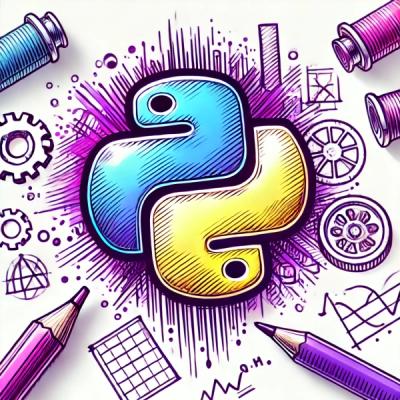
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
next-with-error
Advanced tools
Next.js HoC to render the Error page and send the correct HTTP status code from any page
Next.js plugin to render the Error page and send the correct HTTP status code from any page's getInitialProps
.
This higher-order-components allows you to easily return Next.js's Error page + the correct HTTP status code just by defining error.statusCode
in your pages getInitialProps
:
// pages/something.js
const SomePage = () => (
<h1>I will only render if error.statusCode is lesser than 400</h1>
);
SomePage.getInitialProps = async () => {
const isAuthenticated = await getUser();
if (!isAuthenticated) {
return {
error: {
statusCode: 401
}
};
}
return {
// ...
};
};
npm install next-with-error
withError([ErrorPage])(App)
Adapt pages/_app.js
so it looks similar to what is described in the official Next.js documentation and add the withError
HoC.
import React from 'react';
import App from 'next/app';
import withError from 'next-with-error';
class MyApp extends App {
static async getInitialProps(appContext) {
// calls page's `getInitialProps` and fills `appProps.pageProps`
const appProps = await App.getInitialProps(appContext);
return { ...appProps };
}
render() {
const { Component, pageProps } = this.props;
return <Component {...pageProps} />;
}
}
export default withError()(MyApp);
Then, in any of your pages, define error.statusCode
if needed in your page's getInitialProps
// pages/article.js
import React from 'react';
import fetchPost from '../util/fetch-post';
class ArticlePage extends React.Component {
static async getInitialProps() {
const article = await fetchPost();
if (!article) {
// No article found, let's display a "not found" page
// Will return a 404 status code + display the Error page
return {
error: {
statusCode: 404
}
};
}
// Otherwise, all good
return {
article
};
}
render() {
return (
<h1>{this.props.article.title}</h1>
// ...
);
}
}
export default HomePage;
generatePageError(statusCode[, additionalProps])
If you find the code to write the error object is a bit verbose, feel free to use the generatePageError
helper:
import { generatePageError } from 'next-with-error';
// ...
SomePage.getInitialProps = async () => {
const isAuthenticated = await getUser();
if (!isAuthenticated) {
return generatePageError(401);
}
return {};
};
You can use the additionalProps
argument to pass custom props to the Error component.
By default, withError
will display the default Next.js error page. If you need to display your own error page, you will need to pass it as the first parameter of your HoC:
import ErrorPage from './_error';
// ...
export default withError(ErrorPage)(MyApp);
Work to automate this is tracked here.
The error object properties are accessible via the props
of your custom Error component (props.statusCode
, props.message
, etc if you have custom props).
⚠️ If your custom Error page has a getInitialProps
method, the error object will be merged in getInitialProps
's return value. Be careful to not have conflicting names.
You can also pass custom props to your Error Page component by adding anything you would like in the error
object:
// /pages/article.js
const HomePage = () => <h1>Hello there!</h1>;
HomePage.getInitialProps = () => {
return {
error: {
statusCode: 401,
message: 'oopsie'
}
};
};
export default HomePage;
// /pages/_error.js
import React from 'react';
const Error = (props) => {
return (
<>
<h1>Custom error page: {props.error.statusCode}</h1>
<p>{props.error.message}</p>
</>
);
};
export default Error;
⚠️ Be careful to add default values for your custom props in the Error
component, as Next.js routing may bypass next-with-error
's behavior by showing the 404 page without the message
variable (in this example).
FAQs
Next.js HoC to render the Error page and send the correct HTTP status code from any page
The npm package next-with-error receives a total of 23 weekly downloads. As such, next-with-error popularity was classified as not popular.
We found that next-with-error demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.