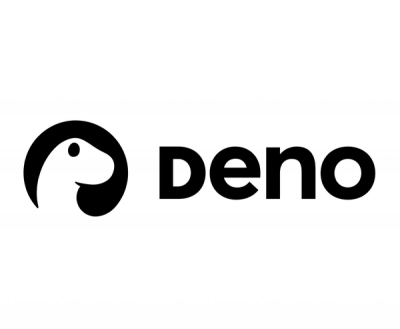
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
The msw (Mock Service Worker) npm package is a tool for mocking network requests at the service worker level. It allows developers to intercept and modify any outgoing HTTP requests from their application, which is useful for testing, development, and debugging purposes. It can be used in both browser and Node.js environments.
Mocking REST API requests
This feature allows you to intercept and mock responses to REST API requests. The code sample demonstrates how to set up a mock server that responds to a GET request to '/user' with a JSON object containing a username.
import { rest } from 'msw';
import { setupServer } from 'msw/node';
const server = setupServer(
rest.get('/user', (req, res, ctx) => {
return res(ctx.status(200), ctx.json({ username: 'admin' }));
})
);
beforeAll(() => server.listen());
afterEach(() => server.resetHandlers());
afterAll(() => server.close());
Mocking GraphQL API requests
This feature enables the mocking of GraphQL API requests. The code sample shows how to create a mock server that handles a GraphQL query named 'GetUser' and returns a response with user data.
import { graphql } from 'msw';
import { setupServer } from 'msw/node';
const server = setupServer(
graphql.query('GetUser', (req, res, ctx) => {
return res(ctx.data({ user: { id: '1', name: 'John Doe' } }));
})
);
beforeAll(() => server.listen());
afterEach(() => server.resetHandlers());
afterAll(() => server.close());
Delaying mocked responses
This feature allows you to simulate network delays in your mocked responses. The code sample sets up a mock server that delays the response to a GET request to '/user' by 1500 milliseconds.
import { rest } from 'msw';
import { setupServer } from 'msw/node';
const server = setupServer(
rest.get('/user', (req, res, ctx) => {
return res(ctx.delay(1500), ctx.json({ username: 'admin' }));
})
);
beforeAll(() => server.listen());
afterEach(() => server.resetHandlers());
afterAll(() => server.close());
Nock is a popular HTTP server mocking and expectations library for Node.js. It allows you to intercept HTTP requests and provide predefined responses. Unlike msw, which works with service workers and can be used in both browser and Node.js environments, nock is designed specifically for Node.js.
axios-mock-adapter is a library for mocking Axios requests. It provides a way to mock requests made using the Axios library, allowing you to specify the expected response for a given request. This package is more specific to Axios, while msw is agnostic to the HTTP client used.
jest-fetch-mock is a package that provides a way to easily mock fetch requests when using Jest. It's specifically tailored for Jest users and is used to mock the global fetch function. msw, on the other hand, intercepts requests at a lower level and is not limited to Jest or fetch.
Serverless offline client-side API mocking for your applications.
There are several points that I find annoying when conducting API mocking with any solution I've found:
This library aims to eradicate those problems, as it takes an entirely different approach to the client-side API mocking.
npm install msw --dev
Run the following command in your project's root directory:
msw create <rootDir>
Replace
rootDir
with the relative path to your server's root directory (i.e.msw create public
).In case you can't execute
msw
directly, trynode_modules/.bin/msw
as a local alternative.
This is going to copy the Mock Service Worker to the specified directory, so it could be served as a static file from your server. This makes it possible to be registered from the client application.
It's up to you where your mocks will reside. It's recommended, however, to isolate them into a separate module, which you can import on demand.
// app/mocks.js
import { msw } from 'msw'
/* Configure mocking routes */
msw.get(
'https://api.github.com/repo/:repoName',
(req, res, { status, set, delay, json }) => {
const { repoName } = req.params // access request's params
return res(
status(403), // set custom status
set({ 'Custom-Header': 'foo' }), // set headers
delay(1000), // delay the response
json({ errorMessage: `Repository "${repoName}" not found` }),
)
)
/* Start the Service Worker */
msw.start()
Import your mocks.js
module anywhere in the root of your application to enable the mocking:
// app/index.js
import './mocks.js'
Service Workers are designed as a caching tool. However, we don't want our mocking definitions to be cached, which may result into out-of-date logic during development.
It's highly recommend to enable "Update on reload" option in the "Application" tab of Chrome's DevTools (under "Service Workers" section). This will force Service Worker to update on each page reload, ensuring the latest logic is applied.
Read more on The Service Worker Lifecycle.
MSW uses Service Worker API with its primary ability to intercept requests, only instead of caching their responses it immitates them. In a nutshell, it works as follows:
resolver
function is executed, and its payload is returned as the mocked response.This library is meant to be used for development only. It doesn't require, nor encourage to install any Service Worker on the production environment.
Have an idea? Found a bug? Please communicate it through using the issues tab of this repositories. Pull requests are welcome as well!
FAQs
Seamless REST/GraphQL API mocking library for browser and Node.js.
The npm package msw receives a total of 3,672,277 weekly downloads. As such, msw popularity was classified as popular.
We found that msw demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.