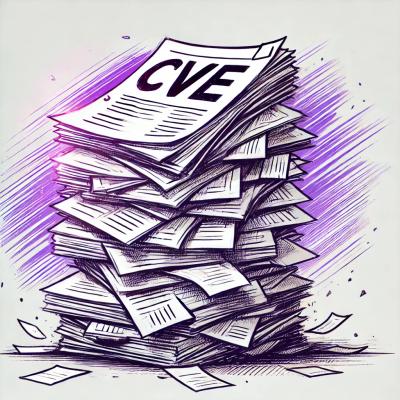
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
next-pipe-middleware
Advanced tools
This is a library for building Next.js middleware declaratively. You can create highly readable and manageable middleware by piping multiple functions together.
This is a library for building Next.js middleware declaratively. You can create highly readable and manageable middleware by piping multiple functions together.
Next.js v12.2.0+ (Middleware support)
Pass NextRequest, NextResponse, and arrays of multiple middleware to pipeMiddleware
function.
export default async function middleware(req: NextRequest) {
return pipeMiddleware(req, NextResponse.next(), [
fooMiddleware,
barMiddleware,
hogeMiddleware,
])
}
Each middleware function (PipeableMiddleware
) differs from the Next.js middleware functions only in that it takes a NextResponse as its second argument:
const fooMiddleware: PipeableMiddleware = async (req, res) => {
res.cookies.set('foo', 'bar')
return res;
};
If you want to control execution of middleware according to the page path, pass an object containing a matcher function as the second element of the tuple
export default async function middleware(req: NextRequest) {
return pipeMiddleware(req, NextResponse.next(), [
basicAuthMiddleware,
[redirectMiddleware, {matcher: (path) => path.startsWith('/secret')}],
[refreshTokenMiddleware, {matcher: (path) => path.startsWith('/my')}],
])
}
If you want to terminate the entire process on a particular piece of middleware (i.e., you do not want subsequent pieces of middleware to run), change the response format as follows
const basicAuth: PipeableMiddleware = async (req, res) => {
const success = validateBasicAuth(req);
if (success) {
return res;
} else {
return {
res: NextResponse.rewrite(/**/),
final: true,
}; // terminate process after this middleware by returning object with `final: true` and `res`
}
};
export default async function middleware(req: NextRequest) {
return pipeMiddleware(req, NextResponse.next(), [
basicAuthMiddleware, // if basic auth failed, the process ends here.
redirectMiddleware,
refreshTokenMiddleware
])
}
If you want to implement the following at the middleware file:
Without this library, you would have to write codes like this:
export default async function middleware(req: NextRequest) {
const res = NextResponse.next()
const success = await basicAuthMiddleware(req, res);
if (!success) {
return res;
}
if (req.url.startsWith('/secret')) {
const [shouldRedirect, redirectRes] = await redirectMiddleware(req, res);
if (shouldRedirect) {
return redirectRes;
}
}
if (req.url.startsWith('/my')) {
await refreshTokenMiddleware(req, res);
}
return res;
}
It is difficult to know what kind of process the middleware consists of, because it is necessary to check whether the process should be terminated depending on the response, or whether it should be executed according to the path, etc.
This library allows you to write what you want to do declaratively and readably as above.
FAQs
This is a library for building Next.js complex middleware declaratively. You can create highly readable and manageable middleware by composing multiple functions together.
We found that next-pipe-middleware demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.