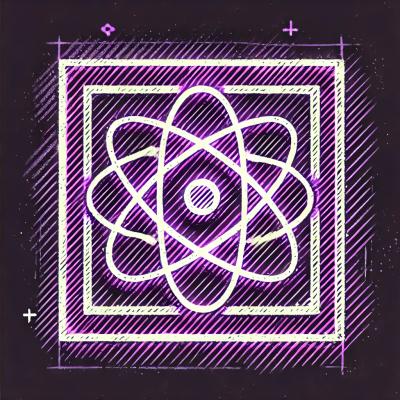
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
newtype-ts
Advanced tools
The newtype-ts package provides utilities for creating and working with newtypes in TypeScript. Newtypes are a way to create distinct types from existing ones without runtime overhead, which can help with type safety and code clarity.
Creating Newtypes
This feature allows you to create newtypes, which are distinct types derived from existing ones. The example demonstrates creating a newtype for meters and using an isomorphism to wrap and unwrap values.
import { Newtype, iso } from 'newtype-ts';
type Meter = Newtype<{ readonly Meter: unique symbol }, number>;
const meterIso = iso<Meter>();
const distance: Meter = meterIso.wrap(5);
const value: number = meterIso.unwrap(distance);
Using Newtypes with Functions
This feature shows how to use newtypes with functions to ensure type safety. The example defines newtypes for meters and seconds, and a function to calculate speed, ensuring that only the correct types are used.
import { Newtype, iso } from 'newtype-ts';
type Meter = Newtype<{ readonly Meter: unique symbol }, number>;
const meterIso = iso<Meter>();
type Second = Newtype<{ readonly Second: unique symbol }, number>;
const secondIso = iso<Second>();
const speed = (distance: Meter, time: Second): number => meterIso.unwrap(distance) / secondIso.unwrap(time);
const distance: Meter = meterIso.wrap(100);
const time: Second = secondIso.wrap(10);
const result = speed(distance, time); // 10
Combining Newtypes
This feature demonstrates how to combine newtypes to create more complex types. The example shows how to calculate speed from distance and time, and wrap the result in a newtype for speed.
import { Newtype, iso } from 'newtype-ts';
type Meter = Newtype<{ readonly Meter: unique symbol }, number>;
const meterIso = iso<Meter>();
type Second = Newtype<{ readonly Second: unique symbol }, number>;
const secondIso = iso<Second>();
type Speed = Newtype<{ readonly Speed: unique symbol }, number>;
const speedIso = iso<Speed>();
const calculateSpeed = (distance: Meter, time: Second): Speed => speedIso.wrap(meterIso.unwrap(distance) / secondIso.unwrap(time));
const distance: Meter = meterIso.wrap(100);
const time: Second = secondIso.wrap(10);
const speed: Speed = calculateSpeed(distance, time);
io-ts is a runtime type system for IO decoding/encoding in TypeScript. It provides a way to define types that can be checked at runtime, which is useful for validating external data. Unlike newtype-ts, io-ts focuses on runtime type validation rather than creating distinct types at compile time.
runtypes is a library for runtime validation and type checking in TypeScript. It allows you to define types that can be validated at runtime, similar to io-ts. While runtypes provides runtime type safety, newtype-ts focuses on creating distinct types at compile time without runtime overhead.
typescript-is is a TypeScript transformer that allows you to perform type checking at runtime. It provides a way to assert types and validate data at runtime. Unlike newtype-ts, which focuses on compile-time type safety, typescript-is provides runtime type assertions.
A common programming practice is to define a type whose representation is identical to an existing one but which has a separate identity in the type system.
type USD = number
type EUR = number
const myamount: USD = 1
declare function change(usd: USD): EUR
declare function saveAmount(eur: EUR): void
saveAmount(change(myamount)) // ok
saveAmount(myamount) // opss... this is also ok because both EUR and USD are type alias of number!
Let's define a newtype for the EUR currency
import { Newtype, iso } from 'newtype-ts'
interface EUR extends Newtype<{ readonly EUR: unique symbol }, number> {}
// isoEUR: Iso<EUR, number>
const isoEUR = iso<EUR>()
// myamount: EUR
const myamount = isoEUR.wrap(0.85)
// n: number = 0.85
const n = isoEUR.unwrap(myamount)
declare function saveAmount(eur: EUR): void
saveAmount(0.85) // static error: Argument of type '0.85' is not assignable to parameter of type 'EUR'
saveAmount(myamount) // ok
By definition a "newtype" must have the exact same runtime representation as the value that it stores, e.g. a value of type EUR
is just a number
at runtime.
For the Iso
type, see the monocle-ts documentation.
An Integer
is a refinement of number
import { Newtype, prism } from 'newtype-ts'
interface Integer extends Newtype<{ readonly Integer: unique symbol }, number> {}
const isInteger = (n: number) => Number.isInteger(n);
// prismInteger: Prism<number, Integer>
const prismInteger = prism<Integer>(isInteger)
// oi: Option<Integer>
const oi = prismInteger.getOption(2)
declare function f(i: Integer): void
f(2) // static error: Argument of type '2' is not assignable to parameter of type 'Integer'
oi.map(f) // ok
For the Prism
type, see the monocle-ts documentation.
Char
Integer
Negative
NegativeInteger
NonNegative
NonNegativeInteger
NonPositive
NonPositiveInteger
NonEmptyString
NonZero
NonZeroInteger
Positive
PositiveInteger
import { NonZero, prismNonZero } from 'newtype-ts/lib/NonZero'
// a total function
const safeDivide = (numerator: number, denominator: NonZero): number => {
return numerator / prismNonZero.reverseGet(denominator)
}
// result: Option<number>
const result = prismNonZero.getOption(2).map(denominator => safeDivide(2, denominator))
The stable version is tested against TypeScript 3.1.6
const double = n => n * 2
const doubleEUR = eurIso.modify(double)
Test double(2)
vs doubleEUR(eurIso.wrap(2))
Results (node v8.9.3
)
double x 538,301,203 ops/sec ±0.45% (87 runs sampled)
doubleEUR x 536,575,600 ops/sec ±0.27% (87 runs sampled)
const double = (n: number): number => n * 2
// doubleEUR: (s: EUR) => EUR
const doubleEUR = eurIso.modify(double)
import { over } from 'newtype-ts'
interface USD extends Newtype<{ readonly USD: unique symbol }, number> {}
const USDFromEUR = (n: number): number => n * 1.18
// getter: Getter<EUR, USD>
const getter = over<EUR, USD>(USDFromEUR)
// usd: USD
const usd = getter.get(eur)
For the Getter
type, see monocle-ts documentation.
0.2.4
Integer
prism (@tatchi)FAQs
Implementation of newtypes in TypeScript
The npm package newtype-ts receives a total of 367,735 weekly downloads. As such, newtype-ts popularity was classified as popular.
We found that newtype-ts demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.