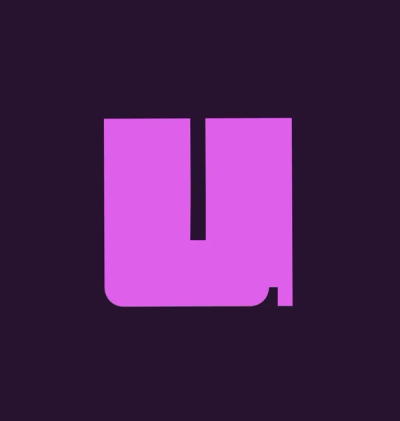
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
The netlify npm package provides a command-line interface (CLI) for interacting with Netlify's platform. It allows developers to deploy sites, manage DNS settings, configure build settings, and more, directly from the terminal.
Deploy a site
This feature allows you to deploy a site to Netlify. You need to provide your access token, site ID, and the directory path of your site.
const netlify = require('netlify');
async function deploySite() {
const client = new netlify.NetlifyAPI('YOUR_ACCESS_TOKEN');
const site = await client.deploy({
siteId: 'YOUR_SITE_ID',
dir: 'path/to/your/site'
});
console.log('Site deployed:', site);
}
deploySite();
Create a new site
This feature allows you to create a new site on Netlify. You need to provide your access token and the desired name for the new site.
const netlify = require('netlify');
async function createSite() {
const client = new netlify.NetlifyAPI('YOUR_ACCESS_TOKEN');
const site = await client.createSite({
body: {
name: 'my-new-site'
}
});
console.log('New site created:', site);
}
createSite();
List all sites
This feature allows you to list all the sites associated with your Netlify account. You need to provide your access token.
const netlify = require('netlify');
async function listSites() {
const client = new netlify.NetlifyAPI('YOUR_ACCESS_TOKEN');
const sites = await client.listSites();
console.log('All sites:', sites);
}
listSites();
The vercel npm package provides a CLI for interacting with Vercel's platform. It allows developers to deploy sites, manage projects, and configure settings. Vercel is similar to Netlify in that it offers serverless functions, continuous deployment, and a global CDN.
The firebase-tools npm package provides a CLI for interacting with Firebase services. It allows developers to deploy web apps, manage databases, and configure hosting settings. Firebase Hosting is similar to Netlify in that it offers static site hosting, serverless functions, and continuous deployment.
The aws-cli npm package provides a CLI for interacting with Amazon Web Services. It allows developers to manage S3 buckets, deploy Lambda functions, and configure CloudFront distributions. AWS offers a more extensive range of services compared to Netlify, but it requires more configuration and management.
A Netlify open-api client that works in the browser and Node.js.
const NetlifyAPI = require('netlify')
const client = new NetlifyAPI('1234myAccessToken')
const sites = await client.listSites()
client = new NetlifyAPI([accessToken], [opts])
Create a new instance of the Netlify API client with the provided accessToken
.
accessToken
is optional. Without it, you can't make authorized requests.
opts
includes:
{
userAgent: 'netlify/js-client',
scheme: 'https',
host: 'api.netlify.com',
pathPrefix: '/api/v1',
globalParams: {} // parameters you want available for every request
}
client.accessToken
A setter/getter that returns the accessToken
that the client is configured to use. You can set this after the class is instantiated, and all subsequent calls will use the newly set accessToken
.
client.basePath
A getter that returns the formatted base URL of the endpoint the client is configured to use.
The client is dynamically generated from the open-api definition file. Each method is is named after the operationId
name of each endpoint action. To see list of available operations see the open-api website.
Every open-api method has the following signature:
promise(response) = client.operationId([params], [opts])
Perform a call to the given endpoint corresponding with the operationId
. Returns promise that will resolve with the body of the response, or reject with an error with details about the request attached. Rejects if the status
> 400. Successful response objects have status
and statusText
properties on their prototype.
params
is an object that includes any of the required or optional endpoint parameters. params.body
should be an object which gets serialized to JSON automatically. If the endpoint accepts binary
, params.body
can be a Node.js readable stream.
// example params
{
any_param_needed,
paramsCanAlsoBeCamelCase,
body: {
an: 'arbitrary js object'
}
}
Optional opts
can include any property you want passed to node-fetch
. The headers
property is merged with some defaultHeaders
.
// example opts
{
headers: { // Default headers
'User-agent': 'netlify-js-client',
accept: 'application/json'
}
// any other properties for node-fetch
}
All methods are conveniently consumed with async/await:
async function getSomeData () {
// Calls may fail!
try {
const resposnse = await client.getSites()
return response
} catch (e) {
// handle error
}
}
If the request response includes json
in the contentType
header, fetch will deserialize the JSON body. Otherwise the text
of the response is returned.
Some methods have been added in addition to the open API methods that make certain actions simpler to perform.
promise(accessToken) = client.getAccessToken(ticket, [opts])
Pass in a ticket
and get back an accessToken
. Call this with the response from a client.createTicket({ client_id })
call. Automatically sets the accessToken
to this.accessToken
and returns accessToken
for the consumer to save for later.
Optional opts
include:
{
poll: 1000, // number of ms to wait between polling
timeout: 3.6e6 // number of ms to wait before timing out
}
See the authenticating docs for more context.
// example:
async function login () {
const ticket = await api.createTicket({
clientId: CLIENT_ID
})
// Open browser for authentication
await openBrowser(`https://app.netlify.com/authorize?response_type=ticket&ticket=${ticket.id}`)
const accessToken = await api.getAccessToken(ticket)
// API is also set up to use the returned access token as a side effect
return accessToken
}
promise(deploy) = client.deploy(siteId, buildDir, [opts])
Node.js Only: Pass in a siteId
, a buildDir
(the folder you want to deploy) and an options object to deploy the contents of that folder.
Sometimes need to write to a tmpDir
.
The following paths can be passed in the options:
tomlPath
(a netlify.toml
file that includes redirect rules for the deploy)functionsDir
(a folder with lambda functions to deploy)Optional opts
include:
{
functionsDir: null, // path to a folder of functions to deploy
tomlPath: null, // path to a netlify.toml file to include in the deploy (e.g. redirect support for manual deploys)
draft: false, // draft deploy or production deploy
message: undefined, // a short message to associate with the deploy
deployTimeout: 1.2e6, // 20 mins
parallelHash: 100, // number of parallel hashing calls
parallelUpload: 4, // number of files to upload in parallel
filter: filename => { /* return false to filter a file from the deploy */ },
tmpDir: tempy.directory(), // a temporary directory to zip loose files into
statusCb: statusObj => {
// a callback function to receive status events
/* statusObj: {
type: name-of-step
msg: msg to print
phase: [start, progress, stop]
} */
// See https://github.com/netlify/cli/blob/v2.0.0-beta.3/src/commands/deploy.js#L161-L195
// for an example of how this can be used.
}
}
FAQs
Netlify Node.js API client
The npm package netlify receives a total of 114,953 weekly downloads. As such, netlify popularity was classified as popular.
We found that netlify demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 17 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.