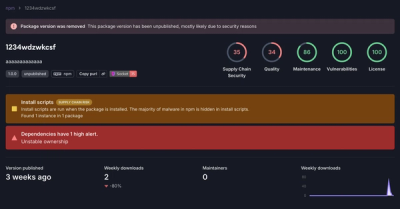
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
The mqtt npm package is a client library for the MQTT protocol, which is a lightweight messaging protocol designed for small sensors and mobile devices. It allows you to connect to an MQTT broker, publish messages to topics, and subscribe to topics to receive messages.
Connect to an MQTT broker
This feature allows you to connect to an MQTT broker. The code sample demonstrates how to connect to a public MQTT broker (HiveMQ) and log a message upon successful connection.
const mqtt = require('mqtt');
const client = mqtt.connect('mqtt://broker.hivemq.com');
client.on('connect', () => {
console.log('Connected to broker');
});
Publish messages to a topic
This feature allows you to publish messages to a specific topic. The code sample shows how to publish a message 'Hello MQTT' to the topic 'test/topic' after connecting to the broker.
const mqtt = require('mqtt');
const client = mqtt.connect('mqtt://broker.hivemq.com');
client.on('connect', () => {
client.publish('test/topic', 'Hello MQTT');
console.log('Message published');
});
Subscribe to a topic
This feature allows you to subscribe to a specific topic and receive messages published to that topic. The code sample demonstrates subscribing to 'test/topic' and logging any received messages.
const mqtt = require('mqtt');
const client = mqtt.connect('mqtt://broker.hivemq.com');
client.on('connect', () => {
client.subscribe('test/topic', (err) => {
if (!err) {
console.log('Subscribed to topic');
}
});
});
client.on('message', (topic, message) => {
console.log(`Received message: ${message.toString()} on topic: ${topic}`);
});
mqttjs is another popular MQTT client library for Node.js. It offers similar functionalities to the mqtt package, including connecting to brokers, publishing, and subscribing to topics. It is known for its simplicity and ease of use.
aedes is a barebone MQTT broker that can be embedded in Node.js applications. While it serves as a broker rather than a client, it can be used in conjunction with mqtt to create a complete MQTT solution. It is lightweight and highly performant.
mosca is an MQTT broker that can be used with Node.js. It is designed to be fast and scalable, making it suitable for IoT applications. Like aedes, it can be used alongside mqtt to provide a full MQTT ecosystem.
mqtt.js is a library for the MQTT protocol, written in JavaScript to be used in node.js.
v0.3.0 improves connection stability, performance, the reconnection
logic and SSL support. See #118 for
details. A Connection is a Writable stream, so you can run
MQTT.js over any kind of Stream (doc needed). Both the constructors of
MqttClient and MqttConnection changed, but not the factory method
mqtt.createClient
and mqtt.createConnection
.
v0.2.0 has brough some API breaking changes to mqtt.js. Please consult the migration guide for information or open an issue if you need any help.
npm install mqtt
First you will need to install and run a broker, such as Mosquitto or Mosca, and launch it.
For the sake of simplicity, let's put the subscriber and the publisher in the same file:
var mqtt = require('mqtt')
client = mqtt.createClient(1883, 'localhost');
client.subscribe('presence');
client.publish('presence', 'Hello mqtt');
client.on('message', function (topic, message) {
console.log(message);
});
client.end();
output:
Hello mqtt
If you do not want to install a separate broker, you can try using the server/orig example. It implements enough of the semantics of the MQTT protocol to run the example.
Detailed documentation can be found in the wiki
See: examples/client
var mqtt = require('mqtt')
, client = mqtt.createClient();
client.publish('messages', 'mqtt');
client.publish('messages', 'is pretty cool');
client.publish('messages', 'remember that!', {retain: true});
client.end();
var mqtt = require('mqtt')
, client = mqtt.createClient();
client.subscribe('messages');
client.publish('messages', 'hello me!');
client.on('message', function(topic, message) {
console.log(message);
});
client.options.reconnectPeriod = 0; // disable automatic reconnect
var mqtt = require('mqtt')
, client = mqtt.createClient();
client
.subscribe('messages')
.publish('presence', 'bin hier')
.on('message', function(topic, message) {
console.log(topic);
});
Included in examples/broadcast.js:
var mqtt = require('mqtt');
mqtt.createServer(function(client) {
var self = this;
if (!self.clients) self.clients = {};
client.on('connect', function(packet) {
client.connack({returnCode: 0});
client.id = packet.clientId;
self.clients[client.id] = client;
});
client.on('publish', function(packet) {
for (var k in self.clients) {
self.clients[k].publish({topic: packet.topic, payload: packet.payload});
}
});
client.on('subscribe', function(packet) {
var granted = [];
for (var i = 0; i < packet.subscriptions.length; i++) {
granted.push(packet.subscriptions[i].qos);
}
client.suback({granted: granted, messageId: packet.messageId});
});
client.on('pingreq', function(packet) {
client.pingresp();
});
client.on('disconnect', function(packet) {
client.stream.end();
});
client.on('close', function(err) {
delete self.clients[client.id];
});
client.on('error', function(err) {
client.stream.end();
console.log('error!');
});
}).listen(1883);
MIT
FAQs
A library for the MQTT protocol
The npm package mqtt receives a total of 825,823 weekly downloads. As such, mqtt popularity was classified as popular.
We found that mqtt demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.