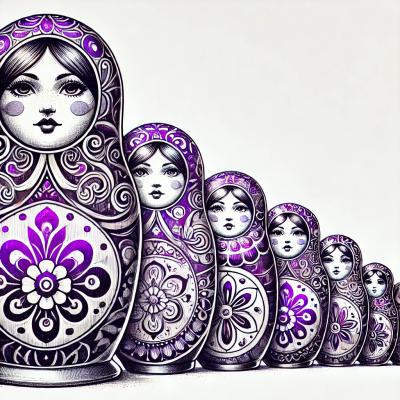
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
nestjs-prisma
Advanced tools
Add Prisma support to your NestJS application.
nest add nestjs-prisma
Example output
✔ Package installation in progress... ☕
Starting library setup...
? Do you like to generate your own PrismaService for customizations? No
? Do you like to Dockerize your application? Yes
? Which datasource provider do you want to use for `prisma init`? sqlite
✅️ Added prisma@2.26.0
✅️ Added @prisma/client@2.26.0
✅️ Added Prisma scripts [6]
✅️ Added Docker files
✅️ Add "prisma" directory to "excludes" in tsconfig.build.json
CREATE .dockerignore (42 bytes)
CREATE .env (192 bytes)
CREATE Dockerfile (455 bytes)
CREATE docker-compose.yml (474 bytes)
UPDATE package.json (2380 bytes)
UPDATE tsconfig.build.json (130 bytes)
✔ Packages installed successfully.
✔ Packages installed successfully.
✅️ Initialized Prisma - Datasource sqlite
PrismaService
and PrismaModule
provided by nestjs-prismaAnswer the first question with no if you like to use the provided PrismaService
and PrismaModule
. Add PrismaModule
to the imports
section in your AppModule
or other modules to gain access to PrismaService
.
import { Module } from '@nestjs/common';
import { PrismaModule } from 'nestjs-prisma';
@Module({
imports: [PrismaModule.forRoot()],
})
export class AppModule {}
PrismaModule
allows to be used globally and to pass options to the PrismaClient
.
import { Module } from '@nestjs/common';
import { PrismaModule } from 'nestjs-prisma';
@Module({
imports: [
PrismaModule.forRoot({
isGlobal: true,
prismaServiceOptions: {
prismaOptions: { log: ['info'] },
explicitConnect: true,
},
}),
],
})
export class AppModule {}
Additionally, PrismaModule
provides a forRootAsync
to pass options asynchronously. One option is to use a factory function:
import { Module } from '@nestjs/common';
import { PrismaModule } from 'nestjs-prisma';
@Module({
imports: [
PrismaModule.forRootAsync({
isGlobal: true,
useFactory: () => ({
prismaOptions: {
log: ['info', 'query'],
},
explicitConnect: false,
}),
}),
],
})
export class AppModule {}
You can inject dependencies such as ConfigModule
to load options from .env files.
import { Module } from '@nestjs/common';
import { ConfigModule, ConfigService } from '@nestjs/config';
import { PrismaModule } from 'nestjs-prisma';
@Module({
imports: [
ConfigModule.forRoot({
isGlobal: true,
}),
PrismaModule.forRootAsync({
isGlobal: true,
useFactory: async (configService: ConfigService) => {
return {
prismaOptions: {
log: [configService.get('log')],
datasources: {
db: {
url: configService.get('DATABASE_URL'),
},
},
},
explicitConnect: configService.get('explicit'),
};
},
inject: [ConfigService],
}),
],
})
export class AppModule {}
Alternatively, you can use a class instead of a factory:
import { Module } from '@nestjs/common';
import { PrismaModule } from 'nestjs-prisma';
@Module({
imports: [
ConfigModule.forRoot({
isGlobal: true,
}),
PrismaModule.forRootAsync({
isGlobal: true,
useClass: PrismaConfigService,
}),
],
})
export class AppModule {}
Create the PrismaConfigService
and extend it with the PrismaOptionsFactory
import { Injectable } from '@nestjs/common';
import { PrismaOptionsFactory, PrismaServiceOptions } from '.nestjs-prisma';
@Injectable()
export class PrismaConfigService implements PrismaOptionsFactory {
constructor() {
// TODO inject any other service here like the `ConfigService`
}
createPrismaOptions(): PrismaServiceOptions | Promise<PrismaServiceOptions> {
return {
prismaOptions: {
log: ['info', 'query'],
},
explicitConnect: true,
};
}
}
PrismaService
and PrismaModule
Answer the first question with yes if you like to generate your own PrismaService
and PrismaModule
for further customizations. Add PrismaModule
to the imports
section in your AppModule
or other modules to gain access to PrismaService
.
import { Module } from '@nestjs/common';
import { PrismaModule } from './prisma/prisma.module';
@Module({
imports: [PrismaModule],
})
export class AppModule {}
Note: It is safe to remove
nestjs-prisma
as dependency otherwise you have two import suggestions forPrismaService
andPrismaModule
.
nestjs-prisma
provides an PrismaClientExceptionFilter
to catch unhandled PrismaClientKnownRequestError and returning different status codes instead of 500 Internal server error
.
To use the filter you have the following two options.
main.ts
and pass the HttpAdapterHost
//src/main.ts
import { ValidationPipe } from '@nestjs/common';
import { HttpAdapterHost, NestFactory } from '@nestjs/core';
import { AppModule } from './app.module';
import { PrismaClientExceptionFilter } from 'nestjs-prisma';
async function bootstrap() {
const app = await NestFactory.create(AppModule);
const { httpAdapter } = app.get(HttpAdapterHost);
app.useGlobalFilters(new PrismaClientExceptionFilter(httpAdapter));
await app.listen(3000);
}
bootstrap();
APP_FILTER
token in any module//src/app.module.ts
import { Module } from '@nestjs/common';
import { APP_FILTER } from '@nestjs/core';
import { PrismaClientExceptionFilter } from 'nestjs-prisma';
@Module({
providers: [
{
provide: APP_FILTER,
useClass: PrismaClientExceptionFilter,
},
],
})
export class AppModule {}
All available flags:
Flag | Description | Type | Default |
---|---|---|---|
addPrismaService | Create a Prisma service extending the Prisma Client and module. | boolean | Prompted |
addDocker | Create a Dockerfile and docker-compose.yaml. | boolean | false |
dockerNodeImageVersion | Node version for the builder and runner image. | string | 14 |
name | The name for the Prisma service extending the Prisma Client and module. | string | Prisma |
prismaVersion | The Prisma version to be installed. | string | latest |
skipInstall | Skip installing dependency packages. | boolean | false |
skipPrismaInit | Skip initializing Prisma. | boolean | false |
You can pass additional flags to customize the schematic. For example, if you want to install a different version for Prisma use --prismaVersion
flag:
nest add nestjs-prisma --prismaVersion 2.20.1
If you want to skip installing dependencies use --skipInstall
flag:
nest add nestjs-prisma --skipInstall
Add Dockerfile
and docker-compose.yaml
, you can even use a different node
version (14-alpine
or 15
).
Currently uses to PostgreSQL as a default database in
docker-compose.yaml
.
nest add nestjs-prisma --addDocker --dockerNodeImageVersion 14-alpine
Install @angular-devkit/schematics-cli
to be able to use schematics
command
npm i -g @angular-devkit/schematics-cli
Now build the schematics and run the schematic.
npm run build:schematics
# or
npm run dev:schematics
# dry-run
schematics .:nest-add
# execute schematics
schematics .:nest-add --debug false
# or
schematics .:nest-add --dry-run false
Helpful article about Custom Angular Schematics which also applies to Nest.
FAQs
Library and schematics to add Prisma integration to a NestJS application
The npm package nestjs-prisma receives a total of 15,890 weekly downloads. As such, nestjs-prisma popularity was classified as popular.
We found that nestjs-prisma demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.