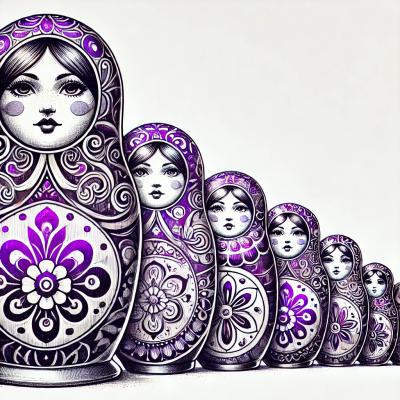
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
nforce is node.js a REST API wrapper for force.com, database.com, and salesforce.com.
$ npm install nforce
Require nforce in your app and create a connection to an org.
var nforce = require('nforce');
var org = nforce.createConnection({
clientId: 'SOME_OAUTH_CLIENT_ID',
clientSecret: 'SOME_OAUTH_CLIENT_SECRET',
redirectUri: 'http://localhost:3000/oauth/_callback',
apiVersion: 'v24.0', // optional, defaults to v24.0
environment: 'production' // optional, sandbox or production, production default
});
Now we just need to authenticate and get our OAuth credentials. Here is one way...
var oauth;
org.authenticate({ username: 'my_test@gmail.com', password: 'mypassword'}, function(err, resp){
if(!err) oauth = resp;
});
Now we can go nuts. Inserting a record...
var record = {
type: 'Account',
fieldValues: {
Name: 'Spiffy Cleaners',
Custom__c: 234
}
}
org.insert(record, oauth, function(err, resp){
if(!err) console.log('It worked!');
});
nforce supports two OAuth 2.0 flows, username/password and authorization code.
To request an access token and other oauth information using the username and password flow, use the authenticate()
method and pass in your username and password in the options
var oauth;
org.authenticate({ username: 'my_test@gmail.com', password: 'mypassword'}, function(err, resp){
if(!err) {
console.log('Access Token: ' + resp.access_token);
oauth = resp;
} else {
console.log('Error: ' + err.message);
}
});
To perform an authorization code flow, first redirect users to the Authorization URI. nforce provides a helper function to build this url for you.
org.getAuthUri()
Once you get a callback at the Redirect URI that you specify, you need to request your access token and other important oauth information by calling authenticate()
and passing in the "code" that you received.
var oauth;
org.authenticate({ code: 'SOMEOAUTHAUTHORIZATIONCODE' }, function(err, resp){
if(!err) {
console.log('Access Token: ' + resp.access_token);
oauth = resp;
} else {
console.log('Error: ' + err.message);
}
});
At the end of a successful authorization, you a returned an OAuth object for the user. Cache this object as it will be used for subsequent requests. This object contains your access token, endpoint, id, and other information.
nforce has built-in support for express using the express/connect middleware system. The middleware handles the oauth callbacks for you. To use the middleware you must have sessions enabled in your express configuration.
app.configure(function(){
app.set('views', __dirname + '/views');
app.set('view engine', 'jade');
app.use(express.bodyParser());
app.use(express.methodOverride());
app.use(express.cookieParser());
app.use(express.session({ secret: 'nforce testing baby' }));
app.use(org.expressOAuth({onSuccess: '/home', onError: '/oauth/error'})); // <--- nforce middleware
app.use(app.router);
app.use(express.static(__dirname + '/public'));
});
Callbacks will always pass an optional error object, and a response object.
callback(err, resp);
The createConnection method creates an nforce connection object. You need to supply some arguments including oauth information and some optional arguments for version and environment.
clientId
: Required. This is the OAuth client idclientSecret
: Required. This is the OAuth client secretredirectUri
: Required. This is the redirect URI for OAuth callbacksapiVersion
: Optional. This is a number or string representing a valid REST API version. Default is v24.0.environment
: Optional. Values can be 'production' or 'sandbox'. Default is production.The following list of methods are available for an nforce connection object:
This is a helper method to build the authentication uri for a authorization code OAuth 2.0 flow.
This method requests the OAuth access token and instance information from Salesforce. This method either requires that you pass in the authorization code (authorization code flow) or username and password (username/password flow).
code
: (String) An OAuth authorization code-- OR --
username
: (String) Your salesforce/force.com/database.com usernamepassword
: (String) Your salesforce/force.com/database.com passwordGets the salesforce versions. Note: Does not require authentication.
Gets the available resources
Get all sObjects for an org
Get metadata for a single sObject. type
is a required String for the sObject type
Get describe information for a single sObject. type
is a required String for the sObject type
Insert a record. data
is an object with the following properties:
type
: (String) sObject typefieldValues
: (Object) contains field names and valuesUpdate a record. data
is an object with the following properties:
type
: (String) sObject typeid
: (String) the id of the recordfieldValues
: (Object) contains field names and valuesUpdate a record. data
is an object with the following properties:
type
: (String) sObject typeexternalId
: (String) the external id of the recordexternalIdField
: (String) the external id field to be used for upsertingfieldValues
: (Object) contains field names and valuesDelete a record. data
is an object with the following properties:
type
: (String) sObject typeid
: (String) the id of the recordGet a single record. data
is an object with the following properties:
type
: (String) sObject typeid
: (String) the id of the recordExecute a SOQL query for records. query
should be a SOQL string.
Execute a SOSL search for records. search
should be a SOSL string.
Get a REST API resource by its url. url
should be a REST API resource.
v0.0.1
: Initial release. OAuth, CRUD, describesFAQs
nforce is a REST API wrapper for force.com, database.com, and salesforce.com
The npm package nforce receives a total of 5,852 weekly downloads. As such, nforce popularity was classified as popular.
We found that nforce demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.