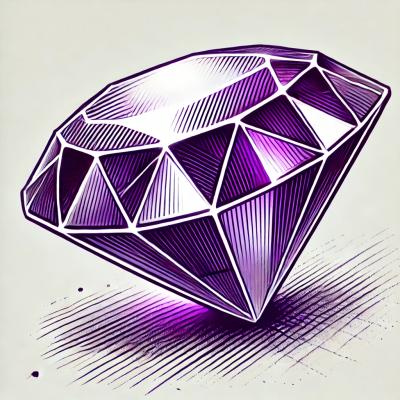
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
ngx-audio-player
Advanced tools
A library for loading and playing audio using HTML 5 for Angular 7/8/9/10. (https://vmudigal.github.io/ngx-audio-player/)
A library for loading and playing audio using HTML 5 for Angular 7/8/9/10.
(https://vmudigal.github.io/ngx-audio-player/)
A simple, clean, responsive player for playing single audio with or without title.
A simple, clean, responsive player for playing multiple audios with playlist support.
ngx-audio-player
is available via npm and yarn
Using npm:
$ npm install ngx-audio-player --save
Using yarn:
$ yarn add ngx-audio-player
NgxAudioPlayerModule needs Angular Material.
Make sure you have installed below dependencies with same or higher version than mentioned.
"@angular/core": "^10.0.0"
"@angular/common": "^10.0.0"
"@angular/material": "^10.0.0"
"rxjs": "^6.5.5"
Import NgxAudioPlayerModule
in in the root module(AppModule
):
// Import library module
import { NgxAudioPlayerModule } from 'ngx-audio-player';
@NgModule({
imports: [
// ...
NgxAudioPlayerModule
]
})
export class AppModule { }
<mat-basic-audio-player [audioUrl]="msbapAudioUrl" [title]="msbapTitle" [autoPlay]="false" muted="muted" (trackEnded)="onEnded($event)"
[displayTitle]="msbapDisplayTitle" [displayVolumeControls]="msaapDisplayVolumeControls"
[disablePositionSlider]="msbapDisablePositionSlider"></mat-basic-audio-player>
// Material Style Basic Audio Player Audio URL (Mandatory)
msbapAudioUrl = 'Link to audio URL';
// Material Style Basic Audio Player (Optional Parameters)
msbapTitle = 'Audio Title';
msbapDisplayTitle = false;
msbapDisplayVolumeControls = true;
msbapDisablePositionSlider = true;
Name | Description | Type | Default Value |
---|---|---|---|
@Input() title: string; | title to be displayed | optional | none |
@Input() audioUrl: string; | url of the audio | mandatory | none |
@Input() autoPlay: false; | true - if the audio needs to be played automaticlly | optional | false |
@Input() displayTitle = false; | true - if the audio title needs to be displayed | optional | false |
@Output() trackEnded: Subject; | Callback method thats triggers once the track ends | optional | - N.A - |
@Input() displayVolumeControls = true; | false - if the volume controls needs to be hidden | optional | true |
@Input() startOffset = 0; | offset from start of audio file in seconds | optional | 0 |
@Input() endOffset = 0; | offset from end of audio file in seconds | optional | 0 |
@Input() disablePositionSlider = false; | true - if the position slider needs to be disabled | optional | false |
<mat-advanced-audio-player [playlist]="msaapPlaylist" [displayTitle]="msaapDisplayTitle" [autoPlay]="false"
muted="muted" [displayPlaylist]="msaapDisplayPlayList" [pageSizeOptions]="pageSizeOptions" (trackEnded)="onEnded($event)"
[displayVolumeControls]="msaapDisplayVolumeControls" [disablePositionSlider]="msaapDisablePositionSlider"
[expanded]="true"></mat-advanced-audio-player>
import { Track } from 'ngx-audio-player';
.
.
msaapDisplayTitle = true;
msaapDisplayPlayList = true;
msaapPageSizeOptions = [2,4,6];
msaapDisplayVolumeControls = true;
msaapDisablePositionSlider = true;
// Material Style Advance Audio Player Playlist
msaapPlaylist: Track[] = [
{
title: 'Audio One Title',
link: 'Link to Audio One URL'
},
{
title: 'Audio Two Title',
link: 'Link to Audio Two URL'
},
{
title: 'Audio Three Title',
link: 'Link to Audio Three URL'
},
];
Name | Description | Type | Default Value |
---|---|---|---|
@Input() playlist: Track[]; | playlist containing array of title and link | mandatory | None |
@Input() autoPlay: false; | true - if the audio needs to be played automaticlly | optional | false |
@Input() displayTitle: true; | false - if the audio title needs to be hidden | optional | true |
@Input() displayPlaylist: true; | false - if the playlist needs to be hidden | optional | true |
@Input() pageSizeOptions = [10, 20, 30]; | number of items to be displayed in the playlist | optional | [10,20,30] |
@Input() expanded = true; | false - if the playlist needs to be minimized | optional | true |
@Input() displayVolumeControls = true; | false - if the volume controls needs to be hidden | optional | true |
@Output() trackEnded: Subject | Callback method thats triggers once the track ends | optional | - N.A - |
@Input() startOffset = 0; | offset from start of audio file in seconds | optional | 0 |
@Input() endOffset = 0; | offset from end of audio file in seconds | optional | 0 |
@Input() disablePositionSlider = false; | true - if the position slider needs to be disabled | optional | false |
ngx-audio-player will be maintained under the Semantic Versioning guidelines. Releases will be numbered with the following format:
<major>.<minor>.<patch>
For more information on SemVer, please visit http://semver.org.
Thanks goes to these wonderful people:
Edric Chan 💻 | RokiFoki 💻 | ewwwgiddings 📖 | Caleb Crosby 💻 |
If you like my work you can buy me a :beer: or :pizza:
FAQs
A library for loading and playing audio using HTML 5 for Angular 7/8/9/10/11/12. (https://mudigal-technologies.github.io/ngx-audio-player/)
The npm package ngx-audio-player receives a total of 156 weekly downloads. As such, ngx-audio-player popularity was classified as not popular.
We found that ngx-audio-player demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.