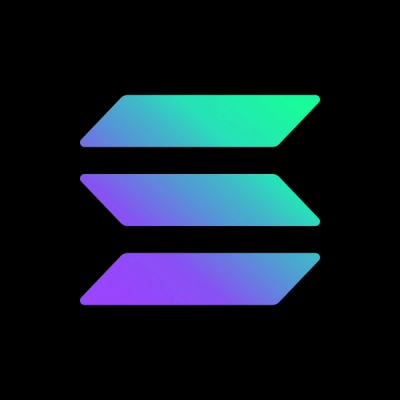
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
mui-daterange-picker-plus
Advanced tools
A modern Typescript/JavaScript library for simplifying date-range related operations and enhancing date-picker components in web applications. It provides a range of utilities, including date formatting, parsing, and a fully customizable date range picker
An advanced and highly customizable Date Range Picker component for Material-UI (MUI).
View Demo here ✨
Install the MUI Date Range Picker package via npm:
npm install mui-daterange-picker-plus
import { useState } from "react";
import Button from "@mui/material/Button";
import { PickerModal } from "mui-daterange-picker-plus/dist";
import type { DateRange } from "mui-daterange-picker-plus/dist";
export default function YourComponent() {
// state + handlers for the Modal
const [anchorEl, setAnchorEl] = useState<HTMLButtonElement | null>(null);
const handleClick = (event: React.MouseEvent<HTMLButtonElement>) => {
setAnchorEl(event.currentTarget);
};
const handleClose = () => {
setAnchorEl(null);
};
const open = Boolean(anchorEl);
// state + handlers for the DateRange Value
const [dateRangeOnChange, setDateRangeOnChange] = useState<DateRange>({});
const [dateRangeOnSubmit, setDateRangeOnSubmit] = useState<DateRange>({});
const handleSetDateRangeOnChange = (dateRange: DateRange) => {
setDateRangeOnChange(dateRange);
handleSetDateRangeOnSubmit({});
};
const handleSetDateRangeOnSubmit = (dateRange: DateRange) => {
setDateRangeOnSubmit(dateRange);
// handleClose(); // close the modal
};
console.log("dateRangeOnChange", dateRangeOnChange);
console.log("dateRangeOnSubmit", dateRangeOnSubmit);
return (
<>
<Button variant="contained" onClick={handleClick}>
View Picker Model
</Button>
<PickerModal
onChange={(range: DateRange) => handleSetDateRangeOnChange(range)}
customProps={{
onSubmit: (range: DateRange) => handleSetDateRangeOnSubmit(range),
onCloseCallback: handleClose,
}}
modalProps={{
open,
anchorEl,
onClose: handleClose,
slotProps: {
paper: {
sx: {
borderRadius: "16px",
boxShadow: "rgba(0, 0, 0, 0.21) 0px 0px 4px",
},
},
},
anchorOrigin: {
vertical: "bottom",
horizontal: "left",
},
}}
/>
</>
);
}
import { useState } from "react";
import { PickerBase } from "mui-daterange-picker-plus/dist";
import type { DateRange } from "mui-daterange-picker-plus/dist";
export default function YourComponent() {
// state + handlers for the DateRange Value
const [dateRangeOnChange, setDateRangeOnChange] = useState<DateRange>({});
const handleSetDateRangeOnChange = (dateRange: DateRange) => {
setDateRangeOnChange(dateRange);
};
console.log("dateRangeOnChange", dateRangeOnChange);
return (
<PickerBase
onChange={(range: DateRange) => handleSetDateRangeOnChange(range)}
/>
);
}
import { useState } from "react";
import Button from "@mui/material/Button";
import { PickerModal } from "mui-daterange-picker-plus/dist";
import type { DateRange } from "mui-daterange-picker-plus/dist";
import ArrowCircleRightIcon from "@mui/icons-material/ArrowCircleRight";
import ArrowCircleDownIcon from "@mui/icons-material/ArrowCircleDown";
export default function YourComponent() {
// state + handlers for the Modal
const [anchorEl, setAnchorEl] = useState<HTMLButtonElement | null>(null);
const handleClick = (event: React.MouseEvent<HTMLButtonElement>) => {
setAnchorEl(event.currentTarget);
};
const handleClose = () => {
setAnchorEl(null);
};
const open = Boolean(anchorEl);
// state + handlers for the DateRange Value
const [dateRangeOnChange, setDateRangeOnChange] = useState<DateRange>({});
const [dateRangeOnSubmit, setDateRangeOnSubmit] = useState<DateRange>({});
const handleSetDateRangeOnChange = (dateRange: DateRange) => {
setDateRangeOnChange(dateRange);
handleSetDateRangeOnSubmit({});
};
const handleSetDateRangeOnSubmit = (dateRange: DateRange) => {
setDateRangeOnSubmit(dateRange);
// handleClose(); // close the modal
};
console.log("dateRangeOnChange", dateRangeOnChange);
console.log("dateRangeOnSubmit", dateRangeOnSubmit);
return (
<>
<Button variant="contained" onClick={handleClick}>
View Picker Model
</Button>
<PickerModal
hideOutsideMonthDays={false}
initialDateRange={{
startDate: new Date(),
endDate: new Date("2024-12-31"),
}}
minDate={new Date("2023-08-02")}
maxDate={new Date("2025-02-04")}
onChange={(range: DateRange) => handleSetDateRangeOnChange(range)}
customProps={{
onSubmit: (range: DateRange) => handleSetDateRangeOnSubmit(range),
onCloseCallback: handleClose,
RangeSeparatorIcons: {
xs: ArrowCircleDownIcon,
md: ArrowCircleRightIcon,
},
}}
modalProps={{
open,
anchorEl,
onClose: handleClose,
slotProps: {
paper: {
sx: {
borderRadius: "16px",
boxShadow: "rgba(0, 0, 0, 0.21) 0px 0px 4px",
},
},
},
anchorOrigin: {
vertical: "bottom",
horizontal: "left",
},
}}
/>
</>
);
}
import { useState } from "react";
import { PickerBase } from "mui-daterange-picker-plus/dist";
import type { DateRange } from "mui-daterange-picker-plus/dist";
export default function YourComponent() {
// state + handlers for the DateRange Value
const [dateRangeOnChange, setDateRangeOnChange] = useState<DateRange>({});
const handleSetDateRangeOnChange = (dateRange: DateRange) => {
setDateRangeOnChange(dateRange);
};
console.log("dateRangeOnChange", dateRangeOnChange);
return (
<PickerBase
hideOutsideMonthDays={false}
initialDateRange={{
startDate: new Date("2023-09-15"),
endDate: new Date("2024-12-31"),
}}
minDate={new Date("2023-08-02")}
maxDate={new Date("2025-02-04")}
onChange={(range: DateRange) => handleSetDateRangeOnChange(range)}
/>
);
}
Prop | Type | Default | Description |
---|---|---|---|
initialDateRange | DateRange | - | Initial date range for the picker. |
definedRanges | DefinedRange[] | - | Predefined date ranges for quick selection. |
minDate | Date | string | startOfYear(addYears( new Date(), -10)) | Minimum selectable date. |
maxDate | Date | string | endOfYear(addYears( new Date(), 10)) | Maximum selectable date. |
onChange | (dateRange: DateRange) => void | - | Callback function triggered on date range change. |
locale | Locale | - | Locale for date formatting. |
labels | Labels | - | Customize labels used in UI (Apply, Cancel, Start Date, End Date etc.) |
hideDefaultRanges | boolean | false | Option to hide default predefined ranges. |
hideOutsideMonthDays | boolean | true | Option to hide days outside the current month. |
Make sure to provide
initialDateRange
within the min and max date.
Prop | Type | Default | Description |
---|---|---|---|
onSubmit | (dateRange: DateRange) => void | - | Callback function triggered on date range submission. |
onCloseCallback | () => void | - | Callback function triggered on popover close. |
RangeSeparatorIcons | RangeSeparatorIconsProps | - | Icons for the range separator in different sizes. |
hideActionsButtons | boolean | false | Option to hide action buttons. |
import { PopoverProps } from "@mui/material/Popover";
import { PickerProps, ModalCustomProps } from "./utils";
type PickerModalProps = PickerProps & {
modalProps: PopoverProps;
customProps: ModalCustomProps;
};
type PickerBaseProps = PickerProps;
In the above examples, the
PickerBase
has includedPickerBaseProps
props. Same as that,PickerModal
has includedPickerModalProps
props.
The PickerProps
, ModalCustomProps
types are utility types and you can refer them as per your requirement. ( With or Without Modal)
In the below section, you can find the details of the utility types.
The PopoverProps
is a Material-UI Popover component props. You can refer to the Material-UI Popover API for more details.
import { ElementType } from "react";
import { SvgIconProps } from "@mui/material";
import { Locale } from "date-fns";
type DateRange = {
startDate?: Date;
endDate?: Date;
};
type DefinedRange = {
startDate: Date;
endDate: Date;
label: string;
};
type RangeSeparatorIconsProps = {
xs?: ElementType<SvgIconProps>;
md?: ElementType<SvgIconProps>;
};
type PickerProps = {
initialDateRange?: DateRange;
definedRanges?: DefinedRange[];
minDate?: Date | string;
maxDate?: Date | string;
locale?: Locale;
onChange?: (dateRange: DateRange) => void;
hideDefaultRanges?: boolean;
hideOutsideMonthDays?: boolean;
};
type ModalCustomProps = {
onSubmit?: (dateRange: DateRange) => void;
onCloseCallback?: () => void;
RangeSeparatorIcons?: RangeSeparatorIconsProps;
hideActionButtons?: boolean;
};
You can use these types as per your requirement.
This project is licensed under the MIT License.
1.0.8
FAQs
A modern Typescript/JavaScript library for simplifying date-range related operations and enhancing date-picker components in web applications. It provides a range of utilities, including date formatting, parsing, and a fully customizable date range picker
The npm package mui-daterange-picker-plus receives a total of 918 weekly downloads. As such, mui-daterange-picker-plus popularity was classified as not popular.
We found that mui-daterange-picker-plus demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.