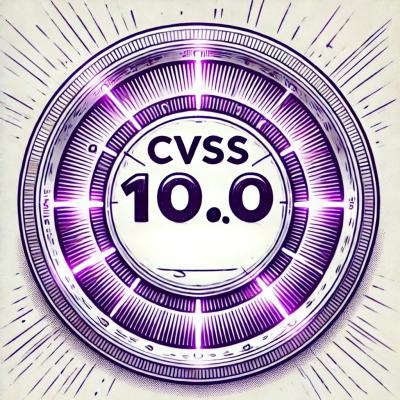
Security News
cURL Project and Go Security Teams Reject CVSS as Broken
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
neverthrow
Advanced tools
The neverthrow npm package provides a functional way to handle errors in TypeScript and JavaScript. It introduces the Result and Option types, which help in managing success and failure cases without using exceptions.
Result Type
The Result type is used to represent either a success (ok) or a failure (err). This example demonstrates a division function that returns a Result type, handling division by zero as an error.
const { ok, err, Result } = require('neverthrow');
function divide(a, b) {
if (b === 0) {
return err(new Error('Division by zero'));
}
return ok(a / b);
}
const result = divide(4, 2);
result.match({
ok: value => console.log('Result:', value),
err: error => console.error('Error:', error.message)
});
Option Type
The Option type is used to represent an optional value that may or may not be present. This example shows a function that looks up a user by ID and returns an Option type.
const { some, none, Option } = require('neverthrow');
function findUserById(id) {
const users = { 1: 'Alice', 2: 'Bob' };
return users[id] ? some(users[id]) : none();
}
const user = findUserById(1);
user.match({
some: value => console.log('User found:', value),
none: () => console.log('User not found')
});
Chaining Operations
Chaining operations with Result types allows for sequential error handling. This example demonstrates parsing a number and then dividing it, with each step returning a Result type.
const { ok, err, Result } = require('neverthrow');
function parseNumber(str) {
const num = Number(str);
return isNaN(num) ? err(new Error('Invalid number')) : ok(num);
}
function divide(a, b) {
if (b === 0) {
return err(new Error('Division by zero'));
}
return ok(a / b);
}
const result = parseNumber('4').andThen(num => divide(num, 2));
result.match({
ok: value => console.log('Result:', value),
err: error => console.error('Error:', error.message)
});
Folktale is a standard library for functional programming in JavaScript. It provides similar functionality to neverthrow with its Result and Maybe types, but also includes a broader range of functional programming utilities.
fp-ts is a library for functional programming in TypeScript. It offers a comprehensive set of tools for functional programming, including Result and Option types, similar to neverthrow, but with a more extensive ecosystem and integration with other functional programming concepts.
Purify is a functional programming library for TypeScript that provides Maybe and Either types, which are analogous to neverthrow's Option and Result types. It focuses on immutability and functional programming patterns.
Encode failure into your program.
This program contains a Result type that represents either success (Ok) or failure (Err).
This package works for both JS and TypeScript. However, the types that this package provides will allow you to get compile-time guarantees around error handling if you are using TypeScript.
Although the package is called neverthrow
, please don't take this literally. I am simply encouraging the developer to think a bit more about the ergonomics and usage of whatever software they are writing. Throw
ing and catching
is syntactic sugar for goto
statements - in other words; it makes reasoning about your programs harder.
With all that said, there are definitely good use cases for throwing in your program. But much less than you might think.
FAQs
Stop throwing errors, and instead return Results!
The npm package neverthrow receives a total of 620,637 weekly downloads. As such, neverthrow popularity was classified as popular.
We found that neverthrow demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
Security News
Biden's executive order pushes for AI-driven cybersecurity, software supply chain transparency, and stronger protections for federal and open source systems.