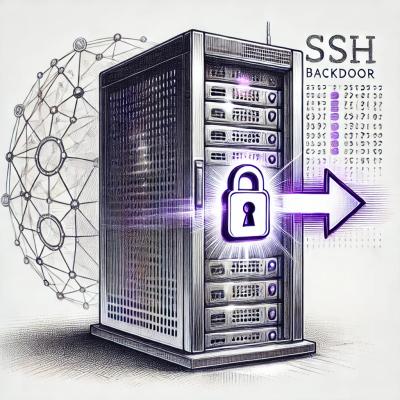
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
next-page-tester
Advanced tools
The missing DOM integration testing tool for Next.js.
Given a Next.js route, this library will render the matching page in JSDOM, provided with the expected props derived from Next.js' routing system and data fetching methods.
import { getPage } from 'next-page-tester';
import { screen, fireEvent } from '@testing-library/react';
describe('Blog page', () => {
it('renders blog page', async () => {
const { render } = await getPage({
route: '/blog/1',
});
render();
expect(screen.getByText('Blog')).toBeInTheDocument();
fireEvent.click(screen.getByText('Link'));
await screen.findByText('Linked page');
});
});
The idea behind this library is to reproduce as closely as possible the way Next.js works without spinning up servers, and render the output in a local JSDOM environment.
In order to provide a valuable testing experience next-page-tester
replicates the rendering flow of an actual next.js
application:
head
element)The mounted application is interactive and can be tested with any DOM testing library (like @testing-library/react
).
next-page-tester
will take care of:
getServerSideProps
, getInitialProps
or getStaticProps
) if the case_app
and _document
componentsLink
, router.push
, router.replace
redirect
returnsnext/router
, next/head
, next/link
, next/config
and environment variablesgetPage
accepts an option object and returns 4 values:
import { getPage } from 'next-page-tester';
const { render, serverRender, serverRenderToString, page } = await getPage({
options,
});
Type: () => { nextRoot: HTMLElement<NextRoot> }
Returns: #__next
root element element.
Unless you have an advanced use-case, you should mostly use this method. Under the hood it calls serverRender()
and then mounts/hydrates the React application into JSDOM #__next
root element. This is what users would get/see when they visit a page.
Type: () => { nextRoot: HTMLElement<NextRoot> }
Returns: #__next
root element element.
Inject the output of server side rendering into the DOM but doesn't mount React. Use it to test how Next.js renders in the following scenarios:
Type: () => { html: string }
Render the output of server side rendering as HTML string. This is a pure method without side-effects.
Type: React.ReactElement<AppElement>
React element of the application.
Property | Description | type | Default |
---|---|---|---|
route (mandatory) | Next route (must start with / ) | string | - |
req | Enhance default mocked request object | req => req | - |
res | Enhance default mocked response object | res => res | - |
router | Enhance default mocked Next router object | router => router | - |
useApp | Render custom App component | boolean | true |
useDocument (experimental) | Render Document component | boolean | false |
nextRoot | Absolute path to Next.js root folder | string | auto detected |
nonIsolatedModules | Array of modules that should use the same module instance in server and client environment | string[] | - |
dotenvFile | Relative path to a .env file holding environment variables | string | - |
Since Next.js is not designed to run in a JSDOM environment we need to setup the default JSDOM to allow a smoother testing experience:
window.scrollTo
mockIntersectionObserver
mockRun initTestHelpers
in your global tests setup (in case of Jest It is setupFilesAfterEnv
file):
import { initTestHelpers } from 'next-page-tester';
initTestHelpers();
Until Jest v27 is published, you might need to patch jest
in order to load modules with proper server/client environments. Don't do this until you actually encounter issues.
patch-package
and follow its setup instructionsnode_modules/jest-runtime/build/index.js
file and replicate this commitnpx patch-package jest-runtime
or yarn patch-package jest-runtime
Under examples folder we're documenting the testing cases which next-page-tester
enables.
req
and res
objects are mocked with node-mocks-http@types/react-dom
and @types/webpack
when using Typescript in strict
mode due to this buguseDocument
optionuseDocument
option is partially implemented and might be unstable.
next-page-tester
focuses on supporting only the last major version of Next.js:
next-page-tester | next.js |
---|---|
v0.1.0 - v0.7.0 | v9.X.X |
v0.8.0 + | v10.X.X |
You can set cookies by appending them to document.cookie
before calling getPage
. next-page-tester
will propagate cookies to ctx.req.headers.cookie
so they will be available to data fetching methods. This also applies to subsequent fetching methods calls triggered by client side navigation.
test('authenticated page', async () => {
document.cookie = 'SessionId=super=secret';
document.cookie = 'SomeOtherCookie=SomeOtherValue';
const { render } = await getPage({
route: '/authenticated',
});
render();
});
Note: document.cookie
does not get cleaned up automatically. You'll have to clear it manually after each test to keep everything in isolation.
Next.js Link
component invokes window.scrollTo
on click which is not implemented in JSDOM environment. In order to fix the error you should set up your test environment or provide your own window.scrollTo
mock.
This warning means that your page renders differently between server and browser. This can be an expected behavior or signal a bug in your code.
The same error could also be triggered when you import modules that, in order to work, need to preserve module identity throughout the entire SSR cycle (from the moment they are imported to SSR rendering): e.g. React.Context
or css-in-js
libraries. In this case you can list these modules in nonIsolatedModules
option to preserve their identity: see styletron-react example
Environment variables are automatically loaded from next.config.js
file and from the first available dotfile among: .env.test.local
, .env.test
and .env
.
trailingSlash
option_error
page404
pagedebug
option to log execution infoThanks goes to these wonderful people (emoji key):
Andrea Carraro 💻 🚇 ⚠️ 🚧 | Matej Šnuderl 💻 🚇 ⚠️ 👀 🤔 📖 | Jason Williams 🤔 | arelaxend 🐛 | kfazinic 🐛 | Tomasz Rondio 🐛 | Alexander Mendes 💻 |
Jan Sepke 🐛 | DavidOrchard 🐛 |
This project follows the all-contributors specification. Contributions of any kind welcome!
0.18.0
renderPage
dotenvFile
option to support environment variables loadinggetServerSideProps
's notFound
returnFAQs
Enable DOM integration testing on Next.js pages
The npm package next-page-tester receives a total of 3,247 weekly downloads. As such, next-page-tester popularity was classified as popular.
We found that next-page-tester demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.