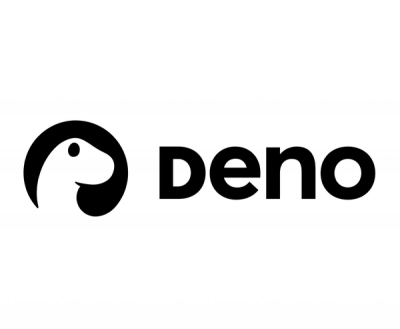
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
The mqtt npm package is a client library for the MQTT protocol, which is a lightweight messaging protocol designed for small sensors and mobile devices. It allows you to connect to an MQTT broker, publish messages to topics, and subscribe to topics to receive messages.
Connect to an MQTT broker
This feature allows you to connect to an MQTT broker. The code sample demonstrates how to connect to a public MQTT broker (HiveMQ) and log a message upon successful connection.
const mqtt = require('mqtt');
const client = mqtt.connect('mqtt://broker.hivemq.com');
client.on('connect', () => {
console.log('Connected to broker');
});
Publish messages to a topic
This feature allows you to publish messages to a specific topic. The code sample shows how to publish a message 'Hello MQTT' to the topic 'test/topic' after connecting to the broker.
const mqtt = require('mqtt');
const client = mqtt.connect('mqtt://broker.hivemq.com');
client.on('connect', () => {
client.publish('test/topic', 'Hello MQTT');
console.log('Message published');
});
Subscribe to a topic
This feature allows you to subscribe to a specific topic and receive messages published to that topic. The code sample demonstrates subscribing to 'test/topic' and logging any received messages.
const mqtt = require('mqtt');
const client = mqtt.connect('mqtt://broker.hivemq.com');
client.on('connect', () => {
client.subscribe('test/topic', (err) => {
if (!err) {
console.log('Subscribed to topic');
}
});
});
client.on('message', (topic, message) => {
console.log(`Received message: ${message.toString()} on topic: ${topic}`);
});
mqttjs is another popular MQTT client library for Node.js. It offers similar functionalities to the mqtt package, including connecting to brokers, publishing, and subscribing to topics. It is known for its simplicity and ease of use.
aedes is a barebone MQTT broker that can be embedded in Node.js applications. While it serves as a broker rather than a client, it can be used in conjunction with mqtt to create a complete MQTT solution. It is lightweight and highly performant.
mosca is an MQTT broker that can be used with Node.js. It is designed to be fast and scalable, making it suitable for IoT applications. Like aedes, it can be used alongside mqtt to provide a full MQTT ecosystem.
mqtt.js library for the MQTT protocol, written in javascript.
It allows the creation of both MQTT clients and MQTT brokers
through the createClient
and createServer
API methods.
Much of this document requires an understanding of the MQTT protocol, so consult the MQTT documentation for more information.
npm install mqttjs
This project also contains two extremely simple MQTT clients bin/mqtt_pub
and bin/mqtt_sub
can be executed from the command line in the following ways:
mqtt_pub <port> <host> <topic> <payload>
mqtt_sub <port> <host> <topic>
where
port
is the port the MQTT server is listening onhost
is the MQTT server's hosttopic
is the topic to publish/subscribe topayload
is the payload to publishThese are expected to improve as the project goes on.
A broadcast server example, included in examples/broadcast.js
:
var mqtt = require('../mqtt');
mqtt.createServer(function(client) {
var self = this;
if (!self.clients) self.clients = {};
client.on('connect', function(packet) {
client.connack({returnCode: 0});
client.id = packet.client;
self.clients[client.id] = client;
});
client.on('publish', function(packet) {
for (var k in self.clients) {
self.clients[k].publish({topic: packet.topic, payload: packet.payload});
}
});
client.on('subscribe', function(packet) {
var granted = [];
for (var i = 0; i < packet.subscriptions.length; i++) {
granted.push(packet.subscriptions[i].qos);
}
client.suback({granted: granted});
});
client.on('pingreq', function(packet) {
client.pingresp();
});
client.on('disconnect', function(packet) {
client.stream.end();
});
client.on('close', function(err) {
delete self.clients[client.id];
});
client.on('error', function(err) {
client.stream.end();
util.log('error!');
});
}).listen(1883);
A basic publish client, the basis for bin/mqtt_pub
:
var mqtt = require('../lib/mqtt');
var argv = process.argv;
for (var i = 2; i <= 5; i++) {
if(!argv[i]) process.exit(-1);
}
var port = argv[2],
host = argv[3],
topic = argv[4],
payload = argv[5];
mqtt.createClient(port, host, function(err, client) {
if (err) process.exit(1);
client.connect({keepalive: 3000});
client.on('connack', function(packet) {
if (packet.returnCode === 0) {
client.publish({topic: topic, payload: payload});
client.disconnect();
} else {
console.log('connack error %d', packet.returnCode);
process.exit(-1);
}
});
client.on('close', function() {
process.exit(0);
});
client.on('error', function(e) {
console.log('error %s', e);
process.exit(-1);
});
});
The mqtt
module provides two methods for creating MQTT servers and clients
as specified below:
Creates a new mqtt.Server
. The listener argument is set as a listener for
the client
event.
Creates a new mqtt.Client
and connects it to the specified port
and host
.
If port
and host
are omitted 1883
and localhost
will be assumed for
each respectively.
When the client is connected, the connected
event will be fired and callback
will be called, if supplied. If connection fails for any reason, the error
parameter of callback will bet set to the error. Otherwise it will be null
and the client parameter will be the newly created client.
##mqtt.Server
The mqtt.Server
class represents an MQTT server.
mqtt.Server
extends net.Server
and so shares all of its methods with the
distinction that the net.Server#connected
event is caught and the client
event is fired.
function(client) {}
Emitted when a new TCP connection is received. client
is an instance of
mqtt.Client
.
##mqtt.Client
The mqtt.Client
class represents a connected MQTT client, be it on the server
or client side.
It is preferred that mqtt.Client
s be constructed using the mqtt.createClient
factory method.
For all methods below invalid options
will cause the method to return false
.
For all events below packet
will also contain all of the information contained
in the MQTT static header. This includes cmd
, dup
, qos
and retain
even
when they do not apply to the packet. It will also contain the length
of the packet.
###client.connect([options]) Send an MQTT connect packet.
options
is an object with the following defaults:
{ "version": "MQIsdp",
"versionNum": 3,
"keepalive": 60,
"client": "mqtt_" + process.pid,
}
options
supports the following properties:
version
: version string, defaults to MQIsdp
. Must be a string
versionNum
: version number, defaults to 3
. Must be a number
keepalive
: keepalive period, defaults to 60
. Must be a number
between 0
and 65535
client
: the client ID supplied for the session, defaults to mqtt_<pid>
. string
will
: the client's will message options. object
that supports the following properties:
topic
: the will topicpayload
: the will payloadqos
: the qos level to publish the will message withretain
: whether or not to retain the will messageclean
: the 'clean start' flag. boolean
username
: username for protocol v3.1. string
password
: password for protocol v3.1. string
###client.connack([options]) Send an MQTT connack packet.
options
is an object with the following defaults:
{ "returnCode": 0 }
options
supports the following properties:
returnCode
: the return code of the connack, defaults to 0
. Must be a number
between 0
and 5
###client.publish([options]) Send an MQTT publish packet.
options
is an object with the following defaults:
{ "messageId": Math.floor(65535 * Math.random()),
"payload": "",
"qos": 0,
"retain": false
}
options
supports the following properties:
topic
: the topic to publish to. string
payload
: the payload to publish, defaults to an empty buffer. string
or buffer
qos
: the quality of service level to publish on. number
between 0
and 2
messageId
: the message ID of the packet, defaults to a random integer between 0
and 65535
. number
retain
: whether or not to retain the published message. boolean
###client.['puback', 'pubrec', 'pubcomp', 'unsuback']([options])
Send an MQTT [puback, pubrec, pubcomp, unsuback]
packet.
options
supports the following properties:
messageId
: the ID of the packet###client.pubrel([options]) Send an MQTT pubrel packet.
options
is an object with the following defaults:
{ "dup": false }
options
supports the following properties:
dup
: duplicate message flagmessageId
: the ID of the packet###client.subscribe([options]) Send an MQTT subscribe packet.
options
is an object with the following defaults:
{ "dup": false,
"messageId": Math.floor(65535 * Math.random())
}
options
supports the following properties:
dup
: duplicate message flagmessageId
: the ID of the packetAnd either:
topic
: the topic to subscribe toqos
: the requested QoS subscription levelOr:
subscriptions
: a list of subscriptions of the form [{topic: a, qos: 0}, {topic: b, qos: 1}]
or of the form ['a', 'b']###client.suback([options]) Send an MQTT suback packet.
options
is an object with the following defaults:
{ "granted": [0],
"messageId": Math.floor(65535 * Math.random())
}
options
supports the following properties:
granted
: a vector of granted QoS levels, of the form [0, 1, 2]
messageId
: the ID of the packet###client.unsubscribe([options]) Send an MQTT unsubscribe packet.
options
is an object with the following defaults:
{ "messageId": Math.floor(65535 * Math.random()) }
options
supports the following properties:
messageId
: the ID of the packetdup
: duplicate message flagAnd either:
topic
: the topic to unsubscribe fromOr:
unsubscriptions
: a list of topics to unsubscribe from, of the form ["topic1", "topic2"]
###client.['pingreq', 'pingresp', 'disconnect']()
Send an MQTT [pingreq, pingresp, disconnect]
packet.
###Event: 'connected'
function() {}
Emitted when the socket underlying the mqtt.Client
is connected.
Note: only emitted by clients created using mqtt.createClient()
.
###Event: 'connect'
function(packet) {}
Emitted when an MQTT connect packet is received by the client.
packet
is an object that may have the following properties:
version
: the protocol version string
versionNum
: the protocol version number
keepalive
: the client's keepalive period
client
: the client's ID
will
: an object of the form:
{ "topic": "topic", "payload": "payload", "retain": false, "qos": 0 }
where topic
is the client's will topic, payload
is its will message,
retain
is whether or not to retain the will message and qos
is the
QoS of the will message.
clean
: clean start flag
username
: v3.1 username
password
: v3.1 password
###Event: 'connack'
function(packet) {}
Emitted when an MQTT connack packet is received by the client.
packet
is an object that may have the following properties:
returnCode
: the return code of the connack packet###Event: 'publish'
function(packet) {}
Emitted when an MQTT publish packet is received by the client.
packet
is an object that may have the following properties:
topic
: the topic the message is published onpayload
: the payload of the messagemessageId
: the ID of the packetqos
: the QoS level to publish at###Events: ['puback', 'pubrec', 'pubrel', 'pubcomp', 'unsuback']
function(packet) {}
Emitted when an MQTT [puback, pubrec, pubrel, pubcomp, unsuback]
packet
is received by the client.
packet
is an object that may contain the property:
messageId
: the ID of the packet###Event: 'subscribe'
function(packet) {}
Emitted when an MQTT subscribe packet is received.
packet
is an object that may contain the properties:
messageId
: the ID of the packetsubscriptions
: a list of topics and their requested QoS level, of the form [{topic: 'a', qos: 0},...]
###Event: 'suback'
function(packet) {}
Emitted when an MQTT suback packet is received.
packet
is an object that may contain the properties:
messageId
: the ID of the packetgranted
: a vector of granted QoS levels###Event: 'unsubscribe'
function(packet) {}
Emitted when an MQTT unsubscribe packet is received.
packet
is an object that may contain the properties:
messageId
: the ID of the packetunsubscriptions
: a list of topics the client is unsubscribing from, of the form [topic1, topic2, ...]
###Events: ['pingreq', 'pingresp', 'disconnect']
function(packet){}
Emitted when an MQTT [pingreq, pingresp, disconnect]
packet is received.
packet
is an empty object and can be ignored.
FAQs
A library for the MQTT protocol
The npm package mqtt receives a total of 965,658 weekly downloads. As such, mqtt popularity was classified as popular.
We found that mqtt demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.