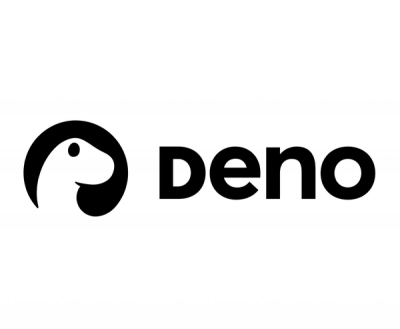
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
node-apex-api-security
Advanced tools
APEX API security utiity. Includes helper operations to generate HMAC-SHA256 and RSA-SHA256 signatures
A Javascript utility that generates HTTP security headers for authenticating with secured Apex endpoints, for Node.js.
$ npm install node-apex-api-security
ApiSigningUtil.getSignatureToken(options)
Returns a signature token used for authentication with a secured Apex API.
Authorization token with HMACSHA256 signature
const ApiSigningUtil = require('node-apex-api-security').ApiSigningUtil;
// Required options for L1 authentication
const requestOptions = {
appId: 'my-app-id', // Apex App ID
secret: 'my-app-secret', // Apex App secret used for L1 signature
authPrefix: 'apex_l1_eg', // Authentication prefix, determined by authentication level and gateway type
httpMethod: 'get', // HTTP method, e.g. GET/POST
urlPath: 'https://my.apex.api/endpoint' // URL to Apex API
};
// Apex_l1_ig realm="https://my.apex.api/endpoint",apex_l1_ig_app_id="my-app-id",apex_l1_ig_nonce="UldycUAF56GWJGlWz0YSwOOp5gruJqvBy0CJeZ4XpGk=",apex_l1_ig_signature="u5nTX4ZbkL8c9pp5C79VHu07QPPLG9yx2VxpLX7kqGM=",apex_l1_ig_signature_method="HMACSHA256",apex_l1_ig_timestamp="1523935422173",apex_l1_ig_version="1.0"
const L1SignatureToken = ApiSigningUtil.getSignatureToken(requestOptions);
Authorization token with SHA256withRSA signature
const ApiSigningUtil = require('node-apex-api-security').ApiSigningUtil;
// Required options for L2 authentication
const requestOptions = {
appId: 'my-app-id', // Apex App ID
keyString: '----BEGIN PRIVATE KEY-----...', // The PEM formatted private key's string
// keyFile: '/path/to/my/private.key', // Alternatively, simply pass in the path to private key used for L2 signature
authPrefix: 'apex_l2_eg', // Authentication prefix, determined by authentication level and gateway type
httpMethod: 'get', // HTTP method, e.g. GET/POST
urlPath: 'https://my.apex.api/endpoint' // URL to Apex API
};
// Apex_l2_ig realm="https://my.apex.api/endpoint",apex_l2_ig_app_id="my-app-id",apex_l2_ig_nonce="UldycUAF56GWJGlWz0YSwOOp5gruJqvBy0CJeZ4XpGk=",apex_l2_ig_signature="u5nTX4ZbkL8c9pp5C79VHu07QPPLG9yx2VxpLX7kqGM=",apex_l2_ig_signature_method="SHA256withRSA",apex_l2_ig_timestamp="1523935422173",apex_l2_ig_version="1.0"
const L2SignatureToken = ApiSigningUtil.getSignatureToken(requestOptions);
The generated token should then be added to the Authorization
header when making HTTP API calls:
GET /endpoint HTTP/1.1
Host: my.apex.api
Authorization: Apex_l1_ig realm="https://my.apex.api/endpoint",apex_l1_ig_app_id="my-app-id",apex_l1_ig_nonce="UldycUAF56GWJGlWz0YSwOOp5gruJqvBy0CJeZ4XpGk=",apex_l1_ig_signature="u5nTX4ZbkL8c9pp5C79VHu07QPPLG9yx2VxpLX7kqGM=",apex_l1_ig_signature_method="HMACSHA256",apex_l1_ig_timestamp="1523935422173",apex_l1_ig_version="1.0"
...
appId
Apex App ID. The App needs to be approved and activated by the API provider. This value can be obtained from the gateway portal.
let appId = 'my-app-id';
authPrefix
API gateway-specific authorization scheme for a specific gateway zone. Takes 1 of 4 possible values.
let authPrefix = 'Apex_l1_ig';
// or
let authPrefix = 'Apex_l1_eg';
// or
let authPrefix = 'Apex_l2_ig';
// or
let authPrefix = 'Apex_l2_eg';
httpMethod
The API HTTP method
let httpMethod = 'get';
urlPath
The full API endpoint, for example https://my-apex-api.api.gov.sg/api/my/specific/data.
Note: Must be the endpoint URL as served from the Apex gateway, from the domain api.gov.sg. This may differ from the actual HTTP endpoint that you are calling, for example if it were behind a proxy with a different URL.
IMPORTANT NOTE from v0.7.8 onwards : If you are intending pass in the query params in optional parameters queryString or formData, please remove the queryString from the urlPath. Checkout the optional section
let urlPath = "https://my.apex.api/v1/resources?host=https%3A%2F%2Fnd-hello.api.example.comß&panelName=hello";
secret
- Required for L1 signatureIf the API you are accessing is secured with an L1 policy, you need to provide the generated App secret that corresponds to the appId
provided.
Note: leave secret
undefined if you are using ApiSigningUtil L2 RSA256 Signing
let secret = 's0m3S3ecreT';
keyString
or keyFile
- Required for L2 signaturepassphrase
If the API you are access is secured with an L2 policy, you need to provide the private key corresponding to the public key uploaded for appId
.
Provide either the path to your private key used to generate your L2 signature in keyFile
or the actual contents in keyString
.
let keyFile = '/path/to/my/private.key';
// or
let keyString = '----BEGIN PRIVATE KEY ----\n ${private_key_contents} \n -----END PRIVATE KEY-----';
let passphrase = 'passphrase for the keyString';
realm
An identifier for the caller, this can be set to any value.
formData
Object representation of form data (x-www-form-urlencoded) passed during HTTP POST / HTTP PUT requests
//For Signature generation (do not need to be URL encoded)
let formData = {key : 'value'};
//For making the actual HTTP POST call (need to be URL encoded)
let postData = qs.stringify(formData, null, null, {encodeURIComponent: encodeURIComponent});
let req = request(param.httpMethod, targetURL.href);
req.buffer(true);
req = req.type("application/x-www-form-urlencoded").set("Content-Length", Buffer.byteLength(postData)).send(postData);
NOTE
For formData parameter used for Signature generation, the key value parameters do not need to be URL encoded, When your client program is making the actual HTTP POST call, the key value parameters has to be URL encoded
queryString
Object representation of URL query parameters, for the API.
IMPORTANT NOTE For version v0.7.7 and below : You can also leave the query string on the urlPath parameter; it will automatically be extracted, and you won't have to use this parameter.
IMPORTANT NOTE From v0.7.8 onwards : If you pass in the params in queryString or formData, please remove the queryString from the urlPath parameter
For example, the API endpoint is https://example.com/v1/api?key=value , then you have you pass in the params in this manner below :
// For example, if the endpoint contains a query string: https://api.example.com?abc=def&ghi=123
let qsData = {
abc: 'def',
ghi: 123
}
//Prepare request options for signature formation
const requestOptions = {
appId: 'my-app-id', // Apex App ID
secret: 'my-app-secret', // Apex App secret used for L1 signature
authPrefix: 'apex_l1_eg', // Authentication prefix, determined by authentication level and gateway type
httpMethod: 'get', // HTTP method, e.g. GET/POST
urlPath: 'https://api.example.com' // URL that remove away the queryString
queryString : qsData
};
nonce
An arbitrary string, needs to be different after each successful API call. Defaults to 32 bytes random value encoded in base64.
timestamp
A unix timestamp. Defaults to the current unix timestamp.
Logging
To see detailed logs while using ApiSigningUtil, set the log level to trace
ApiSigningUtil.setLogLevel('trace');
Authorization: Apex_l2_ig realm="http://api.mygateway.com",
apex_l2_ig_timestamp="1502199514462",
apex_l2_ig_nonce="UldycUAF56GWJGlWz0YSwOOp5gruJqvBy0CJeZ4XpGk=",
apex_l2_ig_app_id="my-apex-app-id",
apex_l2_ig_signature_method="SHA256withRSA",
apex_l2_ig_signature="Gigxd7Yif2NqiFGI3oi0D3+sVv3QxURLPwCSE9ARyeenYhipG+6gncCR+tWEfaQBGyH9gnG6RtwZh3A==",
apex_l2_ig_version="1.0"
For more information about contributing, and raising PRs or issues, see CONTRIBUTING.md.
See CHANGELOG.md.
Licensed under the MIT LICENSE
FAQs
APEX API security utiity. Includes helper operations to generate HMAC-SHA256 and RSA-SHA256 signatures
The npm package node-apex-api-security receives a total of 97 weekly downloads. As such, node-apex-api-security popularity was classified as not popular.
We found that node-apex-api-security demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.