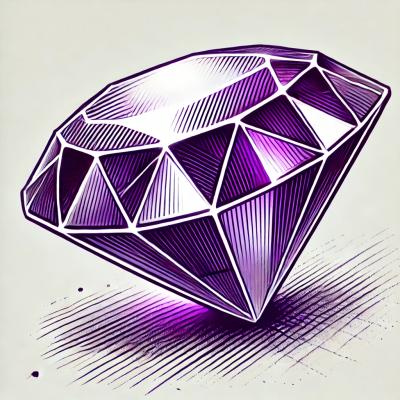
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
nativescript-barcodescanner
Advanced tools
formats
)formats
)Make sure you're using NativeScript 2.3.0+ (2.4.0+ is best for Android). Run npm install -g nativescript
if not.
From the command prompt go to your app's root folder and execute:
tns plugin add nativescript-barcodescanner
You've probably seen a permission popup like this before (this plugin will trigger one as well, automatically):
iOS 10+ requires not only this popup, but also a reason. In this case it's "We'd like to use the Camera ..".
You can provide your own reason for accessing the camera by adding something like this to app/App_Resources/ios/Info.plist
:
<key>NSCameraUsageDescription</key>
<string>My reason justifying fooling around with your camera</string>
To not crash your app in case you forgot to provide the reason this plugin adds an empty reason to the .plist
during build. This value gets overridden by anything you specified yourself. You're welcome.
Tip: during a scan you can use the volume up/down buttons to toggle the torch.
import {BarcodeScanner} from "nativescript-barcodescanner";
let barcodescanner = new BarcodeScanner();
barcodescanner.scan({
formats: "QR_CODE, EAN_13",
cancelLabel: "EXIT. Also, try the volume buttons!", // iOS only, default 'Close'
message: "Use the volume buttons for extra light", // Android only, default is 'Place a barcode inside the viewfinder rectangle to scan it.'
showFlipCameraButton: true, // default false
preferFrontCamera: false, // default false
showTorchButton: true, // default false
orientation: orientation, // Android only, default undefined (sensor-driven orientation), other options: portrait|landscape
openSettingsIfPermissionWasPreviouslyDenied: true // On iOS you can send the user to the settings app if access was previously denied
}).then((result) => {
// Note that this Promise is never invoked when a 'continuousScanCallback' function is provided
alert({
title: "Scan result",
message: "Format: " + result.format + ",\nValue: " + result.text,
okButtonText: "OK"
});
}, (errorMessage) => {
console.log("No scan. " + errorMessage);
}
);
var BarcodeScanner = require("nativescript-barcodescanner").BarcodeScanner;
var barcodescanner = new BarcodeScanner();
barcodescanner.scan({
formats: "QR_CODE,PDF_417", // Pass in of you want to restrict scanning to certain types
cancelLabel: "EXIT. Also, try the volume buttons!", // iOS only, default 'Close'
message: "Use the volume buttons for extra light", // Android only, default is 'Place a barcode inside the viewfinder rectangle to scan it.'
showFlipCameraButton: true, // default false
preferFrontCamera: false, // default false
showTorchButton: true, // default false
orientation: "landscape", // Android only, optionally lock the orientation to either "portrait" or "landscape"
openSettingsIfPermissionWasPreviouslyDenied: true // On iOS you can send the user to the settings app if access was previously denied
}).then(
function(result) {
console.log("Scan format: " + result.format);
console.log("Scan text: " + result.text);
},
function(error) {
console.log("No scan: " + error);
}
);
In this mode the scanner will continuously report scanned codes back to your code,
but it will only be dismissed if the user tells it to, or you call stop
programmatically.
The plugin handles duplicates for you so don't worry about checking those;
every result withing the same scan session is unique unless you set reportDuplicates
to true
.
Here's an example of scanning 3 unique QR codes and then stopping scanning programmatically. You'll notice that the Promise will no longer receive the result as there may be many results:
var count = 0;
barcodescanner.scan({
formats: "QR_CODE",
// this callback will be invoked for every unique scan in realtime!
continuousScanCallback: function (result) {
count++;
console.log(result.format + ": " + result.text + " (count: " + count + ")");
if (count == 3) {
barcodescanner.stop();
}
},
reportDuplicates: false // which is the default
}).then(
function() {
console.log("We're now reporting scan results in 'continuousScanCallback'");
},
function(error) {
console.log("No scan: " + error);
}
);
Note that the iOS implementation will always return true
at the moment,
on Android we actually check for a camera to be available.
var barcodescanner = require("nativescript-barcodescanner");
barcodescanner.available().then(
function(avail) {
console.log("Available? " + avail);
}
);
On Android 6+ you need to request permission to use the camera at runtime when targeting API level 23+.
Even if the uses-permission
tag for the Camera is present in AndroidManifest.xml
.
On iOS 10+ there's something similar going on.
Since version 1.5.0 you can let the plugin handle this for you (if need be a prompt will be shown to the user when the scanner launches), but if for some reason you want to handle permissions yourself you can use these functions.
barcodescanner.hasCameraPermission().then(
function(granted) {
// if this is 'false' you probably want to call 'requestCameraPermission' now
console.log("Has Camera Permission? " + result);
}
);
// if no permission was granted previously this wil open a user consent screen
barcodescanner.requestCameraPermission().then(
function() {
console.log("Camera permission requested");
}
);
nativescript-angular
When using Angular 2, it is best to inject dependencies into your classes. Here is an example of how you
can set up nativescript-barcodescanner
in an Angular 2 app with dependency injection.
//app.module.ts
import { NgModule, ValueProvider } from '@angular/core';
import { BarcodeScanner } from 'nativescript-barcodescanner';
//other imports
@NgModule({
//bootstrap, declarations, imports, etc.
providers: [
BarcodeScanner
]
})
export class AppModule {}
//my-component.ts
import { Component, Inject } from '@angular/core';
import { BarcodeScanner } from 'nativescript-barcodescanner';
//other imports
@Component({ ... })
export class MyComponent {
constructor(private barcodeScanner: BarcodeScanner) {
}
//use the barcodescanner wherever you need it. See general usage above.
scanBarcode() {
this.barcodeScanner.scan({ ... });
}
}
FAQs
Scan QR/barcodes with your NativeScript app.
The npm package nativescript-barcodescanner receives a total of 33 weekly downloads. As such, nativescript-barcodescanner popularity was classified as not popular.
We found that nativescript-barcodescanner demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.