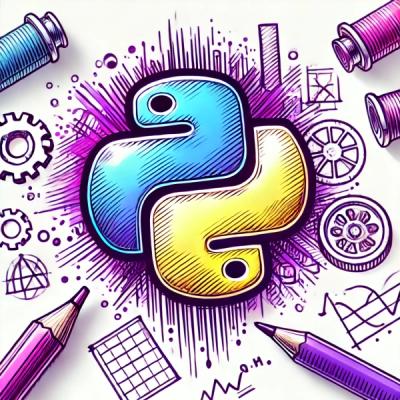
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
ng2-validators
Advanced tools
An implementation of various angular validators for Angular 2+.
npm install ng2-validators --save-dev
No config needed
Add following to project.config.ts
let additionalPackages: ExtendPackages[] = [
{
name: 'google-libphonenumber',
path: 'node_modules/google-libphonenumber/dist/libphonenumber.js'
},
{
name: 'ng2-validators',
path: 'node_modules/ng2-validators/bundles/ng2-validators.umd.min.js'
},
];
this.addPackagesBundles(additionalPackages);
The rules are from https://github.com/vt-middleware/passay
The password validators are:
npm install ng2-validators --save
needs: ReactiveFormsModule
import {PasswordValidators} from 'ng2-validators'
...
password: FormControl = new FormControl('', Validators.compose([
PasswordValidators.repeatCharacterRegexRule(4),
PasswordValidators.alphabeticalCharacterRule(1),
PasswordValidators.digitCharacterRule(1),
PasswordValidators.lowercaseCharacterRule(1),
PasswordValidators.uppercaseCharacterRule(1),
PasswordValidators.specialCharacterRule(1),
PasswordValidators.allowedCharacterRule(['a', 'b'])
]));
import {PasswordValidators} from 'ng2-validators'
...
let password: FormControl = new FormControl('testPassword');
let confirmPassword: FormControl = new FormControl('testPassword');
let form = new FormGroup({
'newPassword': password,
'confirmPassword': confirmPassword
}, PasswordValidators.mismatchedPasswords()
);
import {PasswordValidators} from 'ng2-validators'
...
let password: FormControl = new FormControl('testPassword');
let confirmPassword: FormControl = new FormControl('testPassword');
let form = new FormGroup({
'testName': password,
'testName2': confirmPassword
}, PasswordValidators.mismatchedPasswords('testName', 'testName2' )
);
import {EmailValidators} from 'ng2-validators'
...
email: FormControl = new FormControl('', EmailValidators.normal);
email2: FormControl = new FormControl('', EmailValidators.simple);
import {UniversalValidators} from 'ng2-validators'
...
control: FormControl = new FormControl('', UniversalValidators.noWhitespace);
control: FormControl = new FormControl('', UniversalValidators.isNumber);
control: FormControl = new FormControl('', UniversalValidators.isInRange(2, 5));
control: FormControl = new FormControl('', UniversalValidators.minLength(2));
control: FormControl = new FormControl('', UniversalValidators.maxLength(7));
control: FormControl = new FormControl('', UniversalValidators.min(2));
control: FormControl = new FormControl('', UniversalValidators.max(2));
import {CreditCardValidators} from 'ng2-validators'
...
control: FormControl = new FormControl('', UniversalValidators.isCreditCard);
control: FormControl = new FormControl('', UniversalValidators.americanExpress);
control: FormControl = new FormControl('', UniversalValidators.dinersclub);
control: FormControl = new FormControl('', UniversalValidators.discover);
control: FormControl = new FormControl('', UniversalValidators.jcb);
control: FormControl = new FormControl('', UniversalValidators.maestro);
control: FormControl = new FormControl('', UniversalValidators.mastercard);
control: FormControl = new FormControl('', UniversalValidators.visa);
import {PhoneValidators} from 'ng2-validators'
...
countryCode: FormControl = new FormControl('', PhoneValidators.isValidRegionCode);
phone: FormControl = new FormControl('', PhoneValidators.isPhoneNumber('US'));
phone2: FormControl = new FormControl('', PhoneValidators.isPossibleNumberWithReason('US'));
needs FormsModule and ValidatorsModule
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { ValidatorsModule } from 'ng2-validators'
import { AppComponent } from './app.component';
@NgModule({
imports: [BrowserModule, FormsModule, ValidatorsModule],
declarations: [AppComponent],
bootstrap: [AppComponent]
})
export class AppModule {
}
<form>
<input type="password" [(ngModel)]="model.password" name="password" #formControl="ngModel" password>
<span *ngIf="formControl.hasError('repeatCharacterRegexRule')">Password contains repeating characters</span>
<span *ngIf="formControl.hasError('digitCharacterRule')">Password should contain at least on digit</span>
<span *ngIf="formControl.hasError('alphabeticalCharacterRule')">Password should contain at least on alphabetical character</span>
<span *ngIf="formControl.hasError('lowercaseCharacterRule')">Password should contain at least on lowercase character</span>
<span *ngIf="formControl.hasError('uppercaseCharacterRule')">Password should contain at least on uppercase character</span>
</form>
// Override values
<input type="password" [(ngModel)]="model.password" name="password" #formControl="ngModel"
password
[repeatCharacter]="2"
[alphabeticalCharacter]="2"
[digitCharacter]="2"
[lowercaseCharacter]="2"
[uppercaseCharacter]="2"
>
<form>
<input type="text" [(ngModel)]="model.creditcard" name="creditcard" #formControl="ngModel" creditCard>
<span *ngIf="formControl.hasError('creditcard')">Is not a creditcard</span>
</form>
// Override values
// Test only for a specific creditcard
<input type="text" [(ngModel)]="model.creditcard" name="creditcard" #formControl="ngModel" [creditCard]="all|americanExpress|dinersclub|discover|jcb|maestro|mastercard|visa">
<form>
<input type="text" [(ngModel)]="model.email" name="email" #formControl="ngModel" email>
<span *ngIf="formControl.hasError('normalEmailRule')">Is not a email</span>
</form>
<form>
<input type="text" [(ngModel)]="model.phone" name="phone" #formControl="ngModel" phone="US">
<span *ngIf="formControl.hasError('noValidRegionCode')">Is not a countryCode</span>
<span *ngIf="formControl.hasError('noPhoneNumber')">Is not a phone number</span>
</form>
<form>
<input type="text" [(ngModel)]="model.phone" name="phone" #formControl="ngModel" possiblePhone="US">
<span *ngIf="formControl.hasError('noValidRegionCode')">Is not a countryCode</span>
<span *ngIf="formControl.hasError('phoneNumberTooLong')">Phone number is to long</span>
<span *ngIf="formControl.hasError('phoneNumberTooShort')">Phone number is to short</span>
<span *ngIf="formControl.hasError('noPhoneNumber')">Is not a phone number</span>
</form>
<form>
<input type="text" [(ngModel)]="model.countryCode" name="countryCode" #formControl="ngModel" countryCode>
<span *ngIf="formControl.hasError('noValidRegionCode')">Is not a countryCode</span>
</form>
<form>
<input type="text" [(ngModel)]="model.firstname" name="firstname" #formControl="ngModel" noWhitespace>
<span *ngIf="formControl.hasError('noWhitespaceRequired')">Should not contain a whitespace</span>
</form>
<form>
<input type="number" [(ngModel)]="model.amount" name="amount" #formControl="ngModel" isNumber>
<span *ngIf="formControl.hasError('numberRequired')">Needs to be a number</span>
</form>
<form>
<input type="number" [(ngModel)]="model.amount" name="amount" #formControl="ngModel" isInRange [minValue]="2" [maxValue]="4">
<span *ngIf="formControl.hasError('numberRequired')">Needs to be a number</span>
<span *ngIf="formControl.hasError('rangeValueToSmall')">Number to small</span>
<span *ngIf="formControl.hasError('rangeValueToBig')">Number to big</span>
</form>
<form>
<input type="number" [(ngModel)]="model.amount" name="amount" #formControl="ngModel" [min]="2">
<span *ngIf="formControl.hasError('numberRequired')">Needs to be a number</span>
<span *ngIf="formControl.hasError('min')">Number to small</span>
</form>
<form>
<input type="number" [(ngModel)]="model.amount" name="amount" #formControl="ngModel" [max]="2">
<span *ngIf="formControl.hasError('numberRequired')">Needs to be a number</span>
<span *ngIf="formControl.hasError('max')">Number to small</span>
</form>
##Todo
Get the complete changelog here: https://github.com/Nightapes/ng2-validators/releases
FAQs
An implementation of angular validators for Angular 2
We found that ng2-validators demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.