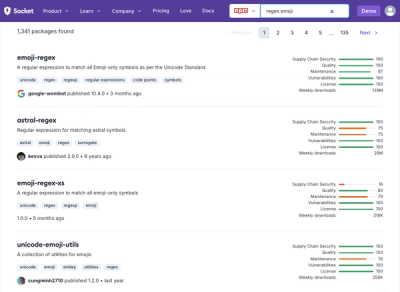
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
nestjs-schedule
Advanced tools
Distributed Schedule module for Nest.js based on the node-schedule package.
$ npm i --save nestjs-schedule
import { Module } from '@nestjs/common';
import { ScheduleModule } from 'nestjs-schedule';
@Module({
imports: [ScheduleModule.forRoot({
// Optional: Import external dependent modules if you need
imports: [MyLockerModule]
// Optional: Inject your global custom lock
useClass: MyScheduleLocker
})],
})
export class AppModule {}
import { Injectable, Logger } from '@nestjs/common';
import { Cron, Timeout, Interval } from 'nestjs-schedule';
@Injectable()
export class TasksService {
private readonly logger = new Logger(TasksService.name);
@Cron('45 * * * * *')
handleCron() {
this.logger.debug('Called when the current second is 45');
}
@Interval(5000)
handleInterval() {
this.logger.debug('Called every 5 seconds');
}
@Timeout(5000)
handleTimeout() {
this.logger.debug('Called after 5 seconds');
}
}
import { Injectable, Logger, OnModuleInit } from '@nestjs/common';
import { InjectSchedule, Schedule } from 'nestjs-schedule';
@Injectable()
export class TasksService implements OnModuleInit {
private readonly logger = new Logger(TasksService.name);
constructor(@InjectSchedule() private readonly schedule: Schedule) {}
execute() {
this.logger.debug('execute dynamic job');
}
onModuleInit() {
this.schedule.createIntervalJob(this.execute.bind(this), 3000, {
name: 'test_job',
});
this.schedule.deleteIntervalJob('test_job');
}
}
import { Locker } from 'nestjs-schedule';
import { Injectable } from '@nestjs/common';
@Injectable()
export class ScheduleLocker implements Locker {
release(jobName: string): any {}
async tryLock(jobName: string): Promise<boolean> {
// use redis lock or other methods
return true;
}
}
import { Injectable, Logger } from '@nestjs/common';
import { Cron, UseLocker } from '@nestjs-schedule';
import { ScheduleLocker } from './schedule-locker';
@Injectable()
export class TasksService {
private readonly logger = new Logger(TasksService.name);
@Cron('45 * * * * *')
// remove it if you want to use the lock which injected forRoot
@UseLocker(ScheduleLocker)
handleCron() {
this.logger.debug('Called when the current second is 45');
}
}
Import schedule module.
Dynamic create a timeout job.
field | type | required | description |
---|---|---|---|
methodRef | Function | true | job method |
timeout | number | true | milliseconds |
options | false | see decorators | |
locker | Locker/false | false | custom lock instance |
If the locker is configured as false, the default lock will be ignored
Dynamic create a interval job.
field | type | required | description |
---|---|---|---|
methodRef | Function | true | job method |
interval | number | true | milliseconds |
options | false | see decorators | |
locker | Locker/false | false | custom lock instance |
Dynamic create a cron job.
field | type | required | description |
---|---|---|---|
rule | Date string number CronObject CronObjLiteral | true | the cron rule |
methodRef | Function | true | job method |
options | false | see decorators | |
locker | Locker/false | false | custom lock instance |
Delete a timeout job
Delete a interval job
Delete a cron job
Get all timeout jobs
Get all interval jobs
Get all cron jobs
Schedule a cron job.
field | type | required | description |
---|---|---|---|
rule | Date string number CronObject CronObjLiteral | true | The cron rule |
rule.dayOfWeek | number | true | Timezone |
options.name | string | false | The unique job key.Distributed lock need it |
options.retries | number | false | the max retry count, default is -1 not retry |
options.retry | number | false | the retry interval, default is 5000 |
Schedule a interval job.
field | type | required | description |
---|---|---|---|
timeout | number | true | milliseconds |
options.retries | number | false | the max retry count, default is -1 not retry |
options.retry | number | false | the retry interval, default is 5000 |
options.immediate | boolean | false | executing job immediately |
Schedule a timeout job.
field | type | required | description |
---|---|---|---|
timeout | number | true | milliseconds |
options.retries | number | false | the max retry count, default is -1 not retry |
options.retry | number | false | the retry interval, default is 5000 |
options.immediate | boolean | false | executing job immediately |
Inject Schedule instance
Set a distributed locker for job.
NestCloud is MIT licensed.
FAQs
nestjs distributed timer schedule lib
The npm package nestjs-schedule receives a total of 714 weekly downloads. As such, nestjs-schedule popularity was classified as not popular.
We found that nestjs-schedule demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.