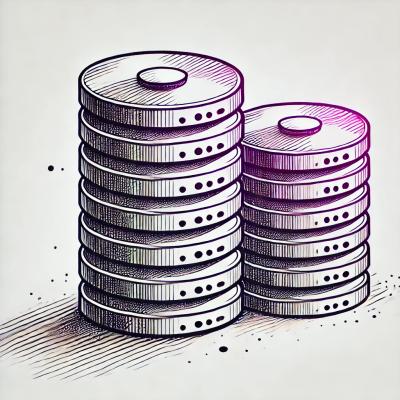
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
A small node.js library for msg91 sms api.
For more info visit MSG91
For Text SMS API docs Text SMS
For Voice SMS API docs Voice SMS
authkey : Login Authentication Key(This key is unique for every user)
number : single mobile number (Keep number in international format)
numbers : array of mobile numbers (Keep numbers in international format)
message : Message Content to send
senderid : Receiver will see this as sender's ID
route : If your operator supports multiple routes then give one route name. Eg: route=1 for promotional, route=4 for transactional SMS.
dialcode : 0 for international, 91 for India, 1 for USA
date & time : when you want to schedule the SMS to be sent. Time format will be yyyy-MM-dd & HH:mm:ss
senderno : Sender Mobile No
duration : Call duration
schtimestart : when you want to schedule the SMS to be sent. Time format will be yyyy-MM-dd & HH:mm:ss
schtimeend : when you want to schedule the SMS to be sent. Time format will be yyyy-MM-dd & HH:mm:ss
$ npm install msg91-sms
var msg91=require('msg91-sms');
//Authentication Key
var authkey='';
//for multiple numbers
var numbers=[];
numbers.push('');
//for single number
var number='';
//message
var message='';
//Sender ID
var senderid='';
//Route
var route='';
//Country dial code
var dialcode='';
//send to single number
msg91.sendOne(authkey,number,message,senderid,route,dialcode,function(response){
//Returns Message ID, If Sent Successfully or the appropriate Error Message
console.log(response);
});
//send to multiple numbers
msg91.sendMultiple(authkey,numbers,message,senderid,route,dialcode,function(response){
//Returns Message ID, If Sent Successfully or the appropriate Error Message
console.log(response);
});
var msg91=require('msg91-sms');
//Authentication Key
var authkey='';
//for multiple numbers
var numbers=[];
numbers.push('');
//for single number
var number='';
//message
var message='';
//Sender ID
var senderid='';
//Route
var route='';
//Country dial code
var dialcode='';
//send to single number
msg91.sendOnewithUnicode(authkey,number,message,senderid,route,dialcode,function(response){
//Returns Message ID, If Sent Successfully or the appropriate Error Message
console.log(response);
});
//send to multiple numbers
msg91.sendMultiplewithUnicode(authkey,numbers,message,senderid,route,dialcode,function(response){
//Returns Message ID, If Sent Successfully or the appropriate Error Message
console.log(response);
});
var msg91=require('msg91-sms');
//Authentication Key
var authkey='';
//for multiple numbers
var numbers=[];
numbers.push('');
//for single number
var number='';
//message
var message='';
//Sender ID
var senderid='';
//Route
var route='';
//Country dial code
var dialcode='';
//send to single number
msg91.sendOnewithFlash(authkey,number,message,senderid,route,dialcode,function(response){
//Returns Message ID, If Sent Successfully or the appropriate Error Message
console.log(response);
});
//send to multiple numbers
msg91.sendMultiplewithFlash(authkey,numbers,message,senderid,route,dialcode,function(response){
//Returns Message ID, If Sent Successfully or the appropriate Error Message
console.log(response);
});
var msg91=require('msg91-sms');
//Authentication Key
var authkey='';
//for multiple numbers
var numbers=[];
numbers.push('');
//for single number
var number='';
//message
var message='';
//Sender ID
var senderid='';
//Route
var route='';
//Country dial code
var dialcode='';
//send to single number
msg91.sendOneandGetJson(authkey,number,message,senderid,route,dialcode,function(response){
//Returns Message ID, If Sent Successfully or the appropriate Error Message
console.log(response);
});
//send to multiple numbers
msg91.sendMultipleandGetJson(authkey,numbers,messages,senderid,route,dialcode,function(response){
//Returns Message ID, If Sent Successfully or the appropriate Error Message
console.log(response);
});
var msg91=require('msg91-sms');
//Authentication Key
var authkey='';
//for multiple numbers
var numbers=[];
numbers.push('');
//for single number
var number='';
//message
var message='';
//Sender ID
var senderid='';
//Route
var route='';
//Country dial code
var dialcode='';
//date and time if sheduled message
//date should be yyyy-MM-dd and time should be HH:mm:ss (24H format)
var date='2015-11-22';
var time='20:19:20';
//Schedule message
//date should be yyyy-MM-dd time should be HH:mm:ss (24H format)
//send to single number
msg91.scheduleOne(authkey,number,message,senderid,route,dialcode,date,time,function(response){
//Returns Message ID, If Sent Successfully or the appropriate Error Message
console.log(response);
});
//send to multiple numbers
msg91.scheduleMultiple(authkey,numbers,message,senderid,route,dialcode,date,time,function(response){
//Returns Message ID, If Sent Successfully or the appropriate Error Message
console.log(response);
});
var msg91=require('msg91-sms');
//Authentication Key
var authkey='';
//for multiple numbers
var numbers=[];
numbers.push('');
//for single number
var number='';
//message
var message='';
//Sender ID
var senderid='';
//Route
var route='';
//Country dial code
var dialcode='';
//date and time if sheduled message
//date should be yyyy-MM-dd and time should be HH:mm:ss (24H format)
var date='2015-11-22';
var time='20:19:20';
//Schedule message
//date should be yyyy-MM-dd time should be HH:mm:ss (24H format)
//Schedule message
//date should be yyyy-MM-dd time should be HH:mm:ss (24H format)
//send to single number
msg91.scheduleOnewithUnicode(authkey,number,message,senderid,route,dialcode,date,time,function(response){
//Returns Message ID, If Sent Successfully or the appropriate Error Message
console.log(response);
});
//send to multiple numbers
msg91.scheduleMultiplewithUnicode(authkey,numbers,message,senderid,route,dialcode,date,time,function(response){
//Returns Message ID, If Sent Successfully or the appropriate Error Message
console.log(response);
});
var msg91=require('msg91-sms');
//Authentication Key
var authkey='';
//message
var message='';
//Sender ID
var senderid='';
//group id of group
var groupid='';
msg91.sendtoGroup(authkey,message,senderid,groupid,function(response){
//Returns Message ID, If Sent Successfully or the appropriate Error Message
console.log(response);
});
var msg91=require('msg91-sms');
//Authentication Key
var authkey='';
//for multiple numbers
var numbers=[];
numbers.push('');
//for single number
var number='';
//Route
var route='';
//Name of the Draft file
var draft_file_name='';
//Name of the folder
var campaign='';
//Call duration
var duration='';
//sender mobile no
var senderno='';
//when you want to schedule voice sms
//date should be yyyy-MM-dd and time should be HH:mm:ss (24H format)
var schtimestart='2015-11-13 09:00:00';
var schtimeend='2015-12-13 23:42:20';
//send to single number
msg91.sendOneVoiceSmsusingDraft(authkey,number,draft_file_name,senderno,route,campaign,duration,schtimestart,schtimeend,function(response){
//Returns Message ID, If Sent Successfully or the appropriate Error Message
console.log(response);
});
//send to multiple numebrs
msg91.sendMVoiceSmsusingDraft(authkey,numbers,draft_file_name,senderno,route,campaign,duration,schtimestart,schtimeend,function(response){
//Returns Message ID, If Sent Successfully or the appropriate Error Message
console.log(response);
});
var msg91=require('msg91-sms');
//Authentication Key
var authkey='';
//for multiple numbers
var numbers=[];
numbers.push('');
//for single number
var number='';
//Route
var route='';
//URL path of the file
var url_file_name='';
//Name of the folder
var campaign='';
//Call duration
var duration='';
//sender mobile no
var senderno='';
//when you want to schedule voice sms
//date should be yyyy-MM-dd and time should be HH:mm:ss (24H format)
var schtimestart='2015-11-13 09:00:00';
var schtimeend='2015-12-13 23:42:20';
//send to single number
msg91.sendVoiceSmsusingUrl(authkey,number,url_file_name,senderno,route,campaign,duration,schtimestart,schtimeend,function(response){
//Returns Message ID, If Sent Successfully or the appropriate Error Message
console.log(response);
});
//send to multiple numebrs
msg91.sendMVoiceSmsusingUrl(authkey,numbers,url_file_name,senderno,route,campaign,duration,schtimestart,schtimeend,function(response){
//Returns Message ID, If Sent Successfully or the appropriate Error Message
console.log(response);
});
var msg91=require('msg91-sms');
//Authentication Key
var authkey='';
//Route
var route='';
msg91.checkBalance(authkey,route,function(response){
//get balance or the appropriate Error Message
console.log(response);
});
FAQs
A small node.js library for msg91 sms api
The npm package msg91-sms receives a total of 71 weekly downloads. As such, msg91-sms popularity was classified as not popular.
We found that msg91-sms demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.