Node Guard
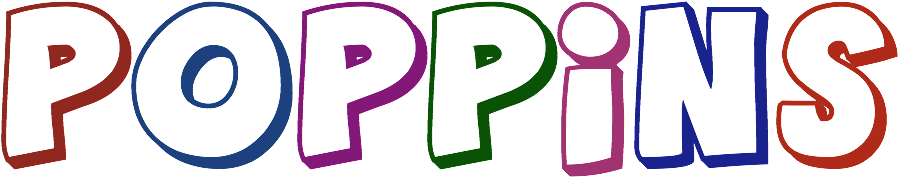

General purpose I/O module to add following http headers to keep your webpages securing them from malware attacks. This module can be used with any node http server.
X-XSS-Protection
X-XSS-Protection
http header saves you from XSS
attacks. node-guard
will set up X-XSS-Protection
header on all modern browsers and will disable it for older versions of IE as they have security vulnerabilities defined here https://technet.microsoft.com/library/security/ms10-002.
const http = require('http')
const guard = require('node-guard')
const options = {
enabled: true,
enableOnOldIE: false
}
http.createServer(function (req, res) {
guard.addXssFilter(req, res, options)
res.end()
}).listen(3000)
X-Frame-Options
X-Frame-Options
defines whether your webpage can be embedded as an iframe to other website. It can take 3 different values from ALLOW-FROM
, DENY
and SAMEORIGIN
const http = require('http')
const guard = require('node-guard')
const options = 'DENY'
const options = 'SAMEORIGIN'
const options = 'ALLOW-FROM http://example.com'
http.createServer(function (req, res) {
guard.addFrameOptions(res, options)
res.end()
}).listen(3000)
X-Content-Type-Options
X-Content-Type-Options
disables sniffing from web browsers, where they will try to sniff
mimetypes. Which means a web browser will execute the javascript file even if the content-type
of that file is not set to javascript
. Give it a read https://miki.it/blog/2014/7/8/abusing-jsonp-with-rosetta-flash/
const http = require('http')
const guard = require('node-guard')
const options = true
http.createServer(function (req, res) {
guard.addNoSniff(res, options)
res.end()
}).listen(3000)
X-Download-Options
IE specific header to stop users from executing html files with the access to your site context. Here is a good read on same https://blogs.msdn.microsoft.com/ie/2008/07/02/ie8-security-part-v-comprehensive-protection/.
const http = require('http')
const guard = require('node-guard')
const options = true
http.createServer(function (req, res) {
guard.addNoOpen(res, options)
res.end()
}).listen(3000)