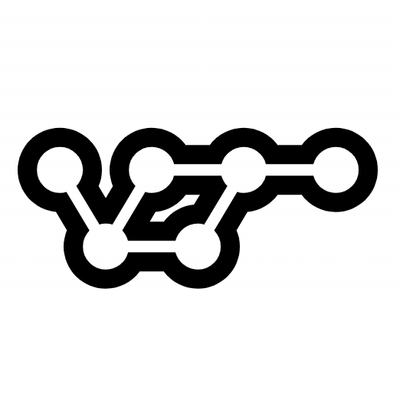
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
ngx-printer
Advanced tools
A simple service to print the window or parts of a window (div). Printing of Angular Templates or Components is possible.
A simple service to print the window or parts of a window (div). Printing of Angular Templates or Components is possible.
See DEMO App for examples.
The service prints by opening a new window. There is an option printWindowOpen to change this behavoir. Set printWindowOpen=false;
You can also set this option in .forRoot while importing the module to the app.module
imports: [
BrowserModule,
NgxPrinterModule.forRoot({printOpenWindow: true})
],
this.printerService.printCurrentWindow();
this.printerService.printDiv('idOfDivToPrint');
@ViewChild('PrintTemplate')
private PrintTemplateTpl: TemplateRef<any>;
printTemplate() {
this.printerService.printAngular(this.PrintTemplateTpl);
}
Beware: To print a component the component needs to be known by service (copy source and add it to entry component of app.module). Otherwise use printHTMLElement instead.
@ViewChild(LittleDummyComponent, {read: ElementRef}) PrintComponent: ElementRef;
printHTMLElementToCurrent() {
this.printerService.printHTMLElement(this.PrintComponent.nativeElement);
}
If you want to check whether the print window is open or not subscribe to obserbable $printWindowOpen
this.printWindowSunscription = this.printerService.$printWindowOpen.subscribe(val => {
console.log('Print window is open:', val);
});
There is an directive ngxPrintItem to mark and store an HTML-Element as an item which can be printed
later and anyhwere on the page.
An id has to be set.
These items are stored in the services observable printerService.$printItems.
Use the async pipe to subscribe and the function printPrintItem
to print the item.
HTML:
<span id="firstPrintItem" ngxPrintItem printName="First one" >A <b>first</b> span with an ngxPrintItem directive</span>
<div *ngFor="let prinItem of $printItems | async">
<span>{{prinItem.id}} - {{prinItem.printName}}</span>
<button (click)="printItem(prinItem)">Print me!</button>
</div>
TS:
printItem(itemToPrint: PrintItem) {
this.printerService.printPrintItem(itemToPrint);
}
});
FAQs
An easy to use service to print a window or parts of a window (div). Printing of Angular Templates or Components is possible.
The npm package ngx-printer receives a total of 1,760 weekly downloads. As such, ngx-printer popularity was classified as popular.
We found that ngx-printer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.