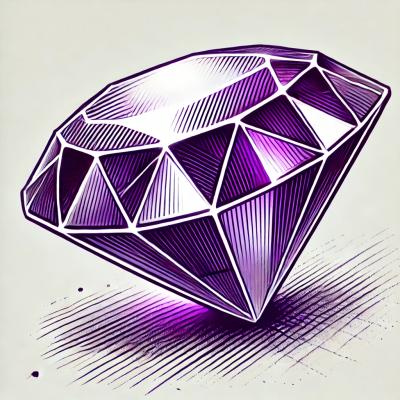
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
next-sanity
Advanced tools
Sanity.io toolkit for Next.js.
Features:
next-sanity
Running groq queries
next-sanity/preview
Live real-time preview
next-sanity/studio
next-sanity/webhook
v2
NextStudioGlobalStyle
is removedServerStyleSheetDocument
is removedisWorkspaceWithTheme
and isWorkspaces
utils are no longer exporteduseBackgroundColorsFromTheme
, useBasePath
, useConfigWithBasePath
, and useTextFontFamilyFromTheme
, hooks are removedNextStudioHead
component has moved from next-sanity/studio
to next-sanity/studio/head
v1
v0.4
npm install next-sanity @portabletext/react @sanity/image-url
yarn add next-sanity @portabletext/react @sanity/image-url
pnpm install next-sanity @portabletext/react @sanity/image-url
next-sanity/studio
peer dependenciesWhen using npm
newer than v7
you should end up with needed dependencies like sanity
and styled-components
when you npm install next-sanity
. For other package managers you may need to do some extra steps.
npx install-peerdeps --yarn next-sanity
You can either setup auto-install-peers
and pnpm install next-sanity
is enough, or:
npx install-peerdeps --pnpm next-sanity
next-sanity
Running groq queriesimport {createClient, groq} from 'next-sanity'
const projectId = process.env.NEXT_PUBLIC_SANITY_PROJECT_ID // "pv8y60vp"
const dataset = process.env.NEXT_PUBLIC_SANITY_DATASET // "production"
const apiVersion = process.env.NEXT_PUBLIC_SANITY_API_VERSION // "2022-11-16"
const client = createClient({
projectId,
dataset,
apiVersion, // https://www.sanity.io/docs/api-versioning
useCdn: true, // if you're using ISR or only static generation at build time then you can set this to `false` to guarantee no stale content
})
const data = await client.fetch(groq`*[]`)
appDir
, React Server Components and cachingAs @sanity/client
will only sometimes use fetch
under the hood, it depends on the environment, it's best to implement the cache function to ensure reliable caching.
import {createClient, groq} from 'next-sanity'
import {cache} from 'react'
const projectId = process.env.NEXT_PUBLIC_SANITY_PROJECT_ID // "pv8y60vp"
const dataset = process.env.NEXT_PUBLIC_SANITY_DATASET // "production"
const apiVersion = process.env.NEXT_PUBLIC_SANITY_API_VERSION // "2022-11-16"
const client = createClient({
projectId,
dataset,
apiVersion, // https://www.sanity.io/docs/api-versioning
useCdn: true, // if you're using ISR or only static generation at build time then you can set this to `false` to guarantee no stale content
})
// Wrap the cache function in a way that reuses the TypeScript definitions
const clientFetch = cache(client.fetch.bind(client))
// Now use it just like before, fully deduped, cached and optimized by react
const data = await clientFetch(groq`*[]`)
// You can use the same generics as before
const total = await clientFetch<number>(groq`count*()`)
next-sanity/preview
Live real-time previewYou can implement real-time client side preview using definePreview
. It works by streaming the whole dataset to the browser, which it keeps updated using listeners and Mendoza patches. When it receives updates, then the query is run against the client-side datastore using groq-js.
It uses @sanity/preview-kit
under the hood, which can be used in frameworks other than Nextjs if it supports React 18 Suspense APIs.
When running next dev
locally these examples start and exit preview mode by opening localhost:3000/api/preview and localhost:3000/api/exit-preview.
Pros:
Cons:
dual
loginMethod in Sanity Studio:
pages/api/preview.ts
:
export default function preview(req, res) {
res.setPreviewData({})
res.writeHead(307, {Location: '/'})
res.end()
}
pages/api/exit-preview.ts
:
export default function exit(req, res) {
res.clearPreviewData()
res.writeHead(307, {Location: '/'})
res.end()
}
components/DocumentsCount.tsx
:
import groq from 'groq'
export const query = groq`count(*[])`
export function DocumentsCount({data}) {
return (
<>
Documents: <strong>{data}</strong>
</>
)
}
lib/sanity.client.ts
import {createClient} from 'next-sanity'
const projectId = process.env.NEXT_PUBLIC_SANITY_PROJECT_ID // "pv8y60vp"
const dataset = process.env.NEXT_PUBLIC_SANITY_DATASET // "production"
const apiVersion = process.env.NEXT_PUBLIC_SANITY_API_VERSION // "2022-11-16"
export const client = createClient({projectId, dataset, apiVersion})
lib/sanity.preview.ts
import {definePreview} from 'next-sanity/preview'
import {projectId, dataset} from 'lib/sanity.client'
function onPublicAccessOnly() {
throw new Error(`Unable to load preview as you're not logged in`)
}
export const usePreview = definePreview({projectId, dataset, onPublicAccessOnly})
components/PreviewDocumentsCount.ts
:
'use client'
import {usePreview} from 'lib/sanity.preview'
import {query, DocumentsCount} from 'components/DocumentsCount'
export default function PreviewDocumentsCount() {
const data = usePreview(null, query)
return <DocumentsCount data={data} />
}
/pages
directorypages/index.tsx
:
import {PreviewSuspense} from 'next-sanity/preview'
import {lazy} from 'react'
import {DocumentsCount, query} from 'components/DocumentsCount'
import {client} from 'lib/sanity.client'
const PreviewDocumentsCount = lazy(() => import('components/PreviewDocumentsCount'))
export const getStaticProps = async ({preview = false}) => {
if (preview) {
return {props: {preview}}
}
const data = await client.fetch(query)
return {props: {preview, data}}
}
export default function IndexPage({preview, data}) {
if (preview) {
return (
<PreviewSuspense fallback="Loading...">
<PreviewDocumentsCount />
</PreviewSuspense>
)
}
return <DocumentsCount data={data} />
}
/app
directory (experimental)We support the new appDir
mode in Next, but please note that appDir
shouldn't be used in production before Vercel says it's stable.
components/PreviewSuspense.tsx
:
'use client'
// Once rollup supports 'use client' module directives then 'next-sanity' will include them and this re-export will no longer be necessary
export {PreviewSuspense as default} from 'next-sanity/preview'
app/page.tsx
:
import {previewData} from 'next/headers'
import PreviewSuspense from 'components/PreviewSuspense'
import {DocumentsCount, query} from 'components/DocumentsCount'
import PreviewDocumentsCount from 'components/PreviewDocumentsCount'
import {client} from 'lib/sanity.client'
import {cache} from 'react'
// Enable NextJS to cache and dedupe queries
const clientFetch = cache(client.fetch.bind(client))
export default async function IndexPage() {
if (previewData()) {
return (
<PreviewSuspense fallback="Loading...">
<PreviewDocumentsCount />
</PreviewSuspense>
)
}
const data = await clientFetch(query)
return <DocumentsCount data={data} />
}
By providing a read token (Sanity API token with viewer
rights) you override the built-in auth and get more control and flexibility.
Pros:
Cons:
token
in your js bundle, or preventing the /api/preview?secret=${secret}
from being easily guessable.@sanity/groq-store
currently requires event-source-polyfill
since native window.EventSource
does not support setting Authorization
headers needed for the token auth.pages/api/preview.ts
:
import getSecret from 'lib/getSecret'
export default async function preview(req, res) {
// The secret can't be stored in an env variable with a NEXT_PUBLIC_ prefix, as it would make you vulnerable to leaking the token to anyone.
// If you don't have an custom API with authentication that can handle checking secrets, you may use https://github.com/sanity-io/sanity-studio-secrets to store the secret in your dataset.
const secret = await getSecret()
// This is the most common way to check for auth, but we encourage you to use your existing auth infra to protect your token and securely transmit it to the client
if (!req.query.secret || req.query.secret !== secret) {
return res.status(401).json({message: 'Invalid secret'})
}
res.setPreviewData({token: process.env.SANITY_API_READ_TOKEN})
res.writeHead(307, {Location: '/'})
res.end()
}
pages/api/exit-preview.ts
:
export default function exit(req, res) {
res.clearPreviewData()
res.writeHead(307, {Location: '/'})
res.end()
}
components/DocumentsCount.tsx
:
import groq from 'groq'
export const query = groq`count(*[])`
export function DocumentsCount({data}) {
return (
<>
Documents: <strong>{data}</strong>
</>
)
}
lib/sanity.client.ts
import {createClient} from 'next-sanity'
const projectId = process.env.NEXT_PUBLIC_SANITY_PROJECT_ID // "pv8y60vp"
const dataset = process.env.NEXT_PUBLIC_SANITY_DATASET // "production"
const apiVersion = process.env.NEXT_PUBLIC_SANITY_API_VERSION // "2022-11-16"
export const client = createClient({projectId, dataset, apiVersion})
lib/sanity.preview.ts
import {definePreview} from 'next-sanity/preview'
import {projectId, dataset} from 'lib/sanity.client'
export const usePreview = definePreview({projectId, dataset})
components/PreviewDocumentsCount.tsx
:
'use client'
import {usePreview} from 'lib/sanity.preview'
import {query, DocumentsCount} from 'components/DocumentsCount'
export default function PreviewDocumentsCount({token}) {
const data = usePreview(token, query)
return <DocumentsCount data={data} />
}
/pages
directorypages/index.tsx
:
import {PreviewSuspense} from 'next-sanity/preview'
import {lazy} from 'react'
import {DocumentsCount, query} from 'components/DocumentsCount'
import {client} from 'lib/sanity.client'
// Wrapping preview components in React.lazy ensures visitors not on preview mode doesn't load any JS related to it
const PreviewDocumentsCount = lazy(() => import('components/PreviewDocumentsCount'))
export const getStaticProps = async ({preview = false, previewData = {}}) => {
if (preview && previewData?.token) {
return {props: {preview, token: previewData.token}}
}
const data = await client.fetch(query)
return {props: {preview, data}}
}
export default function IndexPage({preview, token, data}) {
if (preview) {
return (
<PreviewSuspense fallback="Loading...">
<PreviewDocumentsCount token={token} />
</PreviewSuspense>
)
}
return <DocumentsCount data={data} />
}
/app
directory (experimental)We support the new appDir
mode in Next, but please note that appDir
shouldn't be used in production before Vercel says it's stable.
components/PreviewSuspense.tsx
:
'use client'
// Once rollup supports 'use client' module directives then 'next-sanity' will include them and this re-export will no longer be necessary
export {PreviewSuspense as default} from 'next-sanity/preview'
app/page.tsx
:
import {previewData} from 'next/headers'
import PreviewSuspense from 'components/PreviewSuspense'
import {DocumentsCount, query} from 'components/DocumentsCount'
import PreviewDocumentsCount from 'components/PreviewDocumentsCount'
import {client} from 'lib/sanity.client'
type AppPreviewData = {token: string} | undefined
export default async function IndexPage() {
if ((previewData() as AppPreviewData)?.token) {
return (
<PreviewSuspense fallback="Loading...">
<PreviewDocumentsCount token={(previewData() as AppPreviewData).token} />
</PreviewSuspense>
)
}
const data = await client.fetch(query)
return <DocumentsCount data={data} />
}
The real-time preview isn't optimized and comes with a configured limit of 3000 documents. You can experiment with larger datasets by configuring the hook with documentLimit: <Integer>
. Be aware that this might significantly affect the preview performance.
You may use the includeTypes
option to reduce the amount of documents and reduce the risk of hitting the documentLimit
:
import {definePreview} from 'next-sanity/preview'
export const usePreview = definePreview({
projectId,
dataset,
documentLimit: 10000,
includeTypes: ['page', 'product', 'sanity.imageAsset'],
// If you have a lot of editors changing content at the same time it might help to increase this value
// to reduce the amount of rerenders React have to perform.
subscriptionThrottleMs: 300,
})
We have plans for optimizations in the roadmap.
next-sanity/studio
The latest version of Sanity Studio allows you to embed a near-infinitely configurable content editing interface into any React application. This opens up many possibilities:
NextStudio
loads up the import {Studio} from 'sanity'
component for you and wraps it in a Next-friendly layout. metadata
specifies the necessary <meta>
tags for making the Studio adapt to mobile devices, and prevents the route from being indexed by search engines.
Both the Next /app
and /pages
examples uses this config file:
sanity.config.ts
:
import {defineConfig} from 'sanity'
import {deskTool} from 'sanity/desk'
import {schemaTypes} from './schemas'
const projectId = process.env.NEXT_PUBLIC_SANITY_PROJECT_ID!
const dataset = process.env.NEXT_PUBLIC_SANITY_DATASET!
export default defineConfig({
basePath: '/studio', // <-- important that `basePath` matches the route you're mounting your studio from, it applies to both `/pages` and `/app`
projectId,
dataset,
plugins: [deskTool()],
schema: {
types: schemaTypes,
},
})
To use sanity.cli.ts
with the same projectId
and dataset
as your sanity.config.ts
:
/* eslint-disable no-process-env */
import {loadEnvConfig} from '@next/env'
import {defineCliConfig} from 'sanity/cli'
const dev = process.env.NODE_ENV !== 'production'
loadEnvConfig(__dirname, dev, {info: () => null, error: console.error})
const projectId = process.env.NEXT_PUBLIC_SANITY_PROJECT_ID
const dataset = process.env.NEXT_PUBLIC_SANITY_DATASET
export default defineCliConfig({api: {projectId, dataset}})
Now you can run commands like npx sanity cors add
. See npx sanity help
for a full list of what you can do.
/app
directory (experimental)We support the new appDir
mode in Next, but please note that appDir
shouldn't be used in production before Vercel says it's stable.
app/studio/[[...index]]/page.tsx
:
import {Studio} from './Studio'
// Set the right `viewport`, `robots` and `referer` meta tags
export {metadata} from 'next-sanity/studio/metadata'
export default function StudioPage() {
return <Studio />
}
app/studio/[[...index]]/Studio.tsx
:
'use client'
import {NextStudio} from 'next-sanity/studio'
import config from '../../../sanity.config'
export function Studio() {
// Supports the same props as `import {Studio} from 'sanity'`, `config` is required
return <NextStudio config={config} />
}
Customize meta tags
app/studio/[[...index]]/page.tsx
:
import type {Metadata} from 'next'
import {metadata as studioMetadata} from 'next-sanity/studio/metadata'
import {Studio} from './Studio'
// Set the right `viewport`, `robots` and `referer` meta tags
export const metadata: Metadata = {
...studioMetadata,
// Overrides the viewport to resize behavior
viewport: `${studioMetadata.viewport}, interactive-widget=resizes-content`,
}
export default function StudioPage() {
return <Studio />
}
/pages
directory/pages/studio/[[...index]].tsx
:
import Head from 'next/head'
import {NextStudio} from 'next-sanity/studio'
import {metadata} from 'next-sanity/studio/metadata'
import config from '../../sanity.config'
export default function StudioPage() {
return (
<>
<Head>
{Object.entries(metadata).map(([key, value]) => (
<meta key={key} name={key} content={value} />
))}
</Head>
<NextStudio config={config} />
</>
)
}
StudioProvider
and StudioLayout
If you want to go lower level and have more control over the studio you can pass StudioProvider
and StudioLayout
from sanity
as children
:
import {NextStudio} from 'next-sanity/studio'
import {StudioProvider, StudioLayout} from 'sanity'
import config from '../../../sanity.config'
function StudioPage() {
return (
<NextStudio config={config}>
<StudioProvider config={config}>
{/* Put components here and you'll have access to the same React hooks as Studio gives you when writing plugins */}
<StudioLayout />
</StudioProvider>
</NextStudio>
)
}
next-sanity/webhook
Implements @sanity/webhook
to parse and verify that a Webhook is indeed coming from Sanity infrastructure.
pages/api/revalidate
:
import type {NextApiRequest, NextApiResponse} from 'next'
import {parseBody} from 'next-sanity/webhook'
// Export the config from next-sanity to enable validating the request body signature properly
export {config} from 'next-sanity/webhook'
export default async function revalidate(req: NextApiRequest, res: NextApiResponse) {
try {
const {isValidSignature, body} = await parseBody(req, process.env.SANITY_REVALIDATE_SECRET)
if (!isValidSignature) {
const message = 'Invalid signature'
console.warn(message)
res.status(401).json({message})
return
}
const staleRoute = `/${body.slug.current}`
await res.revalidate(staleRoute)
const message = `Updated route: ${staleRoute}`
console.log(message)
return res.status(200).json({message})
} catch (err) {
console.error(err)
return res.status(500).json({message: err.message})
}
}
v2
The v3
release only contains breaking changes on the next-sanity/studio
imports. If you're only using import {createClient, groq} from 'next-sanity'
or import {definePreview, PreviewSuspense} from 'next-sanity/preview'
then there's no migration for you to do.
NextStudioGlobalStyle
is removedThe layout is no longer using global CSS to set the Studio height. The switch to local CSS helps interop between Next /pages
and /app
layouts.
ServerStyleSheetDocument
is removedIt's no longer necessary to setup styled-components
SSR for the Studio to render correctly.
isWorkspaceWithTheme
and isWorkspaces
utils are no longer exportedThe useTheme
hook is still available if you're building abstractions that need to know what the initial workspace theme variables are.
useBackgroundColorsFromTheme
, useBasePath
, useConfigWithBasePath
, and useTextFontFamilyFromTheme
, hooks are removedYou can useTheme
to replace useBackgroundColorsFromTheme
and useTextFontFamilyFromTheme
:
import {useMemo} from 'react'
import {useTheme} from 'next-sanity/studio'
import type {StudioProps} from 'sanity'
export default function MyComponent(props: Pick<StudioProps, 'config'>) {
const theme = useTheme(config)
// useBackgroundColorsFromTheme
const {themeColorLight, themeColorDark} = useMemo(
() => ({
themeColorLight: theme.color.light.default.base.bg,
themeColorDark: theme.color.dark.default.base.bg,
}),
[theme]
)
// useTextFontFamilyFromTheme
const fontFamily = useMemo(() => theme.fonts.text.family, [theme])
}
The reason why useBasePath
and useConfigWithBasePath
got removed is because Next /pages
and /app
diverge too much in how they declare dynamic segments. Thus you'll need to specify basePath
in your sanity.config.ts
manually to match the route you're loading the studio, for the time being.
NextStudioHead
component has moved from next-sanity/studio
to next-sanity/studio/head
Its props are also quite different and it now requires you to wrap it in import Head from 'next/head'
if you're not using a head.tsx
in appDir
. Make sure you use TypeScript to ease the migration.
v1
createPreviewSubscriptionHook
is replaced with definePreview
There are several differences between the hooks. First of all, definePreview
requires React 18 and Suspense. And as it's designed to work with React Server Components you provide token
in the hook itself instead of in the definePreview
step. Secondly, definePreview
encourages code-splitting using React.lazy
and that means you only call the usePreview
hook in a component that is lazy loaded. Quite different from usePreviewSubscription
which was designed to be used in both preview mode, and in production by providing initialData
.
The files that are imported here are the same as the Next /pages
example.
pages/index.tsx
import {createPreviewSubscriptionHook} from 'next-sanity'
import {DocumentsCount, query} from 'components/DocumentsCount'
import {client, projectId, dataset} from 'lib/sanity.client'
export const getStaticProps = async ({preview = false}) => {
const data = await client.fetch(query)
return {props: {preview, data}}
}
const usePreviewSubscription = createPreviewSubscriptionHook({projectId, dataset})
export default function IndexPage({preview, data: initialData}) {
const {data} = usePreviewSubscription(indexQuery, {initialData, enabled: preview})
return <DocumentsCount data={data} />
}
components/PreviewDocumentsCount.tsx
import {definePreview} from 'next-sanity/preview'
import {projectId, dataset} from 'lib/sanity.client'
const usePreview = definePreview({projectId, dataset})
export default function PreviewDocumentsCount() {
const data = usePreview(null, query)
return <DocumentsCount data={data} />
}
pages/index.tsx
import {lazy} from 'react'
import {PreviewSuspense} from 'next-sanity/preview'
import {DocumentsCount, query} from 'components/DocumentsCount'
import {client} from 'lib/sanity.client'
const PreviewDocumentsCount = lazy(() => import('components/PreviewDocumentsCount'))
export const getStaticProps = async ({preview = false}) => {
const data = await client.fetch(query)
return {props: {preview, data}}
}
export default function IndexPage({preview, data}) {
if (preview) {
return (
<PreviewSuspense fallback={<DocumentsCount data={data} />}>
<PreviewDocumentsCount />
</PreviewSuspense>
)
}
return <DocumentsCount data={data} />
}
createCurrentUserHook
is removedIf you used this hook to check if the user is cookie authenticated:
import {createCurrentUserHook} from 'next-sanity'
const projectId = process.env.NEXT_PUBLIC_SANITY_PROJECT_ID
const useCurrentUser = createCurrentUserHook({projectId})
const useCheckAuth = () => {
const {data, loading} = useCurrentUser()
return loading ? false : !!data
}
export default function Page() {
const isAuthenticated = useCheckAuth()
}
Then you can achieve the same functionality using @sanity/preview-kit
and suspend-react
:
import {suspend} from 'suspend-react'
import {_checkAuth} from '@sanity/preview-kit'
const projectId = process.env.NEXT_PUBLIC_SANITY_PROJECT_ID
const useCheckAuth = () =>
suspend(() => _checkAuth(projectId, null), ['@sanity/preview-kit', 'checkAuth', projectId])
export default function Page() {
const isAuthenticated = useCheckAuth()
}
v0.4
createPortableTextComponent
is removedThis utility used to wrap @sanity/block-content-to-react
. It's encouraged to upgrade to @portabletext/react
.
$ npm install @portabletext/react
// or
$ yarn add @portabletext/react
-import { createPortableTextComponent } from 'next-sanity'
+import { PortableText as PortableTextComponent } from '@portabletext/react'
-export const PortableText = createPortableTextComponent({ serializers: {} })
+export const PortableText = (props) => <PortableTextComponent components={{}} {...props} />
Please note that the serializers
and components
are not 100% equivalent.
Check the full migration guide.
createImageUrlBuilder
is removedThis utility is no longer wrapped by next-sanity
and you'll need to install the dependency yourself:
$ npm install @sanity/image-url
// or
$ yarn add @sanity/image-url
-import { createImageUrlBuilder } from 'next-sanity'
+import createImageUrlBuilder from '@sanity/image-url'
Run "CI & Release" workflow. Make sure to select the main branch and check "Release new version".
Semantic release will only release on configured branches, so it is safe to run release on any branch.
MIT-licensed. See LICENSE.
FAQs
Sanity.io toolkit for Next.js
The npm package next-sanity receives a total of 31,663 weekly downloads. As such, next-sanity popularity was classified as popular.
We found that next-sanity demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 63 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.