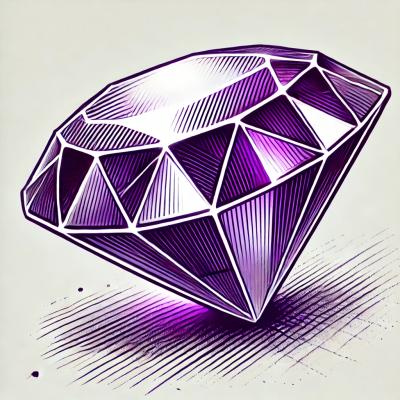
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
multicast-dns
Advanced tools
The multicast-dns npm package allows for low-level network discovery and communication using multicast DNS, which is often used in zero-configuration networking to resolve hostnames to IP addresses within small networks that do not include a local name server.
Discover services on the local network
This code sets up a multicast DNS instance to listen for responses to queries on the local network. It then sends out a query for the A (address) record for 'brunhilde.local'.
const mdns = require('multicast-dns')();
mdns.on('response', function(response) {
console.log('Got a response to an mDNS query:', response)
});
mdns.query({
questions:[{
name: 'brunhilde.local',
type: 'A'
}]
});
Advertise services on the local network
This code listens for mDNS queries and responds with the IP address '192.168.1.5' when a query for 'brunhilde.local' is received.
const mdns = require('multicast-dns')();
mdns.on('query', function(query) {
if (query.questions[0] && query.questions[0].name === 'brunhilde.local') {
mdns.respond({
answers: [{
name: 'brunhilde.local',
type: 'A',
ttl: 300,
data: '192.168.1.5'
}]
})
}
});
Bonjour is a higher-level mDNS package that provides an easy-to-use API for discovering and publishing network services. It builds on top of multicast-dns and abstracts some of the complexities involved in handling the mDNS protocol.
The mdns package is a more comprehensive solution for mDNS/DNS-SD service discovery. It is built on top of Apple's mDNSResponder, which is the same underlying technology used by Bonjour, Apple's implementation of zero-configuration networking. It provides a rich set of features but is not as lightweight as multicast-dns.
node-dns-sd is another package for service discovery using multicast DNS. It is similar to multicast-dns but focuses on the DNS Service Discovery (DNS-SD) protocol, which is built on top of mDNS and provides a standard way to discover services on a local network.
Low level multicast-dns implementation in pure javascript
npm install multicast-dns
var mdns = require('multicast-dns')()
mdns.on('response', function(response) {
console.log('got a response packet:', response)
})
mdns.on('query', function(query) {
console.log('got a query packet:', query)
})
// lets query for an A record for 'brunhilde.local'
mdns.query({
questions:[{
name: 'brunhilde.local',
type: 'A'
}]
})
Running the above (change brunhilde.local
to your-own-hostname.local
) will print an echo of the query packet first
got a query packet: { type: 'query',
qdcount: 1,
ancount: 0,
nscount: 0,
arcount: 0,
questions: [ { name: 'brunhilde.local', type: 'A', class: 1 } ],
answers: [],
authorities: [],
additionals: [] }
And then a response packet
got a response packet: { type: 'response',
qdcount: 0,
ancount: 1,
nscount: 0,
arcount: 2,
questions: [],
answers:
[ { name: 'brunhilde.local',
type: 'A',
class: 1,
ttl: 120,
flush: true,
data: '192.168.1.5' } ],
authorities: [],
additionals:
[ { name: 'brunhilde.local',
type: 'A',
class: 1,
ttl: 120,
flush: true,
data: '192.168.1.5' },
{ name: 'brunhilde.local',
type: 'AAAA',
class: 1,
ttl: 120,
flush: true,
data: 'fe80::5ef9:38ff:fe8c:ceaa' } ] }
npm install -g multicast-dns
multicast-dns brunhilde.local
> 192.168.1.1
A packet has the following format
{
questions: [{
name:'brunhilde.local',
type:'A'
}],
answers: [{
name:'brunhilde.local',
type:'A',
ttl:seconds,
data:(record type specific data)
}],
additionals: [
(same format as answers)
],
authorities: [
(same format as answers)
]
}
Currently data from SRV
, A
, PTR
, TXT
, AAAA
and HINFO
records is passed
mdns = multicastdns([options])
Creates a new mdns
instance. Options can contain the following
{
multicast: true // use udp multicasting
interface: '192.168.0.2' // explicitly specify a network interface. defaults to all
port: 5353, // set the udp port
ip: '224.0.0.251', // set the udp ip
ttl: 255, // set the multicast ttl
loopback: true, // receive your own packets
reuseAddr: true // set the reuseAddr option when creating the socket (requires node >=0.11.13)
}
mdns.on('query', (packet, rinfo))
Emitted when a query packet is received.
mdns.on('query', function(query) {
if (query.questions[0] && query.questions[0].name === 'brunhilde.local') {
mdns.respond(someResponse) // see below
}
})
mdns.on('response', (packet, rinfo))
Emitted when a response packet is received.
The response might not be a response to a query you send as this is the result of someone multicasting a response.
mdns.query(packet, [cb])
Send a dns query. The callback will be called when the packet was sent.
The following shorthands are equivalent
mdns.query('brunhilde.local', 'A')
mdns.query([{name:'brunhilde.local', type:'A'}])
mdns.query({
questions: [{name:'brunhilde.local', type:'A'}]
})
mdns.respond(packet, [cb])
Send a dns response. The callback will be called when the packet was sent.
// reply with a SRV and a A record as an answer
mdns.respond({
answers: [{
name: 'my-service',
type: 'SRV',
data: {
port:9999,
weigth: 0,
priority: 10,
target: 'my-service.example.com'
}
}, {
name: 'brunhilde.local',
type: 'A',
ttl: 300,
data: '192.168.1.5'
}]
})
The following shorthands are equivalent
mdns.respond([{name:'brunhilde.local', type:'A', data:'192.158.1.5'}])
mdns.respond({
answers: [{name:'brunhilde.local', type:'A', data:'192.158.1.5'}]
})
mdns.destroy()
Destroy the mdns instance. Closes the udp socket.
To start hacking on this module you can use this example to get started
git clone git://github.com/mafintosh/multicast-dns.git
npm install
node example.js
node cli.js $(hostname).local
MIT
FAQs
Low level multicast-dns implementation in pure javascript
We found that multicast-dns demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.