A library for loading and playing audio using HTML 5 for Angular 7/8/9/10/11/12.
(https://mudigal-technologies.github.io/ngx-audio-player/)

Table of contents
Demo
A simple, clean, responsive player for playing multiple audios with playlist support.
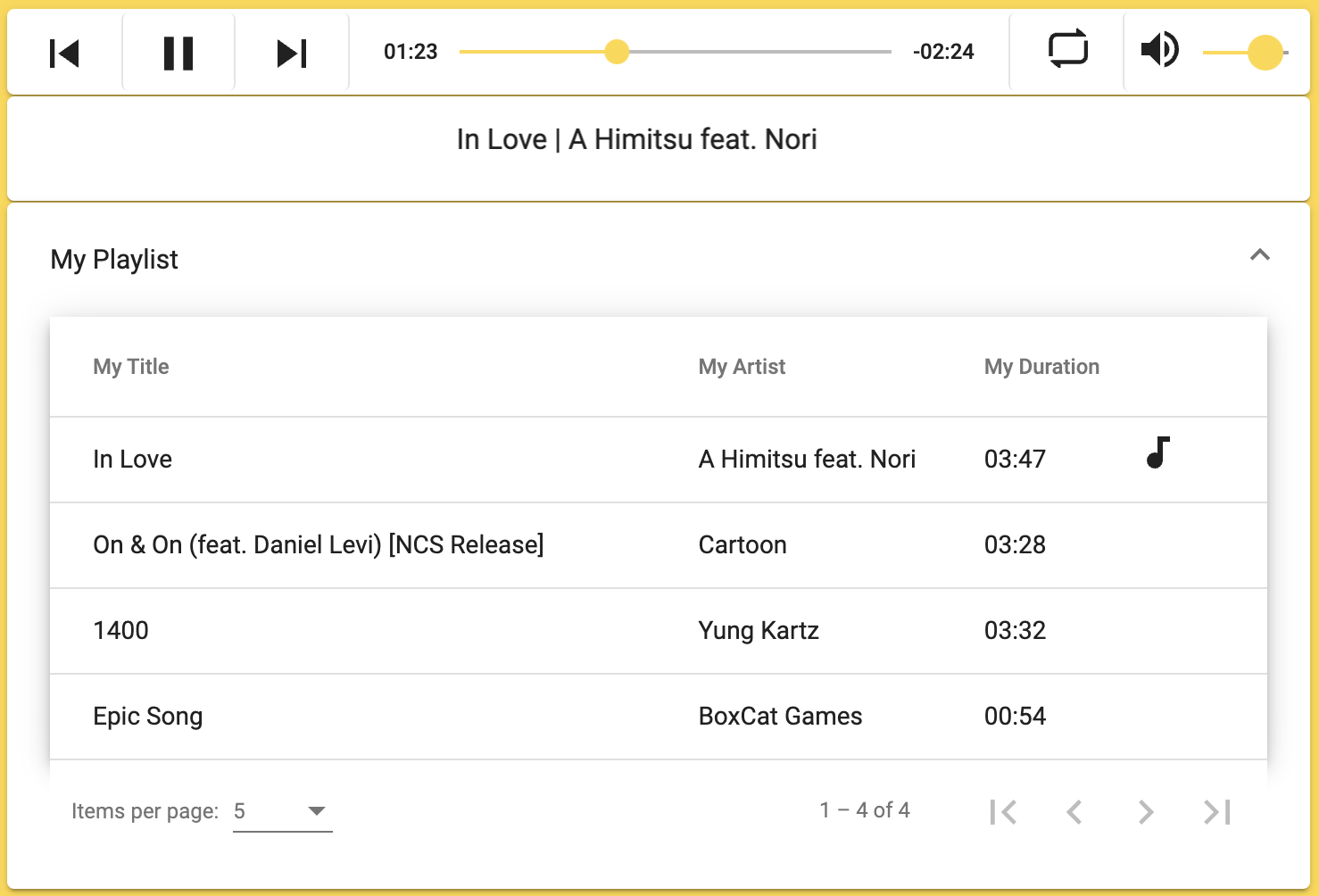
Working Demo
Installation
ngx-audio-player
is available via npm and yarn
Using npm:
$ npm install ngx-audio-player --save
Using yarn:
$ yarn add ngx-audio-player
Getting Started
NgxAudioPlayerModule needs Angular Material.
Make sure you have installed below dependencies with same or higher version than mentioned.
"@angular/core": "^12.0.0"
"@angular/common": "^12.0.0"
"@angular/material": "^12.0.0"
"rxjs": "^6.6.0"
Import NgxAudioPlayerModule
in the root module(AppModule
):
import { NgxAudioPlayerModule } from 'ngx-audio-player';
@NgModule({
imports: [
NgxAudioPlayerModule
]
})
export class AppModule { }
Usage
Simple Audio Player
HTML
<ngx-audio-player [autoPlay]="false" muted="muted"
[playlist]="mssapPlaylist"
[disablePositionSlider]="mssapDisablePositionSlider"
[displayRepeatControls]="mssapDisplayRepeatControls"
[displayVolumeControls]="mssapDisplayVolumeControls"
[displayVolumeSlider]="mssapDisplayVolumeSlider"
[displayTitle]="mssapDisplayTitle"
(trackEnded)="onEnded($event)">
</ngx-audio-player>
TS
import { Track } from 'ngx-audio-player';
.
.
mssapDisplayTitle = true;
mssapDisablePositionSlider = true;
mssapDisplayRepeatControls = true;
mssapDisplayVolumeControls = true;
mssapDisplayVolumeSlider = false;
mssapPlaylist: Track[] = [
{
title: 'Audio Title',
link: 'Link to Audio URL',
artist: 'Audio Artist',
duration: 'Audio Duration in seconds'
}
];
mssapPlaylist: Track[] = [
{
title: 'Audio Title',
link: 'Link to Streaming URL',
mediaType: 'stream'
}
];
Advanced Audio Player
HTML
<ngx-audio-player [autoPlay]="false" muted="muted"
[playlist]="msaapPlaylist"
[disablePositionSlider]="msaapDisablePositionSlider"
[displayRepeatControls]="msaapDisplayRepeatControls"
[displayVolumeControls]="msaapDisplayVolumeControls"
[displayVolumeSlider]="msaapDisplayVolumeSlider"
[displayTitle]="msaapDisplayTitle"
[displayPlaylist]="msaapDisplayPlayList"
[pageSizeOptions]="msaapPageSizeOptions"
[tableHeader]="msaapTableHeader"
[titleHeader]="msaapTitleHeader"
[artistHeader]="msaapArtistHeader"
[durationHeader]="msaapDurationHeader"
[displayArtist]="msaapDisplayArtist"
[displayDuration]="msaapDisplayDuration"
[expanded]="true"
(trackPlaying)="onTrackPlaying($event)"
(trackPaused)="onTrackPaused($event)"
(trackEnded)="onEnded($event)"
(nextTrackRequested)="onNextTrackRequested($event)"
(previousTrackRequested)="onPreviousTrackRequested($event)"
(trackSelected)="onTrackSelected($event)">
</ngx-audio-player>
TS
import { Track } from 'ngx-audio-player';
.
.
msaapDisplayPlayList = true;
msaapDisablePositionSlider = true;
msaapDisplayRepeatControls = true;
msaapDisplayVolumeControls = true;
msaapDisplayVolumeSlider = false;
msaapDisplayTitle = true;
msaapPageSizeOptions = [2,4,6];
msaapDisplayArtist = false;
msaapDisplayDuration = false;
msaapTableHeader = 'My Playlist';
msaapTitleHeader = 'My Title';
msaapArtistHeader = 'My Artist';
msaapDurationHeader = 'My Duration';
msaapPlaylist: Track[] = [
{
title: 'Audio One Title',
link: 'Link to Audio One URL',
artist: 'Artist',
duration: 'Duration'
},
{
title: 'Audio Two Title',
link: 'Link to Audio Two URL',
artist: 'Artist',
duration: 'Duration'
},
{
title: 'Audio Three Title',
link: 'Link to Audio Three URL',
artist: 'Artist',
duration: 'Duration'
},
];
onTrackPlaying(event) {
console.log(event);
}
onTrackPaused(event) {
console.log(event);
}
onEnded(event) {
console.log(event);
}
onNextTrackRequested(event) {
console.log(event);
}
onPreviousTrackRequested(event) {
console.log(event);
}
onTrackSelected(event) {
console.log(event);
}
Properties
Name | Description | Type |
---|
@Input() playlist: Track[]; | playlist containing array of title and link | mandatory |
@Input() autoPlay: false; | true - if the audio needs to be played automaticlly | optional |
Player Controls | | |
@Input() startOffset = 0; | offset from start of audio file in seconds | optional |
@Input() endOffset = 0; | offset from end of audio file in seconds | optional |
@Input() disablePositionSlider = false; | true - if the position slider needs to be disabled | optional |
@Input() displayRepeatControls = true; | false - if the repeat controls needs to be hidden | optional |
@Input() repeat: "all" | "one" | "none" = 'all'; |
@Input() displayVolumeControls = true; | false - if the volume controls needs to be hidden | optional |
@Input() displayVolumeSlider = true; | true - if the volume slider should be shown | optional |
Title Marquee Control | | |
@Input() displayTitle: true; | false - if the audio title needs to be hidden | optional |
Playlist Controls | | |
@Input() displayPlaylist: true; | false - if the playlist needs to be hidden | optional |
@Input() pageSizeOptions = [10, 20, 30]; | number of items to be displayed in the playlist | optional |
@Input() expanded = true; | false - if the playlist needs to be minimized | optional |
@Input() displayArtist = false; | true - if the artist data is to be shown | optional |
@Input() displayDuration = false; | true - if the track duration is to be shown | optional |
Localisation Controls | | |
@Input() tableHeader = 'Playlist'; | localised string | optional |
@Input() titleHeader = 'Title'; | localised string | optional |
@Input() artistHeader = 'Artist'; | localised string | optional |
@Input() durationHeader = 'Duration'; | localised string | optional |
Callback Events | | |
@Output() trackPlaying: EventEmitter | triggers when the track starts playing | optional |
@Output() trackPaused: EventEmitter | Callback method that triggers once the track ends | optional |
@Output() trackEnded: EventEmitter | Callback method that triggers once the track ends | optional |
@Output() nextTrackRequested: EventEmitter | Callback method that triggers once the track ends | optional |
@Output() previousTrackRequested: EventEmitter | Callback method that triggers once the track ends | optional |
@Output() trackSelected: EventEmitter | Callback method that triggers once the track ends | optional |
Versioning
ngx-audio-player will be maintained under the Semantic Versioning guidelines.
Releases will be numbered with the following format:
<major>.<minor>.<patch>
For more information on SemVer, please visit http://semver.org.
Contributors ✨
Thanks goes to these wonderful people:
Misc
License
The MIT License (MIT)
Donate
If you like my work you can buy me a :beer: or :pizza:
