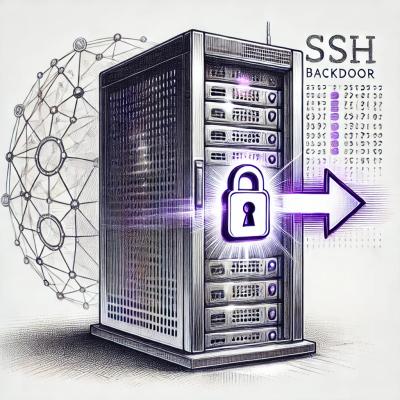
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
ng2-progressbar
Advanced tools
This library is designed to make it easy for your Angular application to request specific resources from a WordPress install.
Wordpress installation and WP API v2 plugin activated.
Install it with npm
npm install ng2-wp-api --save
This library is very flexible and easy to use, you will find everything you need included out of the box:
WpService
One service for all operations (Access/Authenticate/Configure).<wp-collection>
Inputs: args, endpoint - Output: collection response.<wp-model>
Inputs: id, args, endpoint - Output: model response.WpPost
Useful class for posts and pages (contains functions for accessing embedded posts).WpUser
Interface for user response.WpQueryArgs
Class for creating query arguments for collection/model.WpEndpoint
List of default endpoints and their addresses.Default Endpoints are : posts
, pages
, users
, categories
, tags
, taxonomies
, statuses
, comments
, media
WpService
├── config
| ├── baseUrl ** Set WordPress URL
| ├── timeOut ** Http requests timeout
| ├── loading ** Listener for requests loading state
| ├── errors ** Listener for requests errors
|
├── discover(url) ** Discover if a URL has a valid API
|
├── link(url) ** Http Getter with the benefits of loading and errors observables.
| Useful for getting data from external resources
|
├── collection()
| ├── endpoint(ep)
| ├── get(args?) ** Get Collection of endpoint type.
| ├── more() ** Get Next page collection combined with any previous ones.
| ├── next() ** Get Next page collection.
| ├── prev() ** Get Prev page collection.
|
├── model()
| ├── endpoint(ep)
| ├── get(id, args?) ** Get Model by Id.
| ├── add(body) ** Add Model to WP.
| ├── update(id, body) ** Update Model by Id.
| ├── delete(id) ** Delete Model by Id.
|
├── auth()
| ├── basic(username, password) ** Basic authentication, returns loggedInUser.
| ├── cookies() ** Cookies authentication, returns loggedInUser.
| ├── logout() ** Removes authentication info from all requests.
## Usage
Add WordPressModule
to NgModule imports
array.
import { WordPressModule } from 'ng2-wp-api';
@NgModule({
imports: [
WordPressModule
]
})
### Initialization
Set your WordPress base url in the root component
constructor(wpService: WpService){
}
ngOnInit(){
wpService.config.baseUrl = "http://localhost/wordpress";
/** Optional */
//catch loading state (useful for spinner)
wpService.config.loading.subscribe(...);
//catch any errors occurs in all requests
wpService.config.errors.subscribe(...);
}
See [Initializing example](/examples/Initilizing WP Service.ts).
After initializing, you can either consume the library as a service, or as a component.
Import WpService
in component constructor
Import WpEndpoint
to get the desired endpoint address
import {WpService} from "ng2-wp-api";
@Component({...})
export class testComponent {
constructor(private wpService: WpService) {
}
}
**For collection:**
A basic example of fetching 6 embedded posts:
import {WpService,WpEndpoint,WpQueryArgs,CollectionResponse} from "ng2-wp-api";
.
.
var endpoint = WpEndpoint.posts;
var args = new WpQueryArgs({
perPage: 6,
embed: true
});
this.wpService.collection()
.endpoint(endpoint) //or posts()
.get(args)
.subscribe((res: CollectionResponse) => {
if(res.error){
console.log(res.error);
}
else{
this.data = res.data;
this.pagination = res.pagination;
}
});
See [WpService Collection example](/examples/Collection using the service.ts)
**For model:**var endpoint = WpEndpoint.posts;
this.wpService.model()
.endpoint(endpoint) //or posts() or pages() or users() ..etc
.get(id)
.subscribe((res: ModelResponse) => {
if(res.error){
console.log(res.error);
}
else{
this.data = res.data;
}
});
See [WpService Model example](/examples/Model using the service.ts)
<wp-collection [args]="args" [endpoint]="endpoint" (response)="wpResponse($event)">
<!-- Your Template Goes Here -->
</wp-collection>
WpCollection component gets a new response automatically when the input [args]
value has set/changed.
See [Collection Component example](/examples/Collection using the component.ts)
**For model:**NOTE: Make sure
enableProdMode()
is called to avoidonChanges
error.
<wp-model [id]="id" [endpoint]="endpoint" (response)="wpResponse($event)">
<!-- Your Template Goes Here -->
<wp-model>
WpModel component gets a new response automatically when the input [id]
value has set/changed.
See [Model Component example](/examples/Model using the component.ts)
If you want to do a GET
request for any URL, Use WpService.link(url).subscribe(...)
to get the advantage of error and loading notifiers.
//add new post
wpService.model().posts().add(body);
//update page by id
wpService.model().pages().update(pageId, body);
//delete user by id
wpService.model().users().delete(userId);
this.wpService.auth().basic('username', 'password').subscribe(
(loggedInUser: WpUser)=> {
console.log(loggedInUser);
});
this.wpService.auth().cookies().subscribe(
(loggedInUser: WpUser)=> {
console.log(loggedInUser);
});
## Embedded Responses
Usually when displaying a post, you want to display post's featured image, categories, tags, author and comments. The normal post response contains only the Id references which you will have to request each one of them individually.
Embedded responses are very useful to reduce the amount of http requets. you will get all the information you need in one response.
Embedding is triggered by setting the _embed=true
in WpQueryArgs, check Linking and Embedding
And now WpPost
class will be useful to access the following properties:
post ** the original object
id() ** post id
title() ** post title
content() ** post content
excerpt() ** post excerpt without the (read more) link
date() ** post date
type() ** post type
categories() ** post categories array
tags() ** post tags array
author() ** post author object (WpUser)
featuredMedia() ** to check if a post has a featured image
featuredImageUrl(size) ** to get featured image by the size, ("full", large", "medium") or
any other valid size you have in your WP
other properties can be accessed from the original post
object.
var wpPost = new WpPost(originalPost);
wpPost.post.propertyName
where wpPost.post
= originalPost
, See WpPost class source code
WpEndpoint
to get the default endpoints and their addresses.WpService.collection.posts().get(...)
is a shortcut of WpService.Collection.endpoint(WpEndpoint.posts)
WpQueryArgs
class to specify your request argument.WpPost
class when the response is embedded, it has useful functions for accessing embedded posts.WpPost
class works for posts, pages and custom post types.WpUser
interface for user response.If you identify any errors in the library, or have an idea for an improvement, please open an issue. I am excited to see what the community thinks of this project, and I would love your input!
## Author ## LicenseFAQs
A slim customizable progressbar for angular2
The npm package ng2-progressbar receives a total of 65 weekly downloads. As such, ng2-progressbar popularity was classified as not popular.
We found that ng2-progressbar demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.