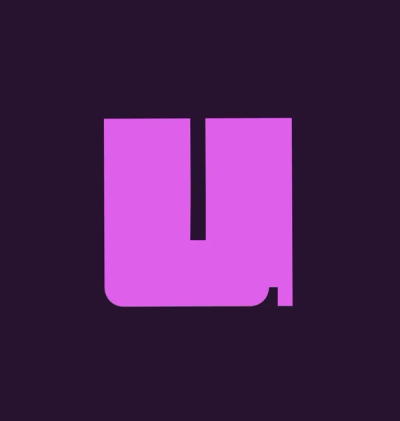
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
mqlight-dev
Advanced tools
MQ Light is designed to allow applications to exchange discrete pieces of information in the form of messages. This might sound a lot like TCP/IP networking, and MQ Light does use TCP/IP under the covers, but MQ Light takes away much of the complexity and provides a higher level set of abstractions to build your applications with.
This Node.js module provides the high-level API by which you can interact with the MQ Light runtime.
See https://developer.ibm.com/messaging/mq-light/ for more details.
Current Features:
You will need a Node.js 0.10 runtime environment to use the MQ Light API module. This can be installed from http://nodejs.org/download/, or by using your operating system's package manager.
The following are the currently supported platform architectures:
You will currently receive an error if you attempt to use any other combination.
Before using MQ Light on Linux, you will also need the 0.9.8 version of an OpenSSL package. This version of the package is not installed by default, so to use the module you will need to install it. For example:
sudo apt-get install libssl0.9.8
sudo yum install openssl098e
Additionally, you will also need to make sure you have the libuuid package installed. For example:
dpkg -l libuuid1
rpm -qa | grep libuuid
Install it in node.js:
npm install mqlight
OR
npm install https://ibm.biz/node-mqlight
var mqlight = require('mqlight');
Then create some clients to send and receive messages:
var recvClient = mqlight.createClient({
service: 'amqp://localhost',
id: 'recv_client_1'
});
var address = 'public';
recvClient.on('connected', function() {
recvClient.subscribe(address);
recvClient.on('message', function(data, delivery) {
console.log('Recv: %s', data);
});
});
recvClient.connect();
var sendClient = mqlight.createClient({
service: 'amqp://localhost',
id: 'send_client_1'
});
var topic = 'public';
sendClient.on('connected', function() {
sendClient.send(topic, 'Hello World!', function (err, data) {
console.log('Sent: %s', data);
sendClient.disconnect();
});
});
sendClient.connect();
options
])Creates an MQ Light client instance.
options
, (Object) options for the client. Properties include:
function(err, service)
). User names and
passwords may be embedded into the URL (e.g. amqp://user:pass@host
).AUTO_[0-9a-f]{7}
) (optional), a unique
identifier for this client. A client with a duplicate id
will be
prevented from connecting to the messaging service.Returns a Client
object representing the client instance. The client is an
event emitter and listeners can be registered for the following events:
connect
, disconnect
, error
, and message
.
callback
])Connects the MQ Light client instance to the service.
callback
- (Function) (optional) callback to be notified of errors &
completiontopic
, message
, [options
], [callback
])Sends the given MQ Light message object to the specified topic. String and Buffer messages will be sent and received as-is. Any other Object will be converted to JSON before sending and automatically parsed back into the same Object type when received.
topic
- (String) the topic to which the message will be sent.
A topic can contain any character in the Unicode character set.message
- (String | Buffer | Object) the message body to be sentoptions
- (Object) (optional) map of additional options for the send.
Supported options are:
callback
- (Function) (optional) callback to be notified of errors &
completionReturns true
if this message was sent, or is the next to be sent.
Returns false
if the message was queued in user memory, due to either a
backlog of messages, or because the client was not in a connected state.
When the backlog of messages is cleared, drain
will be emitted.
pattern
, [share
], [options
], [callback
])Create a Destination
and associates it with a pattern
.
The pattern
argument is matched against the topic
that messages are
sent to, allowing the messaging service to determine whether a paricular
message will be delivered to a particular Destination
, and hence
subscription
.
pattern
- (String) used to match against the topic
specified when a
message is sent to the messaging service. A pattern can contain any character
in the Unicode character set, with #
representing a multilevel wildcard and
+
a single level wildcard as described
here.share
- (String) (optional) name for creating or joining a shared
subscription for which messages are anycast between connected subscribers. If
omitted defaults to unshared (e.g. private to the client).options
- (Object) (optional) map of additional options for the destination.
Supported options are:
client.subscribe(...)
method will behave as if the maximum
value was specified. If this property is not specified then a value of 0
is assumed.callback
- (Function) callback to be notified of errors & completion.Returns the Client
object that the subscribe was called on. message
events
will be emitted when messages arrive.
Returns the identifier associated with the client. This will either be what
was passed in on the Client.createClient
call or an autogenerated id.
Returns the URL of the service to which the client is currently connected to, or undefined if not connected.
Returns the current state of the client, which will be one of: 'connected', 'connecting', 'disconnected' or 'disconnecting'.
Disconnects this Client from the messaging server and frees the system
resources that it uses. Calling this method also implicitly closes any
subscriptions that have been created using the client's
Client.subscribe
method.
Emitted when a message is delivered from a destination matching one of the client's subscriptions.
data
- (String | Buffer | Object) the message body.delivery
- (Object) additional information about why the event was emitted.
Properties include:
data
argument. Values are:
'text/plain' - data
will be a String.
'application/octet-stream' - data
will be a Buffer.
'application/json' - data
will be a JSON Object.This event is emitted when a client successfully connects to the messaging service.
This event is emitted when a client disconnects from the messaging service, either explicitly, or because the connection between the client and the service is interrupted.
Emitted when an error is detected that prevents or interrupts a client's connection to the messaging service.
error
(Error) the error.To run the samples, install the module via npm and navigate to the
mqlight/samples/
folder.
Usage:
Receiver Example:
Usage: recv.js [options]
Options:
-h, --help show this help message and exit
-s URL, --service=URL service to connect to (default: amqp://localhost)
-t TOPICPATTERN, --topic-pattern=TOPICPATTERN
subscribe to receive messages matching TOPICPATTERN
(default: public)
-n NAME, --share-name NAME
optionally, subscribe to a shared destination using
NAME as the share name.
Sender Example:
Usage: send.js [options] <msg_1> ... <msg_n>
Options:
-h, --help show this help message and exit
-s URL, --service=URL service to connect to (default: amqp://localhost)
-t TOPIC, --topic=TOPIC
send messages to topic TOPIC (default: public)
-d NUM, --delay=NUM add a NUM seconds time delay between each request
You can help shape the product we release by trying out the beta code and leaving your feedback.
If you think you've found a bug, please leave us
feedback.
To help us fix the bug a log might be helpful. You can get a log by setting the
environment variable MQLIGHT_NODE_LOG
to debug
and by collecting the output
that goes to stderr when you run your application.
FAQs
IBM MQ Light Client Module
The npm package mqlight-dev receives a total of 5,394 weekly downloads. As such, mqlight-dev popularity was classified as popular.
We found that mqlight-dev demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.