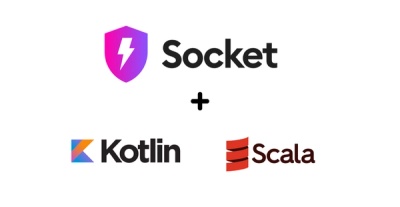
Product
Introducing Scala and Kotlin Support in Socket
Socket now supports Scala and Kotlin, bringing AI-powered threat detection to JVM projects with easy manifest generation and fast, accurate scans.
An Angular testing library for creating mock services, components, directives, pipes and modules in unit tests. It provides shallow rendering, precise stubs to fake child dependencies. ng-mocks works with Angular 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
ng-mocks is a powerful library for Angular that simplifies the process of creating mock components, directives, pipes, and services for unit testing. It helps developers to isolate the unit of work and test it without dependencies on other parts of the application.
Mock Components
This feature allows you to create mock versions of Angular components. This is useful for isolating the component under test from its child components.
import { MockComponent } from 'ng-mocks';
import { MyComponent } from './my-component';
TestBed.configureTestingModule({
declarations: [MockComponent(MyComponent)]
});
Mock Directives
This feature allows you to create mock versions of Angular directives. This is useful for isolating the component under test from its directives.
import { MockDirective } from 'ng-mocks';
import { MyDirective } from './my-directive';
TestBed.configureTestingModule({
declarations: [MockDirective(MyDirective)]
});
Mock Pipes
This feature allows you to create mock versions of Angular pipes. This is useful for isolating the component under test from its pipes.
import { MockPipe } from 'ng-mocks';
import { MyPipe } from './my-pipe';
TestBed.configureTestingModule({
declarations: [MockPipe(MyPipe)]
});
Mock Services
This feature allows you to create mock versions of Angular services. This is useful for isolating the component under test from its services.
import { MockProvider } from 'ng-mocks';
import { MyService } from './my-service';
TestBed.configureTestingModule({
providers: [MockProvider(MyService)]
});
Jest is a delightful JavaScript testing framework with a focus on simplicity. It provides a powerful mocking library that can be used to mock functions, modules, and timers. While Jest is not specific to Angular, it can be used in conjunction with Angular to achieve similar mocking capabilities.
Sinon is a standalone test spies, stubs, and mocks library for JavaScript. It works with any unit testing framework and provides powerful mocking capabilities. Like Jest, Sinon is not specific to Angular but can be used to mock dependencies in Angular applications.
ts-mockito is a mocking library for TypeScript inspired by the Java library Mockito. It provides a simple API for creating mock objects and verifying interactions. ts-mockito is not specific to Angular but can be used to mock dependencies in Angular applications.
ng-mocks
facilitates Angular testing and helps to:
The current version of the library has been tested and can be used with:
angular | ng-mocks | jasmine | jest | ivy | standalone | signals | defer |
---|---|---|---|---|---|---|---|
20 | latest | yes | yes | yes | yes | no | no |
19 | latest | yes | yes | yes | yes | no | no |
18 | latest | yes | yes | yes | yes | no | no |
17 | latest | yes | yes | yes | yes | no | no |
16 | latest | yes | yes | yes | yes | no | |
15 | latest | yes | yes | yes | yes | ||
14 | latest | yes | yes | yes | yes | ||
13 | latest | yes | yes | yes | |||
12 | latest | yes | yes | yes | |||
11 | latest | yes | yes | yes | |||
10 | latest | yes | yes | yes | |||
9 | latest | yes | yes | yes | |||
8 | latest | yes | yes | ||||
7 | latest | yes | yes | ||||
6 | latest | yes | yes | ||||
5 | latest | yes | yes |
Global configuration for mocks in src/test.ts
.
In case of jest, src/setup-jest.ts
/ src/test-setup.ts
should be used.
// All methods in mock declarations and providers
// will be automatically spied on their creation.
// https://ng-mocks.sudo.eu/extra/auto-spy
ngMocks.autoSpy('jasmine'); // or jest
// ngMocks.defaultMock helps to customize mocks
// globally. Therefore, we can avoid copy-pasting
// among tests.
// https://ng-mocks.sudo.eu/api/ngMocks/defaultMock
ngMocks.defaultMock(AuthService, () => ({
isLoggedIn$: EMPTY,
currentUser$: EMPTY,
}));
An example of a spec for a profile edit component.
// Let's imagine that there is a ProfileComponent
// and it has 3 text fields: email, firstName,
// lastName, and a user can edit them.
// In the following test suite, we would like to
// cover behavior of the component.
describe('profile:builder', () => {
// Helps to reset customizations after each test.
// Alternatively, you can enable
// automatic resetting in test.ts.
MockInstance.scope();
// Let's configure TestBed via MockBuilder.
// The code below says to mock everything in
// ProfileModule except ProfileComponent and
// ReactiveFormsModule.
beforeEach(() => {
// The result of MockBuilder should be returned.
// https://ng-mocks.sudo.eu/api/MockBuilder
return MockBuilder(
ProfileComponent,
ProfileModule,
).keep(ReactiveFormsModule);
// // or old fashion way
// return TestBed.configureTestingModule({
// imports: [
// MockModule(SharedModule), // mock
// ReactiveFormsModule, // real
// ],
// declarations: [
// ProfileComponent, // real
// MockPipe(CurrencyPipe), // mock
// MockDirective(HoverDirective), // mock
// ],
// providers: [
// MockProvider(AuthService), // mock
// ],
// }).compileComponents();
});
// A test to ensure that ProfileComponent
// can be created.
it('should be created', () => {
// MockRender is an advanced version of
// TestBed.createComponent.
// It respects all lifecycle hooks,
// onPush change detection, and creates a
// wrapper component with a template like
// <app-root ...allInputs></profile>
// and renders it.
// It also respects all lifecycle hooks.
// https://ng-mocks.sudo.eu/api/MockRender
const fixture = MockRender(ProfileComponent);
expect(
fixture.point.componentInstance,
).toEqual(assertion.any(ProfileComponent));
});
// A test to ensure that the component listens
// on ctrl+s hotkey.
it('saves on ctrl+s hot key', () => {
// A fake profile.
const profile = {
email: 'test2@email.com',
firstName: 'testFirst2',
lastName: 'testLast2',
};
// A spy to track save calls.
// MockInstance helps to configure mock
// providers, declarations and modules
// before their initialization and usage.
// https://ng-mocks.sudo.eu/api/MockInstance
const spySave = MockInstance(
StorageService,
'save',
jasmine.createSpy(), // or jest.fn()
);
// Renders <profile [profile]="params.profile">
// </profile>.
// https://ng-mocks.sudo.eu/api/MockRender
const { point } = MockRender(
ProfileComponent,
{ profile }, // bindings
);
// Let's change the value of the form control
// for email addresses with a random value.
// ngMocks.change finds a related control
// value accessor and updates it properly.
// https://ng-mocks.sudo.eu/api/ngMocks/change
ngMocks.change(
'[name=email]', // css selector
'test3@em.ail', // an email address
);
// Let's ensure that nothing has been called.
expect(spySave).not.toHaveBeenCalled();
// Let's assume that there is a host listener
// for a keyboard combination of ctrl+s,
// and we want to trigger it.
// ngMocks.trigger helps to emit events via
// simple interface.
// https://ng-mocks.sudo.eu/api/ngMocks/trigger
ngMocks.trigger(point, 'keyup.control.s');
// The spy should be called with the user
// and the random email address.
expect(spySave).toHaveBeenCalledWith({
email: 'test3@em.ail',
firstName: profile.firstName,
lastName: profile.lastName,
});
});
});
Profit.
If you like ng-mocks
, please support it:
Thank you!
P.S. Feel free to contact us if you need help.
FAQs
An Angular testing library for creating mock services, components, directives, pipes and modules in unit tests. It provides shallow rendering, precise stubs to fake child dependencies. ng-mocks works with Angular 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
The npm package ng-mocks receives a total of 348,647 weekly downloads. As such, ng-mocks popularity was classified as popular.
We found that ng-mocks demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports Scala and Kotlin, bringing AI-powered threat detection to JVM projects with easy manifest generation and fast, accurate scans.
Application Security
/Security News
Socket CEO Feross Aboukhadijeh and a16z partner Joel de la Garza discuss vibe coding, AI-driven software development, and how the rise of LLMs, despite their risks, still points toward a more secure and innovative future.
Research
/Security News
Threat actors hijacked Toptal’s GitHub org, publishing npm packages with malicious payloads that steal tokens and attempt to wipe victim systems.