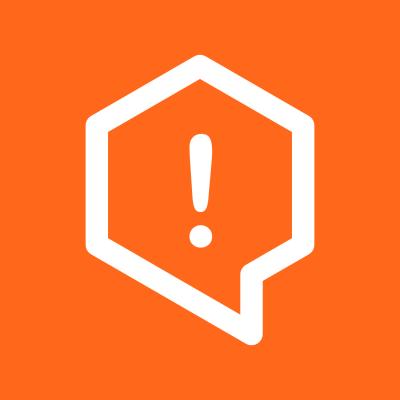
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
next-type-router
Advanced tools
🔬 An experiment to make next.js router usage safer.
Heavily inspired by my work on @swan-io/chicane
$ yarn add next-type-router
# --- or ---
$ npm install --save next-type-router
First, you have to generate the typed router functions. For that, I recommend using npm scripts:
"scripts": {
"type-router": "type-router src/router.ts",
"dev": "yarn type-router && next dev",
"build": "yarn type-router && next build",
When ran, this command parse your pages tree and generates a TS file (src/router.ts
) which looks like this:
import { createTypedFns } from "next-type-router";
export const {
createURL,
getApiRequestParams,
getServerSideParams,
useRouterWithSSR,
useRouterWithNoSSR,
} = createTypedFns([
// Here will be all your project routes:
"/",
"/api/auth/login",
"/api/projects/[projectId]",
"/projects",
"/projects/[projectId]",
"/users",
"/users/[userId]",
"/users/[userId]/favorites/[[...rest]]",
"/users/[userId]/repositories",
"/users/[userId]/repositories/[repositoryId]",
]);
import Link from "next/link";
import { createURL } from "path/to/router";
export default function ExamplePage() {
return (
<>
<h1>Users</h1>
{/* URL params are type safe! */}
<Link href={createURL("/users/[userId]", { userId: "zoontek" })}>
zoontek
</Link>
</>
);
}
import { useRouterWithSSR } from "path/to/router";
export default function ExamplePage() {
const { params } = useRouterWithSSR("/users/[userId]"); // we can use useRouterWithSSR since getServerSideProps is used
const { userId } = params.route; // userId type is string
return <h1>{userId} profile</h1>;
}
import { useRouterWithNoSSR } from "path/to/router";
export default function ExamplePage() {
const { params } = useRouterWithNoSSR("/users/[userId]"); // we have to use useRouterWithNoSSR since getServerSideProps is not used
const { userId } = params.route; // userId type is string | undefined
if (userId == null) {
return <span>Loading…</span>;
}
return <h1>{userId} profile</h1>;
}
import type { NextApiRequest, NextApiResponse } from "next";
import { getApiRequestParams } from "path/to/router";
export default function handler(req: NextApiRequest, res: NextApiResponse) {
// we can access params in a safe way on API routes
const params = getApiRequestParams("/api/projects/[projectId]", req);
res.status(200).json({ handler: "project", params });
}
import { GetServerSideProps } from "next";
import Link from "next/link";
import { getServerSideParams } from "path/to/router";
export const getServerSideProps: GetServerSideProps = async (context) => {
const params = getServerSideParams("/users/[userId]", context);
// we can access params in a safe way on the server
console.log(params.route.userId);
return { props: {} };
};
What happen when I, let's say, use useRouterWithSSR("/users/[userId]")
in page with /project/[projectId]
path?
Well, it will throw an error 💥. That's why I highly recommand to create a 500.tsx
page and wrap your app in an Error Boundary.
FAQs
An experiment to make next.js router usage safer.
The npm package next-type-router receives a total of 0 weekly downloads. As such, next-type-router popularity was classified as not popular.
We found that next-type-router demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.