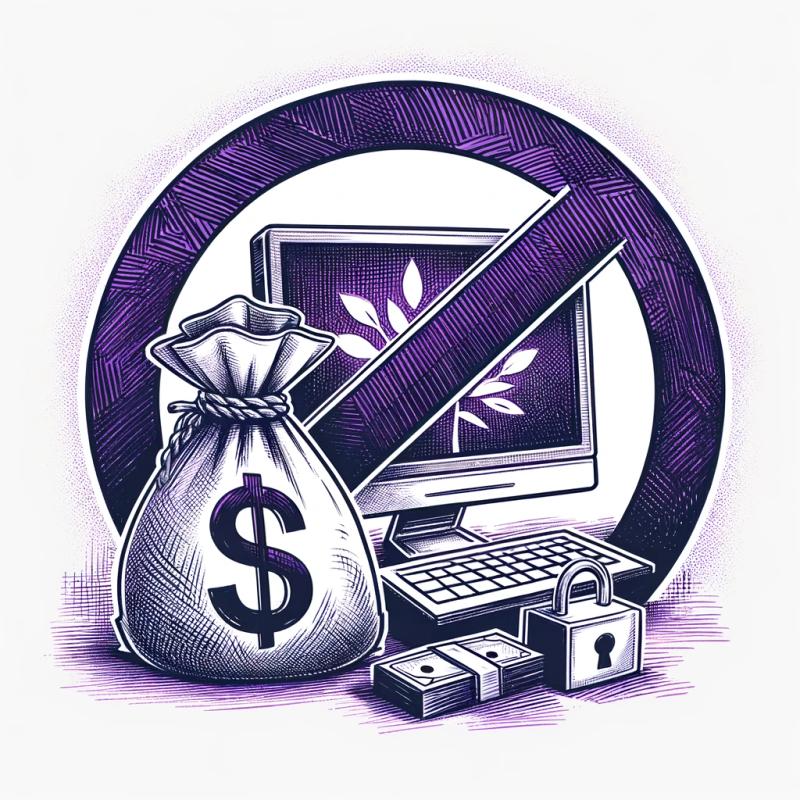
Security News
The Push to Ban Ransom Payments Is Gaining Momentum
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
mysql
Advanced tools
Readme
A pure node.js JavaScript Client implementing the MySQL protocol.
If you like this module, check out and spread the word about our service transloadit.com. We provide file uploading and encoding functionality to other applications, and have performed billions of queries with this module so far.
npm install mysql
Important: If you are upgrading from 0.9.1 or below, there have been backwards incompatible changes in the API. Please read the upgrading guide.
var mysql = require('mysql');
var TEST_DATABASE = 'nodejs_mysql_test';
var TEST_TABLE = 'test';
var client = mysql.createClient({
user: 'root',
password: 'root',
});
client.query('CREATE DATABASE '+TEST_DATABASE, function(err) {
if (err && err.number != mysql.ERROR_DB_CREATE_EXISTS) {
throw err;
}
});
// If no callback is provided, any errors will be emitted as `'error'`
// events by the client
client.query('USE '+TEST_DATABASE);
client.query(
'CREATE TEMPORARY TABLE '+TEST_TABLE+
'(id INT(11) AUTO_INCREMENT, '+
'title VARCHAR(255), '+
'text TEXT, '+
'created DATETIME, '+
'PRIMARY KEY (id))'
);
client.query(
'INSERT INTO '+TEST_TABLE+' '+
'SET title = ?, text = ?, created = ?',
['super cool', 'this is a nice text', '2010-08-16 10:00:23']
);
var query = client.query(
'INSERT INTO '+TEST_TABLE+' '+
'SET title = ?, text = ?, created = ?',
['another entry', 'because 2 entries make a better test', '2010-08-16 12:42:15']
);
client.query(
'SELECT * FROM '+TEST_TABLE,
function selectCb(err, results, fields) {
if (err) {
throw err;
}
console.log(results);
console.log(fields);
client.end();
}
);
Creates a new client instance. Any client property can be set using the
options
object.
The host to connect to.
The port to connect to.
The username to authenticate as.
The password to use.
The name of the database to connect to (optional).
Prints incoming and outgoing packets, useful for development / testing purposes.
Connection flags send to the server.
Sends a sql
query to the server. '?'
characters can be used as placeholders
for an array of params
that will be safely escaped before sending the final
query.
This method returns a Query
object which can be used to stream incoming row
data.
Warning: sql
statements with multiple queries separated by semicolons
are not supported yet.
Sends a ping command to the server.
Same as issuing a 'USE <database>'
query.
Returns some server statistics provided by MySql.
Allows to safely insert a list of params
into a sql
string using the
placeholder mechanism described above.
Escapes a single val
for use inside of a sql string.
Forces the client connection / socket to be destroyed right away.
Schedule a COM_QUIT packet for closing the connection. All currently queued queries will still execute before the graceful termination of the connection is attempted.
When the client has no callback / delegate for an error, it is emitted with this event instead.
Query objects are not meant to be invoked manually. To get a query object, use
the client.query
API.
Emitted when mysql returns an error packet for the query.
Emitted upon receiving a field packet from mysql.
Emitted upon receiving a row. An option for streaming the contents of the row itself will be made available soon.
Emitted once the query is finished. In case there is no result set, a result
parameter is provided which contains the information from the mysql OK packet.
This module is written entirely in JavaScript. There is no dependency on external C libraries such as libmysql. That means you don't have to compile this module at all.
client.query('INSERT INTO my_table SET title = ?', function(err, info) {
console.log(info.insertId);
});
client.query('UPDATE my_table SET title = ?', function(err, info) {
console.log(info.affectedRows);
});
At this point the module is ready to be tried out, but a lot of things are yet to be done:
A stop-gap solution to support multiple statements and transactions is available. Check it out here: http://github.com/bminer/node-mysql-queues
Click here for a full list of contributors.
This is a rather large project requiring a significant amount of my limited resources.
If your company could benefit from a well-engineered non-blocking mysql driver, and wants to support this project, I would greatly appriciate any sponsorship you may be able to provide. All sponsors will get lifetime display in this readme, priority support on problems, and votes on roadmap decisions. If you are interested, contact me at felix@debuggable.com for details.
Of course I'm also happy about code contributions. If you're interested in working on features, just get in touch so we can talk about API design and testing.
mysql.PACKAGE
(#104)client.user
to rootclient.escape()
throwing exceptions on objects. (Nick Payne)These releases were done before starting to maintain the above Changelog:
node-mysql is licensed under the MIT license.
FAQs
A node.js driver for mysql. It is written in JavaScript, does not require compiling, and is 100% MIT licensed.
We found that mysql demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
Application Security
New SEC disclosure rules aim to enforce timely cyber incident reporting, but fear of job loss and inadequate resources lead to significant underreporting.
Security News
The Python Software Foundation has secured a 5-year sponsorship from Fastly that supports PSF's activities and events, most notably the security and reliability of the Python Package Index (PyPI).