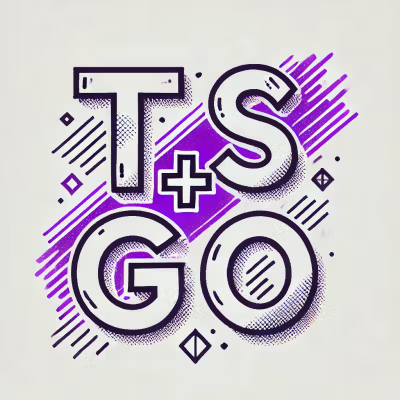
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
A production-grade error creation and serialization library designed for Typescript
A production-grade error creation library designed for Typescript. Useful for direct printing of errors to a client or for internal development / logs.
Error
with additional methods added on.The basic Javascript Error
type is extremely bare bones - you can only specify a message.
In a production-level application, I've experienced the following use-cases:
new-error
was built with these use-cases in mind.
$ npm i new-error --save
The error registry is the fastest way to define and create errors.
// This is a working example
import { ErrorRegistry } from 'new-error'
// Define high level errors
// Do *not* assign a Typescript type to the object
// or IDE autocompletion will not work!
const errors = {
INTERNAL_SERVER_ERROR: {
/**
* The class name of the generated error
*/
className: 'InternalServerError',
/**
* A user-friendly code to show to a client.
*/
code: 'ERR_INT_500',
/**
* (optional) Protocol-specific status code, such as an HTTP status code. Used as the
* default if a Low Level Error status code is not defined.
*/
statusCode: 500,
/**
* (optional) Log level string / number to associate with this error.
* Useful if you want to use your logging system to log the error but
* assign a different log level for it. Used as the default if a
* Low Level log level is not defined.
*/
logLevel: 'error',
/**
* (optional) Callback function to call when calling BaseError#convert().
*
* (baseError) => any type
*
* - If not defined, will return itself when convert() is called
* - If defined in HighLevelError, the HighLevelError definition takes priority
*/
onConvert: (err) => { return err },
/**
* (optional) Full description of the error. Used only when BaseError#newBareError() is called
* without the message parameter.
*
* sprintf() flags can be applied to customize it.
* @see https://www.npmjs.com/package/sprintf-js
*/
message: 'Internal server error'
}
}
// Define low-level errors
// Do *not* assign a Typescript type to the object
// or IDE autocompletion will not work!
const errorSubCodes = {
// 'type' of error
DATABASE_FAILURE: {
/**
* Full description of the error. sprintf() flags can be applied
* to customize it.
* @see https://www.npmjs.com/package/sprintf-js
*/
message: 'There was a database failure, SQL err code %s',
/**
* (optional) A user-friendly code to show to a client.
*/
subCode: 'DB_0001',
/**
* (optional) Protocol-specific status code, such as an HTTP status code.
*/
statusCode: 500,
/**
* (optional) Log level string / number to associate with this error.
* Useful if you want to use your logging system to log the error but
* assign a different log level for it.
*/
logLevel: 'error',
/**
* (optional) Callback function to call when calling BaseError#convert().
*
* (baseError) => any type
*
* - If not defined, will return itself when convert() is called
* - This definition takes priority if HighLevelError#onConvert is defined
*/
onConvert: (err) => { return err }
}
}
// Create the error registry by registering your errors and codes
// you will want to memoize this as you will be using the
// reference throughout your application
const errRegistry = new ErrorRegistry(errors, errorSubCodes)
// Create an instance of InternalServerError
// Typescript autocomplete should show the available definitions as you type the error names
// and type check will ensure that the values are valid
const err = errRegistry.newError('INTERNAL_SERVER_ERROR', 'DATABASE_FAILURE')
.withErrorId('err-1234')
.formatMessage('SQL_1234')
console.log(err.toJSON())
Produces:
(You can omit fields you do not need - see usage section below.)
{
errId: 'err-1234',
name: 'InternalServerError',
code: 'ERR_INT_500',
message: 'There was a database failure, SQL err code SQL_1234',
type: 'DATABASE_FAILURE',
subCode: 'DB_0001',
statusCode: 500,
meta: {},
stack: 'InternalServerError: There was a database failure, SQL err code %s\n' +
' at ErrorRegistry.newError (new-error/src/ErrorRegistry.ts:128:12)\n' +
' at Object.<anonymous> (new-error/src/test.ts:55:25)\n' +
' at Module._compile (internal/modules/cjs/loader.js:1158:30)\n' +
' at Module._compile (new-error/node_modules/source-map-support/source-map-support.js:541:25)\n' +
' at Module.m._compile (/private/var/folders/mx/b54hc2lj3fbfsndkv4xmz8d80000gn/T/ts-node-dev-hook-20649714243977457.js:57:25)\n' +
' at Module._extensions..js (internal/modules/cjs/loader.js:1178:10)\n' +
' at require.extensions.<computed> (/private/var/folders/mx/b54hc2lj3fbfsndkv4xmz8d80000gn/T/ts-node-dev-hook-20649714243977457.js:59:14)\n' +
' at Object.nodeDevHook [as .ts] (new-error/node_modules/ts-node-dev/lib/hook.js:61:7)\n' +
' at Module.load (internal/modules/cjs/loader.js:1002:32)\n' +
' at Function.Module._load (internal/modules/cjs/loader.js:901:14)'
}
generateHighLevelErrors(errorDefs, options: GenerateHighLevelErrorOpts)
If you find yourself doing the following pattern:
const errors = {
INTERNAL_SERVER_ERROR: {
className: 'InternalServerError', // pascal case'd property name
code: 'INTERNAL_SERVER_ERROR', // same as the property name
statusCode: 500
}
}
You can use the utility method to do it instead:
import { generateHighLevelErrors } from 'new-error'
const errors = generateHighLevelErrors({
INTERNAL_SERVER_ERROR: {
statusCode: 500
}
})
className
or code
is already defined, it will not overwrite itinterface GenerateHighLevelErrorOpts {
/**
* Disable to not generate the class name based on the property name if the className is not defined.
*/
disableGenerateClassName?: boolean
/**
* Disable to not generate the error code based on the property name if the code is not defined.
*/
disableGenerateCode?: boolean
}
generateLowLevelErrors(errorDefs, options: GenerateLowLevelErrorOpts)
If you find yourself doing the following pattern:
const errors = {
DATABASE_FAILURE: {
subCode: 'DATABASE_FAILURE', // same as the property name
message: 'Database failure'
}
}
You can use the utility method to do it instead:
import { generateLowLevelErrors } from 'new-error'
const errors = generateLowLevelErrors({
DATABASE_FAILURE: {
message: 'Database failure'
}
})
subCode
is already defined, it will not overwrite itinterface GenerateLowLevelErrorOpts {
/**
* Disable to not generate the error code based on the property name if the subCode is not defined.
*/
disableGenerateSubCode?: boolean
}
You can create concrete error classes by extending the BaseRegistryError
class, which
extends the BaseError
class.
The registry example can be also written as:
import { BaseRegistryError, LowLevelError } from 'new-error'
class InternalServerError extends BaseRegistryError {
constructor (errDef: LowLevelError) {
super({
code: 'ERR_INT_500',
statusCode: 500
}, errDef)
}
}
const err = new InternalServerError({
type: 'DATABASE_FAILURE',
message: 'There was a database failure, SQL err code %s',
subCode: 'DB_0001',
statusCode: 500,
logLevel: 'error'
})
console.log(err.formatMessage('SQL_1234').toJSON())
If you want a native-style Error
, you can use BaseError
.
The registry example can be written as:
import { BaseError } from 'new-error'
class InternalServerError extends BaseError {}
const err = new InternalServerError('There was a database failure, SQL err code %s')
// calling these methods are optional
.withErrorType('DATABASE_FAILURE')
.withErrorCode('ERR_INT_500')
.withErrorSubCode('DB_0001')
.withStatusCode(500)
.withLogLevel('error')
console.log(err.formatMessage('SQL_1234').toJSON())
import express from 'express'
import { ErrorRegistry, BaseError } from 'new-error'
const app = express()
const port = 3000
const errors = {
INTERNAL_SERVER_ERROR: {
className: 'InternalServerError',
code: 'ERR_INT_500',
statusCode: 500
}
}
const errorSubCodes = {
DATABASE_FAILURE: {
message: 'There was a database failure.',
subCode: 'DB_0001',
statusCode: 500
}
}
const errRegistry = new ErrorRegistry(errors, errorSubCodes)
// middleware definition
app.get('/', async (req, res, next) => {
try {
// simulate a failure
throw new Error('SQL issue')
} catch (e) {
const err = errRegistry.newError('INTERNAL_SERVER_ERROR', 'DATABASE_FAILURE')
err.causedBy(err)
// errors must be passed to next()
// to be caught when using an async middleware
return next(err)
}
})
// catch errors
app.use((err, req, res, next) => {
// error was sent from middleware
if (err) {
// check if the error is a generated one
if (err instanceof BaseError) {
// generate an error id
// you'll want to use a library like 'nanoid' instead
// this is just an example
err.withErrorId(Math.random().toString(36).slice(2))
// log the error
// the "null, 2" options formats the error into a readable structure
console.error(JSON.stringify(err.toJSON(), null, 2))
// get the status code, if the status code is not defined, default to 500
res.status(err.getStatusCode() ?? 500)
// spit out the error to the client
return res.json({
err: err.toJSONSafe()
})
}
// You'll need to modify code below to best fit your use-case
// err.message could potentially expose system internals
return res.json({
err: {
message: err.message
}
})
}
// no error, proceed
next()
})
app.listen(port, () => console.log(`Example app listening at http://localhost:${port}`))
If you visit http://localhost:3000
, you'll get a 500 status code, and the following response:
{"err": {"errId": "xd0v1szkziq", code":"ERR_INT_500","subCode":"DB_0001","statusCode":500,"meta":{}}}
You might want to use a different log level when logging common errors, such as validation errors.
import { ErrorRegistry } from 'new-error'
const errors = {
VALIDATION_ERROR: {
className: 'ValidationError',
code: 'VALIDATION_ERROR',
statusCode: 400,
// probably don't want to log every validation error
// in production since these errors tend to happen frequently
// and would pollute the logs
logLevel: 'debug'
}
}
const errorSubCodes = {
MISSING_FORM_FIELDS: {
message: 'Form submission data is missing fields',
subCode: 'MISSING_FORM_FIELDS',
statusCode: 400
}
}
const errRegistry = new ErrorRegistry(errors, errorSubCodes)
// some part of the application throws the error
const err = errRegistry.newError('VALIDATION_ERROR', 'MISSING_FORM_FIELDS')
// another part of the application catches the error
if (err.getLogLevel() === 'debug') {
console.debug(JSON.stringify(err.toJSON(), null, 2))
} else {
console.error(JSON.stringify(err.toJSON(), null, 2))
}
The ErrorRegistry
is responsible for the registration and creation of errors.
new ErrorRegistry(highLvErrors, lowLvErrors, config = {})
interface IErrorRegistryConfig {
/**
* Options when creating a new BaseError
*/
baseErrorConfig?: IBaseErrorConfig
/**
* Handler to modify the created error when newError / newBareError is called
*/
onCreateError?: (err: BaseRegistryError) => void
}
const errRegistry = new ErrorRegistry(errors, errorSubCodes, {
// Config for all BaseErrors created from the registry
baseErrorConfig: {
// Remove the `meta` field if there is no data present for `toJSON` / `toJSONSafe`
omitEmptyMetadata: true
}
})
ErrorRegistry#withContext(context: IErrorRegistryContextConfig)
You can create a child registry that adds context for all new errors created. This is useful if your body of code throws multiple errors and you want to include the same metadata for each one without repeating yourself.
export interface IErrorRegistryContextConfig {
/**
* Metadata to include for each new error created by the registry
*/
metadata?: Record<any, string>
/**
* Safe metadata to include for each new error created by the registry
*/
safeMetadata?: Record<any, string>
}
const childRegistry = errRegistry.withContext({
metadata: {
contextA: 'context-a'
},
safeMetadata: {
contextB: 'context-b'
}
})
const err = childRegistry.newError('INTERNAL_SERVER_ERROR', 'DATABASE_FAILURE')
If we do err.toJSON()
, we should get the following output:
{
name: 'InternalServerError',
code: 'INT_ERR',
message: 'There is an issue with the database',
type: 'DATABASE_FAILURE',
subCode: 'DB_ERR',
statusCode: 500,
// err.toJSONSafe() would exclude contextA
meta: { contextA: 'context-a', contextB: 'context-b' },
stack: '...'
}
We can also append data:
const err = childRegistry.newError('INTERNAL_SERVER_ERROR', 'DATABASE_FAILURE')
.withMetadata({
moreMeta: 'data'
})
If we do err.toJSON()
, we should get the following output:
{
name: 'InternalServerError',
code: 'INT_ERR',
message: 'There is an issue with the database',
type: 'DATABASE_FAILURE',
subCode: 'DB_ERR',
statusCode: 500,
// err.toJSONSafe() would exclude contextA and moreMeta
meta: { contextA: 'context-a', contextB: 'context-b', moreMeta: 'data' },
stack: '...'
}
Errors generated by the registry extends BaseError
.
Method: ErrorRegistry#newError(highLevelErrorName, LowLevelErrorName)
This is the method you should generally use as you are forced to use your well-defined high and low level error definitions. This allows for consistency in how errors are defined and thrown.
// Creates an InternalServerError error with a DATABASE_FAILURE code and corresponding
// message and status code
const err = errRegistry.newError('INTERNAL_SERVER_ERROR', 'DATABASE_FAILURE')
Method: ErrorRegistry#newBareError(highLevelErrorName, [message])
This method does not include a low level error code, and allows direct specification of an error message.
// Creates an InternalServerError error with a custom message
const err = errRegistry.newBareError('INTERNAL_SERVER_ERROR', 'An internal server error has occured.')
const errors = {
AUTH_REQUIRED: {
className: 'AuthRequired',
code: 'AUTH_REQ',
message: 'Auth required'
}
}
// Creates an AuthRequired error with a the 'Auth Required' message
const err = errRegistry.newBareError('AUTH_REQUIRED')
The error will use the code as the default.
const errors = {
DB_ERROR: {
className: 'DatabaseError',
code: 'DB_ERR'
}
}
// Creates an AuthRequired error with 'DB_ERR' as the message
const err = errRegistry.newBareError('DB_ERROR')
If you want all errors created from the registry to have defined properties, you can use the onCreateError
config option to modify the created error.
For example, if you want to create an error id for each new error:
const errRegistry = new ErrorRegistry(errors, errorSubCodes, {
onCreateError: (err) => {
err.withErrorId('test-id')
}
})
// the err should have 'test-id' set for the error id
const err = errRegistry.newError('INTERNAL_SERVER_ERROR', 'DATABASE_FAILURE')
instanceOf
/ comparisonsMethod: ErrorRegistry#instanceOf(classInstance, highLevelErrorName)
Performs an instanceof
operation against a custom error.
// creates an InternalServerError error instance
const err = errRegistry.newError('INTERNAL_SERVER_ERROR', 'DATABASE_FAILURE')
if (errRegistry.instanceOf(err, 'INTERNAL_SERVER_ERROR')) {
// resolves to true since err is an InternalServerError instance
}
instanceof
You can also check if the error is custom-built using this check:
import { BaseError } from 'new-error'
function handleError(err) {
if (err instanceof BaseError) {
// err is a custom error
}
}
Except for the getter and serialization methods, all other methods are chainable.
Generated errors extend the BaseError
class, which extends Error
.
new BaseError(message: string, config: IBaseErrorConfig: IBaseErrorConfig = {})
message
: The error message you would use in new Error(message)
interface IBaseErrorConfig {
/**
* A list of fields to always omit when calling toJSON
*/
toJSONFieldsToOmit?: string[]
/**
* A list of fields to always omit when calling toJSONSafe
*/
toJSONSafeFieldsToOmit?: string[]
/**
* If the metadata has no data defined, remove the `meta` property on `toJSON` / `toJSONSafe`.
*/
omitEmptyMetadata?: boolean
/**
* A function to run against the computed data when calling `toJSON`. This is called prior
* to field omission. If defined, must return the data back.
*/
onPreToJSONData?: (data: Partial<SerializedError>) => Partial<SerializedError>
/**
* A function to run against the computed safe data when calling `toJSONSafe`. This is called
* prior to field omission. If defined, must return the data back.
*/
onPreToJSONSafeData?: (data: Partial<SerializedErrorSafe>) => Partial<SerializedErrorSafe>
/**
* A callback function to call when calling BaseError#convert(). This allows for user-defined conversion
* of the BaseError into some other type (such as a Apollo GraphQL error type).
*
* (baseError) => any type
*/
onConvert?: <E extends BaseError = BaseError>(err: E) => any
/**
* If defined, will append the `.message` value when calling causedBy() after the main error message.
* Useful for frameworks like Jest where it will not print the caused by data.
* To define the format of the appended message, use '%s' for the message value.
*
* Ex: ", caused by: %s"
*/
appendWithErrorMessageFormat?: string
}
The following getters are included with the standard Error
properties and methods:
BaseError#getErrorId()
BaseError#getRequestId()
BaseError#getErrorName()
BaseError#getCode()
BaseError#getErrorType()
BaseError#getSubCode()
BaseError#getStatusCode()
BaseError#getCausedBy()
BaseError#getMetadata()
BaseError#getSafeMetadata()
BaseError#getLogLevel()
BaseError#getConfig()
If you use the registry, you should not need to us these setters as the registry sets the values already.
BaseError#withErrorType(type: string): this
BaseError#withErrorCode(code: string | number): this
BaseError#withErrorSubCode(code: string | number): this
BaseError#withLogLevel(level: string | number): this
BaseError#setConfig(config: IBaseErrorConfig): void
BaseError#setOnConvert(<E extends BaseError = BaseError>(err: E) => any): void
static BaseError#fromJSON(data: object, options?: object): BaseError
BaseError#convert<E = BaseError | any>() : E
BaseError#hasOnConvertDefined(): boolean
Method: BaseError#withErrorId(errId: string)
Attaches an id to the error. Useful if you want to display an error id to a client / end-user and want to cross-reference that id in an internal logging system for easier troubleshooting.
For example, you might want to use nanoid
to generate ids for errors.
import { nanoid } from 'nanoid'
err.withErrorId(nanoid())
// In your logging system, log the error, which will include the error id
logger.error(err.toJSON())
// expose the error to the client via err.toJSONSafe() or err.getErrorId(), which
// will also include the error id - an end-user can reference this id to
// support for troubleshooting
Method: BaseError#withRequestId(reqId: string)
Attaches request id to the error. Useful if you want to display the request id to a client / end-user and want to cross-reference that id in an internal logging system for easier troubleshooting.
For example, you might want to use nanoid
to generate ids.
import { nanoid } from 'nanoid'
err.withRequestId(nanoid())
// In your logging system, log the error, which will include the error id
logger.error(err.toJSON())
// expose the error to the client via err.toJSONSafe() or err.getRequestId(), which
// will also include the error id - an end-user can reference this id to
// support for troubleshooting
Method: BaseError#causedBy(err: any)
You can attach another error to the error.
const externalError = new Error('Some thrown error')
err.causedBy(externalError)
If the config option appendWithErrorMessageFormat
is defined, and the error sent into causedBy
contains a message
property, then the caused by error message will be appended to the main error message.
Useful if you find yourself applying this pattern to expose the attached error message:
const thrownErrorFromApp = new Error('Duplicate key error')
const err = new BaseError('Internal server error: %s');
err.causedBy(thrownErrorFromApp)
err.formatMessage(thrownErrorFromApp.message);
This is also useful for test frameworks like jest
where it will only print out the main error message
and not any properties attached to the error.
// only enable for testing envs
const IS_TEST_ENV = process.env.NODE_ENV === 'test';
const err = new BaseError('Internal server error', {
// %s is the attached error message
appendWithErrorMessageFormat: IS_TEST_ENV ? ': %s' : null
})
err.causedBy(new Error('Duplicate key'))
// prints out "Internal server error: Duplicate key"
console.log(err.message)
// formatted messages also work with this
const IS_TEST_ENV = process.env.NODE_ENV === 'test';
const err = new BaseError('Internal server error: %s', {
appendWithErrorMessageFormat: IS_TEST_ENV ? '===> %s' : null
})
// formatMessage / causedBy can be called in any order
err.formatMessage('Hello')
err.causedBy(new Error('Duplicate key'))
// prints out "Internal server error: Hello ===> Duplicate key"
console.log(err.message)
It is not recommended that appendWithErrorMessageFormat
is defined in a production environment
as the causedBy
error messages tend to be system-level messages that could be exposed to clients
if the error is being thrown back to the client.
Method: BaseError#formatMessage(...formatParams)
See the sprintf-js
package for usage.
// specify the database specific error code
// Transforms the message to:
// 'There was a database failure, SQL err code %s' ->
// 'There was a database failure, SQL err code SQL_ERR_1234',
err.formatMessage('SQL_ERR_1234')
The message can be accessed via the .message
property.
Method: BaseError#convert<T = any>() : T
This is useful if you need to convert the error into another type. This type can be another error or some other data type.
For example, Apollo GraphQL prefers that any errors thrown from a GQL endpoint is an error that extends ApolloError
.
You might find yourself doing the following pattern if your resolver happens to throw a BaseError
:
import { GraphQLError } from 'graphql';
import { BaseError } from 'new-error';
import { ApolloError, ForbiddenError } from 'apollo-server';
const server = new ApolloServer({
typeDefs,
resolvers,
formatError: (err: GraphQLError) => {
const origError = error.originalError;
if (origError instanceof BaseError) {
// re-map the BaseError into an Apollo error type
switch(origError.getCode()) {
case 'PERMISSION_REQUIRED':
return new ForbiddenError(err.message)
default:
return new ApolloError(err.message)
}
}
return err;
},
});
Trying to switch for every single code / subcode can be cumbersome.
Instead of using this pattern, do the following so that your conversions can remain in one place:
import { GraphQLError } from 'graphql';
import { BaseError, ErrorRegistry } from 'new-error';
import { ApolloError, ForbiddenError } from 'apollo-server';
const errors = {
PERMISSION_REQUIRED: {
className: 'PermissionRequiredError',
code: 'PERMISSION_REQUIRED',
// Define a conversion function that is called when BaseError#convert() is called
// error is the BaseError
onConvert: (error) => {
return new ForbiddenError(error.message)
}
},
AUTH_REQUIRED: {
className: 'AuthRequiredError',
code: 'AUTH_REQUIRED'
}
}
const errorSubCodes = {
ADMIN_PANEL_RESTRICTED: {
message: 'Access scope required: admin',
onConvert: (error) => {
return new ForbiddenError('Admin required')
}
},
EDITOR_SECTION_RESTRICTED: {
message: 'Access scope required: editor',
}
}
const errRegistry = new ErrorRegistry(errors, errorSubCodes, {
onCreateError: (err) => {
// if an onConvert handler has not been defined, default to ApolloError
if (!err.hasOnConvertDefined()) {
err.setOnConvert((err) => {
if (process.env.NODE_ENV !== 'production') {
// return full error details
return new ApolloError(err.message, err.getCode(), err.toJSON());
}
// in production, we don't want to expose codes that are internal
return new ApolloError('Internal server error', 'INTERNAL_SERVER_ERROR', {
errId: err.getErrorId(),
});
});
}
}
})
const server = new ApolloServer({
typeDefs,
resolvers,
// errors thrown from the resolvers come here
formatError: (err: GraphQLError) => {
const origError = error.originalError;
if (origError instanceof BaseError) {
// log out the original error
console.log(origError.toJSON())
// Convert out to an Apollo error type
return origError.convert()
}
// log out the apollo error
// you would probably want to see if you can convert this
// to a BaseError type in your code where this may be thrown from
console.log(err)
return err;
},
});
const resolvers = {
Query: {
adminSettings(parent, args, context, info) {
// err.convert() will call onConvert() of ADMIN_PANEL_RESTRICTED (low level defs have higher priority)
throw errRegistry.newError('PERMISSION_REQUIRED', 'ADMIN_PANEL_RESTRICTED')
},
editorSettings(parent, args, context, info) {
// err.convert() will call onConvert() of PERMISSION_REQUIRED since EDITOR_SECTION_RESTRICTED does not
// have the onConvert defined
throw errRegistry.newError('PERMISSION_REQUIRED', 'EDITOR_SECTION_RESTRICTED')
},
checkAuth(parent, args, context, info) {
// err.convert() will return ApolloError from onCreateError() since onConvert() is not defined for either AUTH_REQUIRED or EDITOR_SECTION_RESTRICTED
throw errRegistry.newError('AUTH_REQUIRED', 'EDITOR_SECTION_RESTRICTED')
},
checkAuth2(parent, args, context, info) {
// err.convert() will return ApolloError from onCreateError() since onConvert() is not defined for either AUTH_REQUIRED
throw errRegistry.newBareError('AUTH_REQUIRED', 'Some error message')
},
permRequired(parent, args, context, info) {
// err.convert() will call onConvert() of PERMISSION_REQUIRED
throw errRegistry.newBareError('PERMISSION_REQUIRED', 'Some error message')
}
}
}
Method: BaseError#withSafeMetadata(data = {})
Safe metadata would be any kind of data that you would be ok with exposing to a client, like an HTTP response.
err.withSafeMetadata({
errorId: 'err-12345',
moreData: 1234
})
// can be chained to append more data
.withSafeMetadata({
requestId: 'req-12345'
})
This can also be written as:
err.withSafeMetadata({
errorId: 'err-12345',
moreData: 1234
})
// This will append requestId to the metadata
err.withSafeMetadata({
requestId: 'req-12345'
})
Method: BaseError#withMetadata(data = {})
Internal metadata would be any kind of data that you would not be ok with exposing to a client, but would be useful for internal development / logging purposes.
err.withMetadata({
email: 'test@test.com'
})
// can be chained to append more data
.withMetadata({
userId: 'user-abcd'
})
Method: BaseError#toJSONSafe(fieldsToOmit = [])
Generates output that would be safe for client consumption.
name
message
causedBy
type
logLevel
BaseError#withMetadata()
err.withSafeMetadata({
requestId: 'req-12345'
})
// you can remove additional fields by specifying property names in an array
//.toJSONSafe(['code']) removes the code field from output
.toJSONSafe()
Produces:
{
code: 'ERR_INT_500',
subCode: 'DB_0001',
statusCode: 500,
meta: { requestId: 'req-12345' }
}
Method: BaseError#toJSON(fieldsToOmit = [])
Generates output that would be suitable for internal use.
name
type
message
causedBy
BaseError#withMetadata()
and BaseError#withSafeMetadata()
is includederr.withSafeMetadata({
reqId: 'req-12345',
}).withMetadata({
email: 'test@test.com'
})
// you can remove additional fields by specifying property names in an array
//.toJSON(['code', 'statusCode']) removes the code and statusCode field from output
.toJSON()
Produces:
{
name: 'InternalServerError',
code: 'ERR_INT_500',
message: 'There was a database failure, SQL err code %s',
type: 'DATABASE_FAILURE',
subCode: 'DB_0001',
statusCode: 500,
meta: { errorId: 'err-12345', requestId: 'req-12345' },
stack: 'InternalServerError: There was a database failure, SQL err code %s\n' +
' at ErrorRegistry.newError (new-error/src/ErrorRegistry.ts:128:12)\n' +
' at Object.<anonymous> (new-error/src/test.ts:55:25)\n' +
' at Module._compile (internal/modules/cjs/loader.js:1158:30)\n' +
' at Module._compile (new-error/node_modules/source-map-support/source-map-support.js:541:25)\n' +
' at Module.m._compile (/private/var/folders/mx/b54hc2lj3fbfsndkv4xmz8d80000gn/T/ts-node-dev-hook-17091160954051898.js:57:25)\n' +
' at Module._extensions..js (internal/modules/cjs/loader.js:1178:10)\n' +
' at require.extensions.<computed> (/private/var/folders/mx/b54hc2lj3fbfsndkv4xmz8d80000gn/T/ts-node-dev-hook-17091160954051898.js:59:14)\n' +
' at Object.nodeDevHook [as .ts] (new-error/node_modules/ts-node-dev/lib/hook.js:61:7)\n' +
' at Module.load (internal/modules/cjs/loader.js:1002:32)\n' +
' at Function.Module._load (internal/modules/cjs/loader.js:901:14)'
}
The BaseError
config onPreToJSONData
/ onPreToJSONSafeData
options allow post-processing of the data. This is useful if you want to decorate your data for all new
errors created.
const errRegistry = new ErrorRegistry(errors, errorSubCodes, {
baseErrorConfig: {
// called when toJSON is called
onPreToJSONData: (data) => {
// we want all new errors to contain a date field
data.date = new Date().tostring()
// add some additional metadata
// data.meta might be empty if omitEmptyMetadata is enabled
if (data.meta) {
data.meta.moreData = 'test'
}
return data
}
}
})
const err = errRegistry.newError('INTERNAL_SERVER_ERROR', 'DATABASE_FAILURE')
.withErrorId('err-1234')
.formatMessage('SQL_1234')
// should produce the standard error structure, but with the new fields added
console.log(err.toJSON())
name
property (not present when using toJSONSafe()
), then only a BaseError
instance can be returned.causedBy
data. As a result, it will be copied as-is.JSON.parse()
as JSON.parse()
in its raw form is susceptible to
prototype pollution
if the parse function does not have a proper sanitization function. It is up to the developer to properly
trust / sanitize / parse the data.ErrorRegistry#fromJSON()
methodThis method will attempt to deserialize into a registered error type via the name
property. If it is unable to, a BaseError
instance is
returned instead.
ErrorRegistry#fromJSON(data: object, [options]: DeserializeOpts): IBaseError
data
: Data that is the output of BaseError#toJSON()
. The data must be an object, not a string.options
: Optional deserialization options.interface DeserializeOpts {
/**
* Fields from meta to pluck as a safe metadata field
*/
safeMetadataFields?: {
// the value must be set to true.
[key: string]: true
}
}
Returns a BaseError
instance or an instance of a registered error type.
import { ErrorRegistry } from 'new-error'
const errors = {
INTERNAL_SERVER_ERROR: {
className: 'InternalServerError',
code: 'ERR_INT_500',
statusCode: 500,
logLevel: 'error'
}
}
const errorSubCodes = {
DATABASE_FAILURE: {
message: 'There was a database failure, SQL err code %s',
subCode: 'DB_0001',
statusCode: 500,
logLevel: 'error'
}
}
const errRegistry = new ErrorRegistry(errors, errorSubCodes)
const data = {
'errId': 'err-123',
'code': 'ERR_INT_500',
'subCode': 'DB_0001',
'message': 'test message',
'meta': { 'safeData': 'test454', 'test': 'test123' },
// maps to className in the high level error def
'name': 'InternalServerError',
'statusCode': 500,
'causedBy': 'test',
'stack': 'abcd'
}
// err should be an instance of InternalServerError
const err = errRegistry.fromJSON(data, {
safeMetadataFields: {
safeData: true
}
})
static BaseError#fromJSON()
methodIf you are not using the registry, you can deserialize using this method. This also applies to any class that extends
BaseError
.
static BaseError#fromJSON(data: object, [options]: DeserializeOpts): IBaseError
data
: Data that is the output of BaseError#toJSON()
. The data must be an object, not a string.options
: Optional deserialization options.Returns a BaseError
instance or an instance of the class that extends it.
import { BaseError } from 'new-error'
// assume we have serialized error data
const data = {
code: 'ERR_INT_500',
subCode: 'DB_0001',
statusCode: 500,
errId: 'err-1234',
meta: { requestId: 'req-12345', safeData: '123' }
}
// deserialize
// specify meta field assignment - fields that are not assigned will be assumed as withMetadata() type data
const err = BaseError.fromJSON(data, {
// (optional) Fields to pluck from 'meta' to be sent to BaseError#safeMetadataFields()
// value must be set to 'true'
safeMetadataFields: {
safeData: true
}
})
If the name
property is present in the serialized data if it was serialized with toJson()
, you can use a switch
to map to an instance:
const data = {
// be sure that you trust the source of the deserialized data!
// anyone can modify the 'name' property to whatever
name: 'InternalServerError',
code: 'ERR_INT_500',
subCode: 'DB_0001',
statusCode: 500,
errId: 'err-1234',
meta: { requestId: 'req-12345', safeData: '123' }
}
let err = null
switch (data.name) {
case 'InternalServerError':
// assume InternalServerError extends BaseError
return InternalServerError.fromJSON(data)
default:
return BaseError.fromJSON(data)
}
2.2.0 - Wed Jun 14 2023 12:01:40
Contributor: Theo Gravity
withRequestId()
/ getRequestId()
to BaseError
FAQs
A production-grade error creation and serialization library designed for Typescript
The npm package new-error receives a total of 531 weekly downloads. As such, new-error popularity was classified as not popular.
We found that new-error demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.