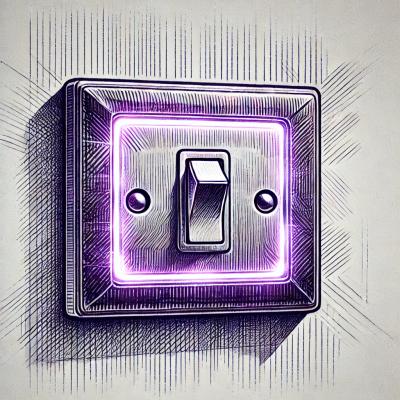
Research
Security News
Kill Switch Hidden in npm Packages Typosquatting Chalk and Chokidar
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
ng2-redux
Advanced tools
Angular 2 bindings for Redux.
For Angular 1 see ng-redux
Ng2Redux lets you easily connect your Angular 2 components with Redux, while still respecting the Angular 2 idiom.
Features include:
Observables
@select
decoratorIn addition, we are committed to providing insight on clean strategies for integrating with Angular 2's change detection and other framework features.
Ng2Redux has a peer dependency on redux, so we need to install it as well.
npm install --save redux ng2-redux
import { platformBrowserDynamic } from '@angular/platform-browser-dynamic';
import { AppModule } from './containers/app.module';
platformBrowserDynamic().bootstrapModule(AppModule);
Import the NgReduxModule
class and add it to your application module as an
import
. Once you've done this, you'll be able to inject NgRedux
into your
Angular 2 components. In your top-level app module, you
can configure your Redux store with reducers, initial state,
and optionally middlewares and enhancers as you would in Redux directly.
import { NgReduxModule, NgRedux } from 'ng2-redux';
import reduxLogger from 'redux-logger';
import { rootReducer } from './reducers';
interface IAppState { /* ... */ };
@NgModule({
/* ... */
imports: [ /* ... */, NgReduxModule ]
})
export class AppModule {
constructor(ngRedux: NgRedux<IAppState>) {
ngRedux.configureStore(rootReducer, {}, [ createLogger() ]);
}
}
Or if you prefer to create the Redux store yourself you can do that and use the
provideStore()
function instead:
import {
applyMiddleware,
Store,
combineReducers,
compose,
createStore
} from 'redux';
import { NgReduxModule, NgRedux } from 'ng2-redux';
import reduxLogger from 'redux-logger';
import { rootReducer } from './reducers';
interface IAppState { /* ... */ };
export const store: Store<IAppState> = createStore(
rootReducer,
compose(applyMiddleware(reduxLogger)));
@NgModule({
/* ... */
imports: [ /* ... */, NgReduxModule ]
})
class AppModule {
constructor(ngRedux: NgRedux<IAppState>) {
ngRedux.provideStore(store);
}
}
Now your Angular 2 app has been reduxified! Use the @select
decorator to
access your store state, and .dispatch()
to dispatch actions:
import { select } from 'ng2-redux';
@Component({
template: '<button (click)="onClick()">Clicked {{ count | async }} times</button>'
})
class App {
@select() count$: Observable<number>;
constructor(private ngRedux: NgRedux<IAppState>) {}
onClick() {
this.ngRedux.dispatch({ type: INCREMENT });
}
}
Here are some examples of Ng2Redux in action:
Ng2Redux uses an approach to redux based on RxJS Observables to select
and transform
data on its way out of the store and into your UI or side-effect handlers. Observables
are an efficient analogue to reselect
for the RxJS-heavy Angular world.
Read more here: Select Pattern
We also have a number of 'cookbooks' for specific Angular 2 topics:
FAQs
Angular 2 bindings for Redux
The npm package ng2-redux receives a total of 73 weekly downloads. As such, ng2-redux popularity was classified as not popular.
We found that ng2-redux demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.