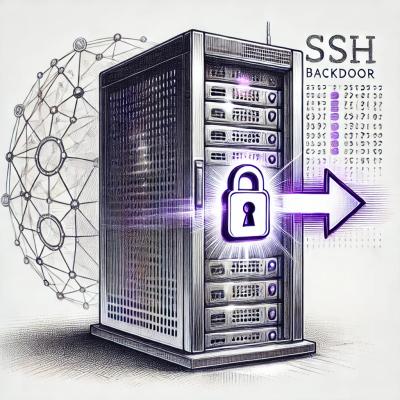
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
next-plugin-websocket
Advanced tools
Add WebSocket support to Next.js API routes
/api
pageyarn add next-plugin-websocket
Export a socket
handler function from a Next.js API route. The first argument will be the WebSocket client and the second argument will be the original request object.
import { appRouter } from "@/server/routers/_app";
import { NextApiHandler } from "next";
import { NextWebSocketHandler } from "next-plugin-websocket";
export const socket: NextWebSocketHandler = (client, req) => {
client.on("message", (msg) => {
client.send(msg);
});
};
const handler: NextApiHandler = (req, res) => {
res.status(405).end();
};
export default handler;
import { appRouter } from "@/server/routers/_app";
import { createNextApiHandler } from "@trpc/server/adapters/next";
import { applyWSSHandler } from "@trpc/server/adapters/ws";
import { NextWebSocketHandler } from "next-plugin-websocket";
import { WebSocketServer } from "ws";
export const socket: NextWebSocketHandler = (client, req) => {
const wss = new WebSocketServer({ noServer: true });
applyWSSHandler({ wss, router: appRouter });
wss.emit("connection", client, req);
};
export default createNextApiHandler({
router: appRouter,
});
TODO
FAQs
Add WebSocket support to Next.js API routes
The npm package next-plugin-websocket receives a total of 2 weekly downloads. As such, next-plugin-websocket popularity was classified as not popular.
We found that next-plugin-websocket demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.