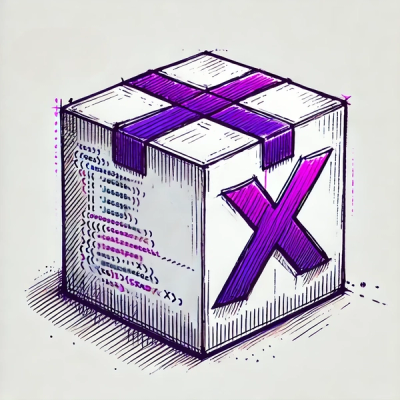
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
node-kraken-api
Advanced tools
a typed REST/WS Node.JS client for the Kraken cryptocurrency exchange
node-kraken-api is a typed REST/WS Node.JS client for the Kraken cryptocurrency exchange.
This is an unofficial API. Please refer to the official documentation for up-to-date information.
REST API Docs: kraken.com/features/api
WebSocket API Docs: docs.kraken.com/websockets
RetrieveExport
(binary endpoint); see the example.CHANGELOG | Synopsis | Usage | Code |
---|
The entire project has been completely rewritten using TypeScript and many features have changed.
If you're upgrading, please review the changes and upgrade guide below.
Settings.parse
, Settings.dataFormatter
)
.call()
function.Settings.pubMethods
, Settings.privMethods
)
.call()
function..call()
.request()
and the named REST methods.Settings.limiter
and Settings.tier
)
Settings.retryCt
)
.timeout
setting.Settings.syncIntervals
)
Settings.hostname
, Settings.version
)
Settings.otp
and .setOTP()
)
Settings.genotp
module.exports()
.call()
with the corresponding named method or .request()
.
.setOTP()
and Settings.otp
; provide .genotp
in the settings.retryCt
times.const kraken = require('node-kraken-api')({...})
), you will need to use the module.exports.Kraken
class instead: import { Kraken } from "node-kraken-api"; const kraken = new Kraken({...});
Minor changes to the Emitter class.
(...args: <type>[]) => boolean
-> (args: [<type>, <type>, ...]) => args is [<subtype>, <subtype>, ...]
.request()
.time()
.systemStatus()
.assets()
.assetPairs()
.ticker()
.ohlc()
.depth()
.trades()
.spread()
.getWebSocketsToken()
.balance()
.tradeBalance()
.openOrders()
.closedOrders()
.queryOrders()
.tradesHistory()
.queryTrades()
.openPositions()
.ledgers()
.queryLedgers()
.tradeVolume()
.addExport()
.exportStatus()
.retrieveExport()
.removeExport()
.addOrder()
.cancelOrder()
.cancelAll()
.cancelAllOrdersAfter()
.depositMethods()
.depositAddresses()
.depositStatus()
.withdrawInfo()
.withdrawStatus()
.withdrawCancel()
.walletTransfer()
.stake()
.unstake()
.stakingAssets()
.stakingPending()
.stakingTransactions()
.ws.ticker()
.ws.ohlc()
.ws.trade()
.ws.spread()
.ws.book()
.ws.ownTrades()
.ws.openOrders()
.ws.addOrder()
.ws.cancelOrder()
.ws.cancelAll()
.ws.cancelAllOrdersAfter()
npm i --save node-kraken-api
import { Kraken } from "node-kraken-api";
new Kraken({
/** REST API key. */
key?: string;
/** REST API secret. */
secret?: string;
/** REST API OTP generator. */
genotp?: () => string;
/**
* Nonce generator (the default is ms time with an incrementation guarantee).
* Note: Some other APIs use a spoofed microsecond time. If you are using an
* API key used by one of those APIs then you will need to use a custom
* nonce generator (e.g. () => Date.now() * 1000). See _GENNONCE for the
* default generation logic.
*/
gennonce?: () => number;
/** Connection timeout (default 1000). */
timeout?: number;
});
const kraken = new Kraken();
const { unixtime } = await kraken.time();
const { XXBT } = await kraken.assets();
const ticker = await kraken.ticker({ pair: "XXBTZUSD" })
const kraken = new Kraken({ key: "...", secret: "..." });
const { txid } = await kraken.addOrder({
pair: "XXBTZUSD",
type: "buy",
ordertype: "limit",
price: "65432.1",
volume: "1",
});
If your key requires an OTP, provide a generator:
const kraken = new Kraken({ key: "...", secret: "...", genotp: () => "..." });
RetrieveExport is the only method that promises a buffer:
const kraken = new Kraken({ key: "...", secret: "..." });
const buf = await kraken.retrieveExport({ id: "FOOB" })
fs.writeFileSync("report.zip", buf)
.ws
.
.ws.pub
and .ws.priv
provides ping, heartbeat, systemStatus, and general error monitoring.Kraken.WS.Connection.open()
and Kraken.WS.Connection.close()
for manual connection management.Kraken.Subscriber
object that manages subscriptions for a given name and options.const kraken = new Kraken();
const trade = await kraken.ws.trade()
.on('update', (update, pair) => console.log(update, pair))
.on('status', (status) => console.log(status))
.on('error', (error, pair) => console.log(error, pair))
// .subscribe() never rejects! rely on the 'error' and 'status' events
.subscribe('XBT/USD')
const book100 = await kraken.ws.book({depth: 100})
// live book construction from "snapshot", "ask", and "bid" events.
.on("mirror", (mirror, pair) => console.log(mirror, pair))
.on("error", (error, pair) => console.log(error, pair))
// resubscribes if there is a checksum validation issue (emits statuses).
.on("status", (status) => console.log(status)
.subscribe("XBT/USD", "ETH/USD"); // subscribe to multiple pairs at once
// unsubscribe from one or more subscriptions
// .unsubscribe() never rejects! rely on the 'error' and 'status' events
await book100.unsubscribe('XBT/ETH');
const kraken = new Kraken({ key: "...", secret: "..." });
const { token } = await kraken.getWebSocketsToken();
const orders = kraken.ws.openOrders({ token: token! })
.on("update", (update, sequence) => console.log(update, sequence))
.subscribe();
await orders.unsubscribe();
// The token does not expire while the subscription is active, but if you wish
// to resubscribe after unsubscribing you may need to call .ws.openOrders() again.
Testing is performed by @jpcx/testts.
To run tests:
auth.json
file with your key and secret: { key: string, secret: string }
npm test
in the main directory.Contribution is welcome! Given the amount of typings in this project, there may be discrepancies so please raise an issue or create a pull request.
Also, I am US-based and can't access the futures API; if you have access and want to contribute let me know!
Justin Collier - jpcx
msg: node-kraken-api
btc: bc1q3asl6wjnmarx4r9qcc04gkcld9q2qaqk42dvh6
sig: J4p7GsyX/2wQLk32Zfi/AmubUzGM66I6ah+mEn8Vpqf4EpfPuWYGaLcu2J8tdcsRGMAsmavbz/SJnw7yr3c0Duw=
Inspired by npm-kraken-api (nothingisdead).
This project is licensed under the MIT License - see the LICENSE file for details
2.2.2 (2022-06-10)
| Changes since 2.2.1 | Release Notes | README | | --- | --- | --- |
| Source Code (zip) | Source Code (tar.gz) | | --- | --- |
Fixed
Changed
<a name="2.2.1"></a>
FAQs
a typed REST/WS Node.JS client for the Kraken cryptocurrency exchange
The npm package node-kraken-api receives a total of 1,490 weekly downloads. As such, node-kraken-api popularity was classified as popular.
We found that node-kraken-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.